Testing JavaScript and DOM with Jest
Best Practices for Testing with Jest
Best Practices for Testing with Jest
Best practices in testing help write test cases that are more maintainable, efficient, and useful in the long term. In this section, we will explore some of the best practices for writing tests with Jest.
1. Write Isolated Tests
Ensure that each test is independent of the others. This improves reliability and makes diagnosing errors easier.
javascript
2. Use Clear Descriptions
Use clear and meaningful descriptions for your tests and groupings (describe
). This makes it easier to read and understand the test results.
javascript
3. Setup and Cleanup
Use beforeEach
, afterEach
, beforeAll
, and afterAll
to setup and cleanup before and after each test. This reduces code duplication and ensures that each test starts from the same initial state.
javascript
4. Avoid Fragile Tests
A fragile test is one that fails for minor reasons or changes frequently. Make sure your tests are robust and do not rely on the internal implementation of the code they are testing.
javascript
5. Use Appropriate Matchers
Jest provides a range of matchers that help make your tests more expressive and precise. Use the appropriate matcher for the situation.
javascript
6. Use Mocks and Spies
Use mocks and spies to spy on function calls or simulate specific behaviors. This is particularly useful for integration and unit testing.
javascript
7. Keep Test Suites and Files Organized
Keep your test files organized by using a consistent naming scheme and location.
bash
8. Generate Coverage Reports
Use the coverage tool to understand which parts of your code are being tested.
json
9. Do Test Driven Development (TDD)
Writing tests before the code helps you think about the requirements and possible failures. Experiment with TDD to improve the quality of your code.
10. Review and Refactor Tests
Your tests should be maintained with the same care as production code. Regularly review and refactor your tests to keep them clean and efficient.
Complete Example Applying Best Practices
javascript
Placeholder for image: [Illustration showing a well-structured and organized test suite, highlighting the different best practices]
Applying these best practices will help you write more effective and maintainable tests, improving the quality of your code. In the next section, we will learn how to debug failing tests in Jest, a crucial skill for identifying and solving problems in your test suite.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
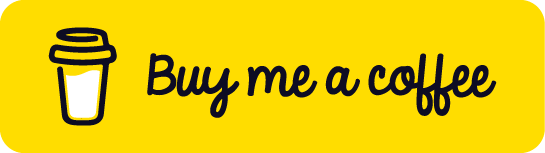
Chat with Chuck
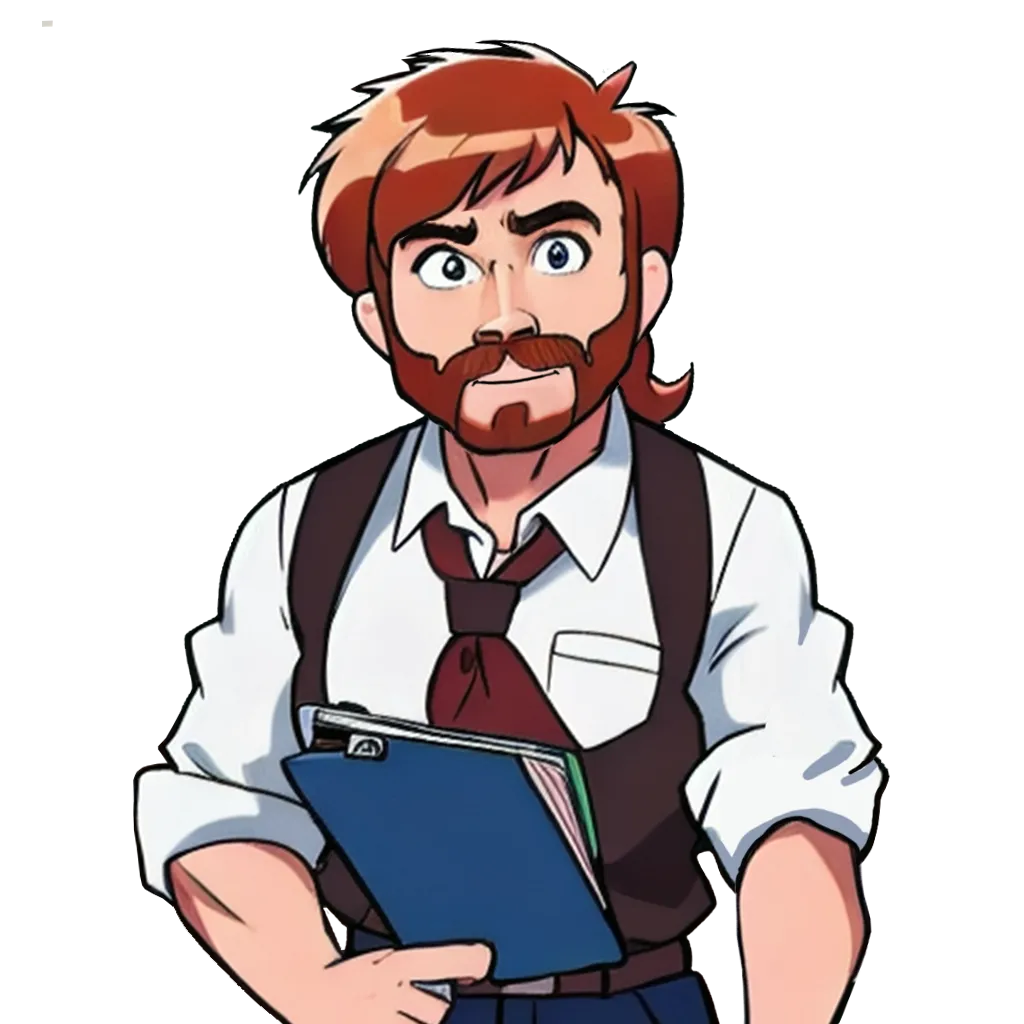
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest