Testing in Node.js with Mocha and Chai
Conclusions and Next Steps
Throughout this course, we have thoroughly explored the various techniques, tools, and best practices for testing Node.js applications using Mocha and Chai. We have covered everything from basic testing concepts to practical examples and advanced use cases. In this final chapter, we will summarize what we have learned and discuss the next steps you can take to continue improving your testing skills.
Course Summary
Introduction to Testing in Node.js
We understood the importance of testing in software development and how Mocha and Chai integrate into the Node.js ecosystem to provide a powerful and flexible testing environment.
Installing and Setting Up Mocha and Chai
We learned how to install and set up Mocha and Chai, establishing a basic yet functional testing environment.
Basic Testing Concepts
We explored different types of tests, such as unit tests, integration tests, and functional tests, and understood how to structure and execute them effectively.
Structure of a Test with Mocha
We saw the basic structure of a test using Mocha, including the use of describe
, it
, before
, beforeEach
, after
, and afterEach
.
Asserts and Matchers with Chai
We learned how to use the different assertion styles provided by Chai (assert
, expect
, and should
) to perform precise and detailed verifications in our tests.
Test Driven Development (TDD) with Mocha and Chai
We explored the Test Driven Development (TDD) methodology and how to implement it using Mocha and Chai to write high-quality, well-tested code.
Mocks and Stubs with Sinon.js
We used Sinon.js to create mocks and stubs that allow us to isolate units of code under test and perform detailed verifications of interactions and states.
Testing Asynchronous Functions
We learned how to test asynchronous functions using different techniques such as callbacks, promises, and async/await
to ensure our applications handle asynchronous operations correctly.
Testing RESTful APIs
We saw how to test RESTful APIs using Supertest, and how to verify both successful responses and errors to ensure the robustness of our APIs.
Integration of Tests in the CI/CD Process
We explored how to integrate our tests into a CI/CD pipeline to ensure that every code change is automatically tested before being deployed, using tools like Travis CI, GitHub Actions, and Jenkins.
Load and Performance Testing
We learned how to perform load and performance testing using tools like Artillery and k6 to ensure our applications can handle high traffic volumes and maintain expected performance.
Best Practices in Testing
We discussed best practices in testing to ensure our tests are efficient, maintainable, and effective. This includes automation, keeping tests simple and clear, and ensuring good code coverage.
Complementary Tools for Testing
We explored several complementary tools that can enhance our testing process, including Istanbul for code coverage, Cypress for E2E testing, and ESLint to ensure code quality.
Practical Examples and Use Cases
We saw practical examples and use cases that integrate all the techniques and tools discussed in the course, providing an applicable understanding of how to structure and execute tests in Node.js projects.
Next Steps
Deepen Your Knowledge in Specific Tools
Although we have covered various tools in this course, there is always more to learn. Dive into the official documentation of Mocha, Chai, Sinon.js, and other mentioned tools to explore advanced features.
Contribute to Open Source Projects
An excellent way to improve your skills is to contribute to open source projects. Many popular libraries and frameworks accept contributions to improve their test coverage.
Learn About DevOps
Testing is just one part of the software development lifecycle. Learning about DevOps practices will help you understand how tests integrate into the continuous deployment cycle and how you can improve overall development and operation processes.
Take Advanced Courses and Certifications
Consider taking advanced courses or certifications in software testing, TDD, or CI/CD tools. This will not only strengthen your skills but also enhance your professional prospects.
Stay Updated
The software development ecosystem changes rapidly. Follow blogs, attend conferences, and stay active in developer communities to keep up with the latest trends and best practices in testing.
Conclusion
Testing is an essential skill for any software developer. Throughout this course, you have gained a comprehensive understanding of how to perform effective tests on Node.js applications using a variety of tools and techniques. By following the recommended next steps and continuing your learning, you will be well-prepared to create robust and high-quality applications. Good luck on your continuous journey in the world of testing!
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
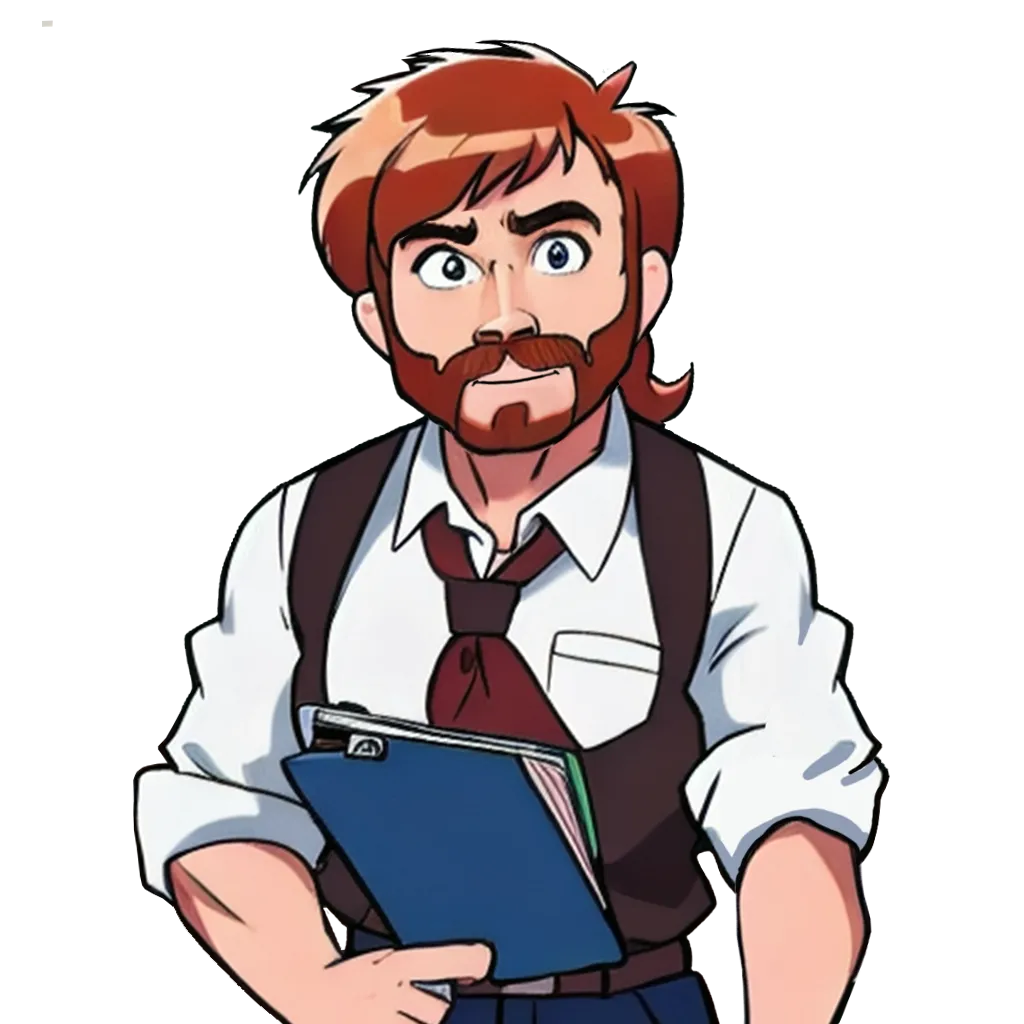