Testing in Node.js with Mocha and Chai
Load and Performance Testing
Load and performance testing are crucial to ensure that your application can handle high volumes of traffic and meet expected response times. These tests help identify bottlenecks and scalability issues before they affect users. In this chapter, we will explore how to perform load and performance testing on Node.js applications using tools like Artillery and k6.
What Are Load and Performance Testing?
- Load Testing: Evaluates the performance of an application under normal and heavy usage conditions. It helps determine the application's behavior when accessed by multiple users simultaneously.
- Stress Testing: Evaluates performance under extreme conditions to identify the application's breaking point.
- Capacity Testing: Determines the maximum number of users or requests an application can handle before becoming unstable.
Installing Tools
Artillery
Artillery is a popular and easy-to-use tool for load and performance testing in Node.js applications.
Install it globally with npm:
shell
k6
k6 is another powerful tool for load testing, especially known for its simplicity and CI/CD integration.
Download and install k6 from its official page.
Configuring Artillery
Create a basic configuration file for Artillery, for example artillery-config.yml
:
yaml
This file configures a test that will send 10 requests per second to http://localhost:3000/usuario/1
for 60 seconds.
Running the Test
To run the test, use the following command:
shell
This will generate a detailed report that includes metrics such as response times, success rates, and errors.
Configuring k6
Here is how to configure and run a load test with k6. Create a file k6-test.js
:
javascript
This script configures a test with three stages: ramping up traffic to 10 users, staying at 10 users for one minute, and then ramping down to 0 users.
Running the Test
To run the test, use the following command:
shell
This will generate a detailed report similar to Artillery.
Analyzing Results
Load testing tools like Artillery and k6 will generate detailed reports that include key metrics such as:
- Response Times: Average, minimum, and maximum time taken by the application to respond to a request.
- Performance under Load: How the application behaves as the number of users or requests increases.
- Errors: Number and types of errors that occurred during the test.
- Capacity: Breaking point at which the application can no longer handle additional load.
Best Practices
- Realistic Tests: Configure your tests to simulate realistic traffic scenarios. Don't just test extreme conditions.
- Real-time Monitoring: Use monitoring tools to complement load tests and get a real-time view of your application's performance.
- Iteration and Improvement: Use test results to identify and fix bottlenecks. Repeat tests after making improvements.
Integration into CI/CD
Both Artillery and k6 can be easily integrated into CI/CD pipelines, allowing load tests to run automatically with each code change.
Example Integration with GitHub Actions:
yaml
Conclusion
Load and performance testing are essential to ensure your application can handle real traffic and meet user expectations. Tools like Artillery and k6 provide a simple and effective way to conduct these tests. Integrating them into your CI/CD pipeline ensures that any code changes do not negatively affect your application's performance. In the next chapter, we will explore best practices in testing to ensure the effectiveness and efficiency of your tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
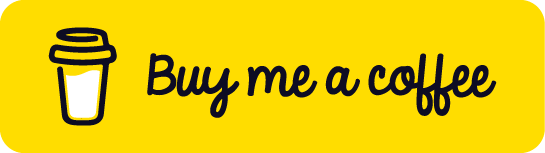
Chat with Chuck
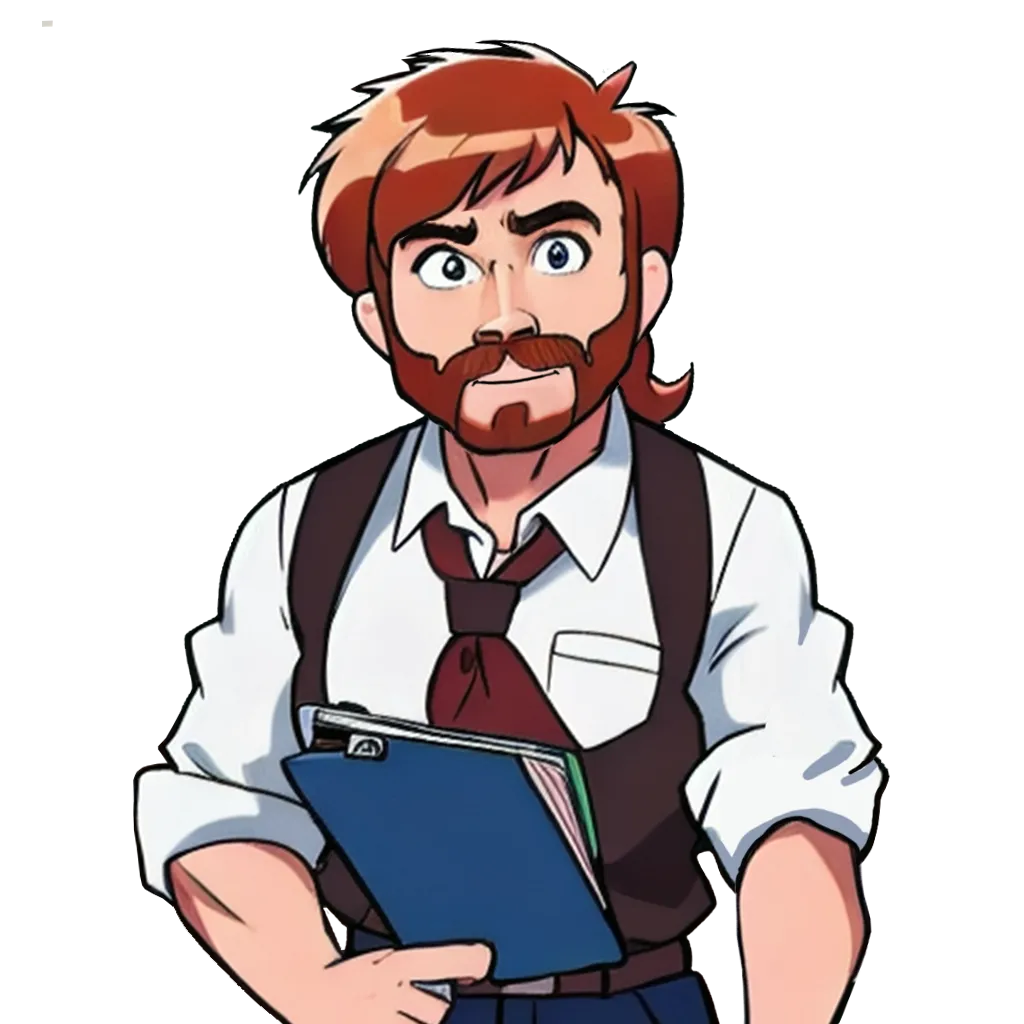
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps