Testing in Node.js with Mocha and Chai
Basic Testing Concepts
Before diving into writing tests with Mocha and Chai, it's crucial to understand some basic testing concepts that will help you write more effective tests and maintain software quality.
Types of Testing
Unit Tests
Unit tests focus on validating the behavior of individual software components, such as functions or methods. These tests ensure that each unit of code works correctly in isolation.
Example of a unit test:
javascript
Integration Tests
Integration tests verify that different modules or services of the system work correctly together. This type of testing is vital to ensure that dependencies and interactions between different parts of the system do not break the software's functionality.
Functional Tests
Functional tests evaluate the entire system to ensure it meets the functional requirements. They focus on the output generated by executing scripts or commands by the user.
Test Lifecycle
- Setup: Initializes the conditions necessary for the test.
- Exercise: Executes the functionality that is to be tested.
- Verify: Checks that the results of the execution are as expected.
- Teardown: Cleans up or resets the test environment.
Key Terminology
Test Suite
A test suite is a collection of related test cases. Mocha provides methods like describe
to group tests into suites.
Test Case
A test case is an individual test that validates a specific aspect of the system's behavior. In Mocha, we use the it
method to define test cases.
Assertions
Assertions are statements about the expected behavior of the code. Chai provides various assertion methods like expect
, should
, and assert
.
Best Practices
- Fast and Isolated Unit Tests: Unit tests should be quick to execute and not depend on external factors like databases or network services.
- Clear and Descriptive Names: Tests and test suites should have clear and descriptive names to make them easier to understand.
- One Test, One Behavior: Each test case should validate a specific behavior, avoiding overloaded tests that make it difficult to identify the cause of a failure.
- Run Tests Frequently: Integrate test execution into the daily workflow to detect issues as early as possible.
Complete Example of a Test Lifecycle
javascript
With these essential concepts clear, you're ready to start writing more structured and effective tests. In the upcoming chapters, we'll focus on the specific features of Mocha and Chai, and how to use them to implement these principles.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
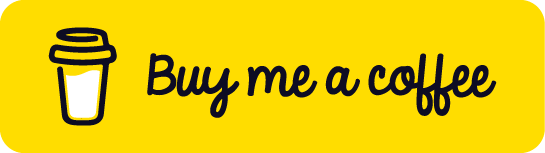
Chat with Chuck
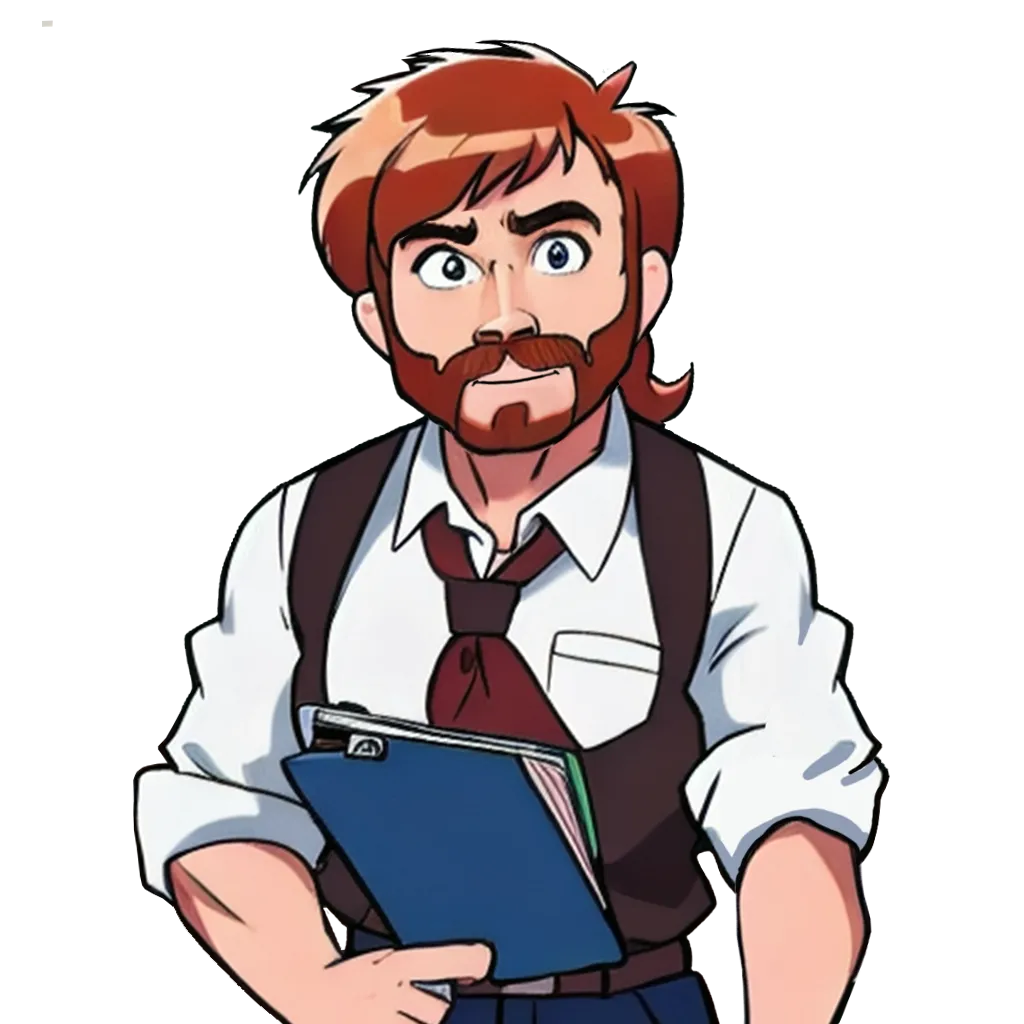
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps