Testing in Node.js with Mocha and Chai
Test Driven Development (TDD) with Mocha and Chai
Test Driven Development (TDD) is a software development methodology that focuses on writing tests before writing functional code. This technique not only improves code quality but also helps define requirements clearly before implementation. In this chapter, we will explore how to implement TDD using Mocha and Chai.
Principles of TDD
- Write a Test that Fails: Write a minimal test that fails because the functionality is not yet implemented.
- Write the Minimum Code to Pass the Test: Implement the necessary functionality for the test to pass.
- Refactor the Code: Improve the code ensuring all tests still pass without adding new functionalities.
- Repeat: Repeat the cycle for each new functionality.
Practical Example
Let's follow a step-by-step example to implement TDD with Mocha and Chai.
Step 1: Write a Failing Test
Suppose we want to implement a function esNumeroPar
that determines if a number is even. We start by writing a test to check this functionality.
Create a file named test/esNumeroPar.test.js
and write the following code:
javascript
Try running npm test
. As expected, the tests will fail because the esNumeroPar
function does not exist yet.
Step 2: Write the Minimum Code to Pass the Test
Next, we implement the esNumeroPar
function so that the tests pass. Create a file src/esNumeroPar.js
and write the minimum code necessary:
javascript
Update the test file test/esNumeroPar.test.js
to import the correct function:
javascript
Run npm test
again. This time, the tests should pass.
Step 3: Refactor the Code
In this case, the esNumeroPar
function is already quite simple, so there's not much to refactor. However, it's important to review the code to see if there are opportunities for simplification or improvement without breaking the tests.
Benefits of TDD
- Higher Quality Code: Writing tests before implementing functionality forces developers to think about requirements and possible edge cases before writing code.
- Fewer Defects: By ensuring that each piece of functionality has adequate test coverage, errors are detected and fixed more quickly.
- Living Documentation: Tests act as live documentation, demonstrating how the code is expected to behave in different situations.
- Easier Refactoring: With a solid test suite, you can refactor code with confidence, knowing that the tests will verify nothing breaks.
Conclusion
Test Driven Development (TDD) is an efficient methodology that can lead to cleaner code, fewer errors, and a better understanding of requirements. Mocha and Chai are excellent tools for applying TDD in Node.js applications, providing a clear and powerful structure for your tests. In the next chapter, we will explore Mocks and Stubs with Sinon.js and how they integrate into our tests.
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
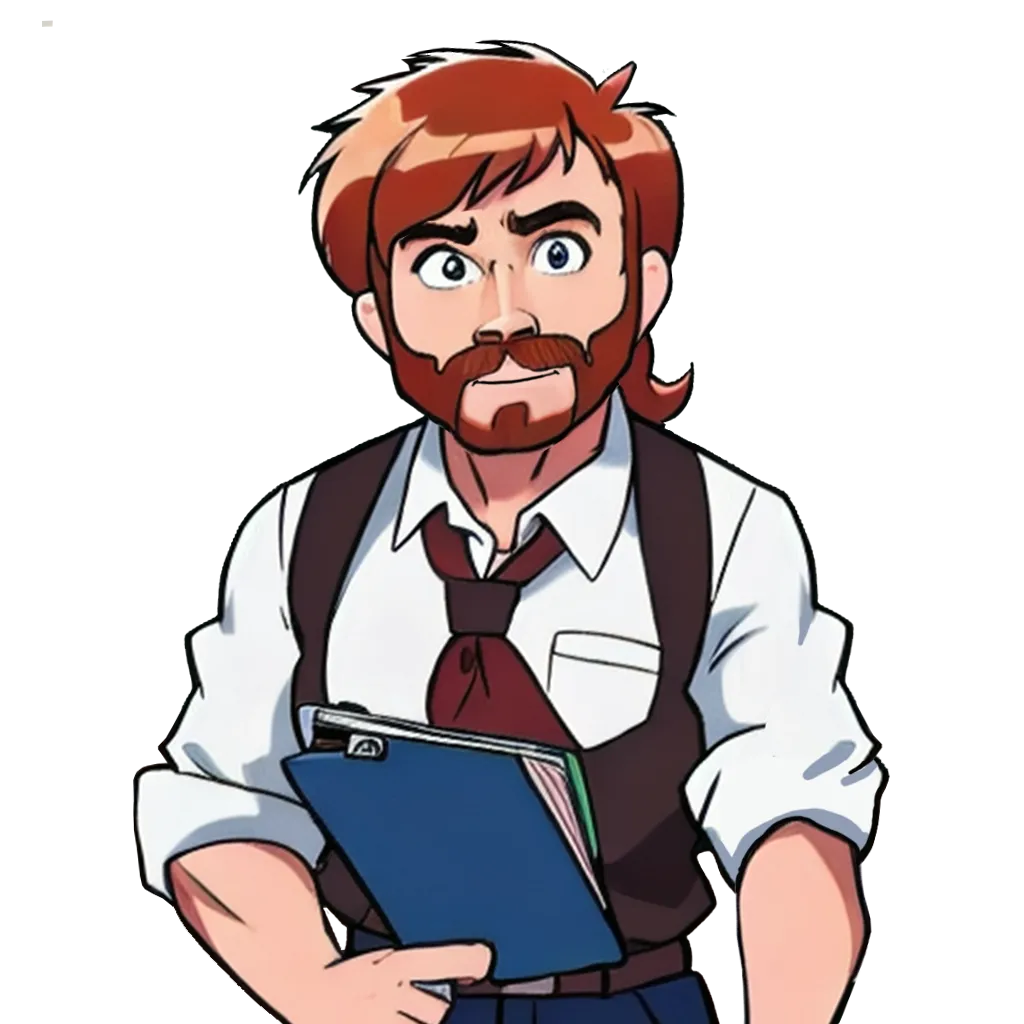