Testing JavaScript and DOM with Jest
Accessibility Testing with Jest
Accessibility Testing with Jest
Accessibility is a crucial aspect of web application development. We ensure that our applications are accessible to everyone, including users with disabilities. In this section, we will learn how to conduct accessibility tests using jest-axe
, a powerful tool that facilitates the incorporation of accessibility rules in our tests.
What is jest-axe
?
jest-axe
is a library that, in combination with Jest, allows running accessibility rules (WCAG, ARIA) on web content to identify accessibility issues.
Installing jest-axe
First, install jest-axe
in your project:
bash
Basic Configuration
Set up jest-axe
for use with Jest by writing a setup file:
javascript
Make sure to configure this file in your Jest setup (jest.config.js
or in package.json
):
json
Writing Accessibility Tests
We will perform an accessibility test on a simple component to ensure it meets accessibility standards. Suppose we have the following component:
html
Let's proceed to write the accessibility tests:
javascript
Improving Accessibility
To improve accessibility, let's add some essential attributes and elements:
html
We modify the accessibility test to verify these changes:
javascript
Additional Accessibility Tests
Accessibility tests are not limited to simple forms but should also include more complex components and dynamic elements. Here are some additional examples:
- Accessible Modals:
html
- Accessible Modal Test:
javascript
- Dynamic Elements:
To test dynamic components like dropdown menus or sliders:
javascript
Placeholder for image: [An illustration showing how jest-axe
performs an automated DOM analysis and detects accessibility violations]
With these tools and practices, you can start integrating accessibility tests into your development workflow. Ensuring your application is accessible not only improves the user experience but also complies with legal and ethical standards. In the next section, we will explore asynchronous testing with Jest, essential for modern applications that rely on network communications and asynchronous operations.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
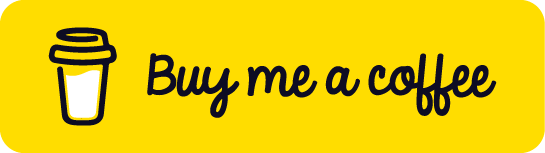
Chat with Chuck
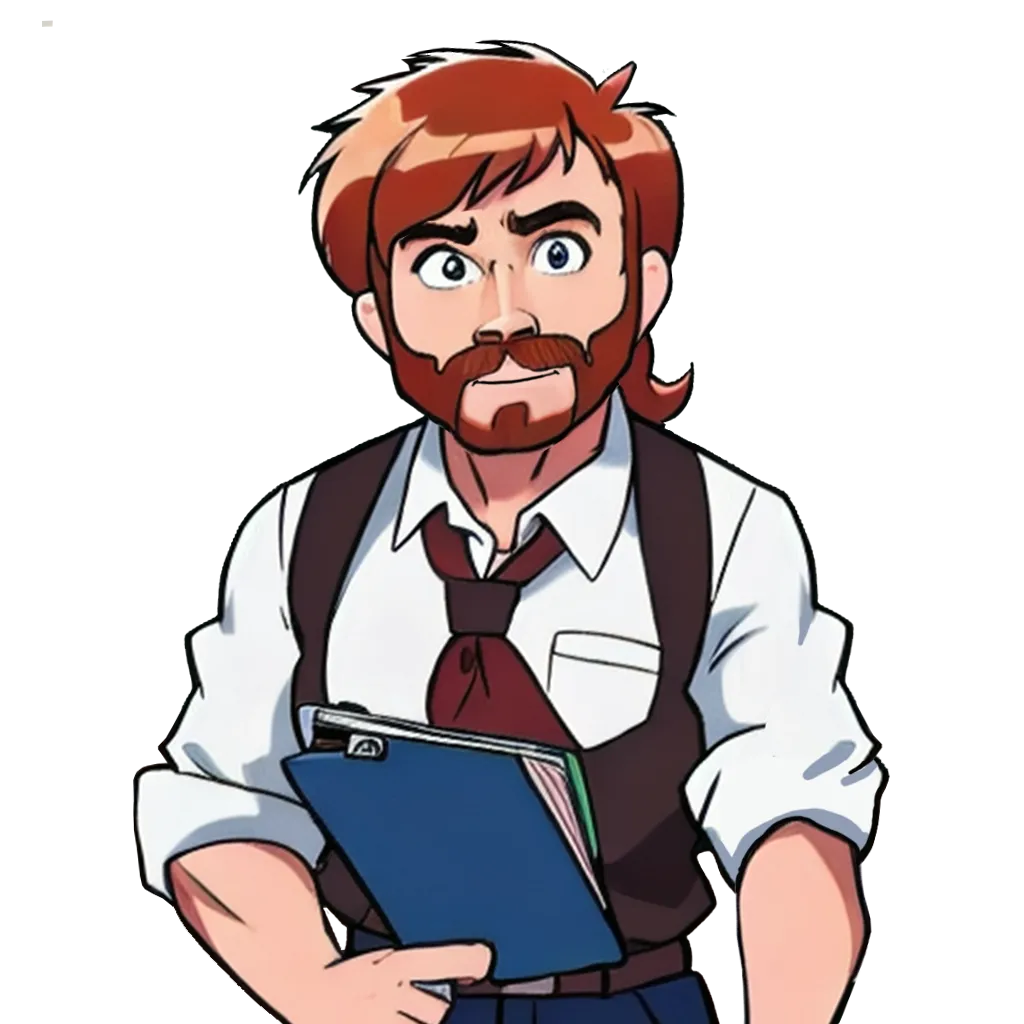
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest