Testing in Node.js with Mocha and Chai
Mocks and Stubs with Sinon.js
In software development, it is common to encounter functions or methods that interact with external services, databases, or other modules. To test these components in isolation, we can use mocks and stubs. Sinon.js is a library that facilitates the creation of mocks, stubs, and spies, and integrates seamlessly with Mocha and Chai. In this chapter, we will explain how to use Sinon.js to improve our tests.
Key Concepts
Mocks
A mock is an object that takes the place of a real object in the code and is configured to expect certain calls and behaviors. A mock can verify if a method or function has been called in the expected way.
Stubs
A stub is a function that temporarily replaces a real function and allows you to specify its behavior, such as returning a predefined value or throwing an error.
Spies
A spy is a function that records information about the interactions with other functions, such as how many times it was called or with what arguments.
Installing Sinon.js
You can install Sinon.js in your project with the following command:
shell
Using Stubs with Sinon.js
Let's say we have a function that makes a call to a database. To test this function without accessing the database, we can use a stub.
Example:
Suppose you have a function obtenerUsuarioDeDB
that interacts with the database:
javascript
We want to test a function that uses obtenerUsuarioDeDB
, called procesarUsuario
:
javascript
Create a stub for obtenerUsuarioDeDB
in a test:
javascript
Using Mocks with Sinon.js
Mocks are useful for verifying that a function is called with certain parameters. Suppose we have a function guardarUsuario
that saves user data to the database.
Example:
javascript
Now we want to test another function manejarUsuario
that calls guardarUsuario
:
javascript
Create a mock for guardarUsuario
in a test:
javascript
Using Spies with Sinon.js
Spies are useful for knowing how and when a function is called. They help to record the function interaction without changing its behavior.
Example:
javascript
Conclusion
Mocks, stubs, and spies are powerful tools for conducting more isolated and controlled tests, allowing you to simulate and verify specific code behaviors. Sinon.js provides robust functionality for working with these tools alongside Mocha and Chai, thereby improving the effectiveness and reliability of your tests. In the next chapter, we'll explore how to handle and test asynchronous functions in Node.js.
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
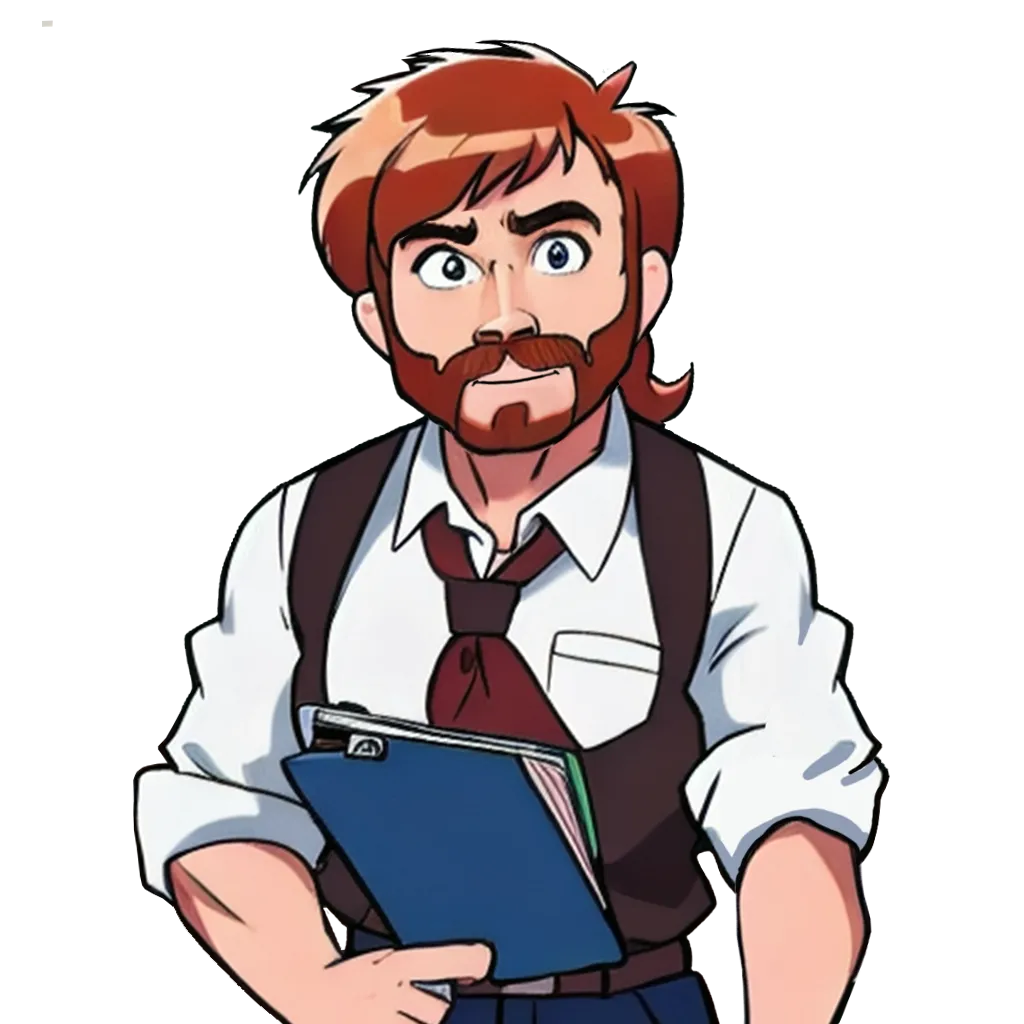