Testing JavaScript and DOM with Jest
Fundamentals of the DOM
Understanding the Fundamentals of the DOM
The Document Object Model (DOM) is a cross-platform interface that treats an HTML or XML document as a hierarchical structure of nodes. This section will provide you with a solid foundation on what the DOM is and how to interact with it using JavaScript.
What is the DOM?
The DOM is an in-memory representation of a web document. When a browser loads a web page, it converts the HTML into a DOM that can be manipulated with JavaScript. The DOM structures the document in the form of a tree of nodes, where each HTML element becomes a node.
Main Components of the DOM
- Document (Document Object): The root object representing the entire web page.
- Elements (Element Nodes): Represent the HTML tags and form the structure of the document.
- Attributes (Attribute Nodes): Associated with elements, representing the properties of HTML tags.
- Text (Text Nodes): Textual content within elements.
Accessing and Manipulating the DOM with JavaScript
We can use JavaScript to access and manipulate DOM nodes. Some of the most common functions include:
document.getElementById(id)
: Accesses an element by its ID.document.getElementsByClassName(className)
: Accesses all elements with a specific class.document.querySelector(selector)
: Selects the first element that matches a CSS selector.document.querySelectorAll(selector)
: Selects all elements that match a CSS selector.
html
Creating and Modifying Elements
In addition to accessing existing nodes, we can also create and modify elements within the DOM.
document.createElement(tagName)
: Creates a new element node.element.appendChild(newNode)
: Adds a child node to an existing element.element.setAttribute(attribute, value)
: Sets an attribute for an element.
html
DOM Events
Events are a fundamental part of the DOM, allowing web pages to respond to user interactions. You can use addEventListener
to bind handler functions to element events.
html
Advanced Interaction with the DOM
Manipulating the DOM isn't limited to changing text or handling events. You can also modify styles, remove nodes, clone nodes, and more.
- Modify Styles:
element.style.property = value;
- Remove Nodes:
element.removeChild(node);
- Clone Nodes:
element.cloneNode(deep);
html
With a firm understanding of the DOM, you are ready to start writing tests to verify proper DOM manipulation. In the next section, we'll learn how to install and configure Jest, the tool we'll be using for our JavaScript tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
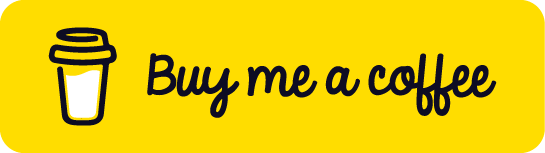
Chat with Chuck
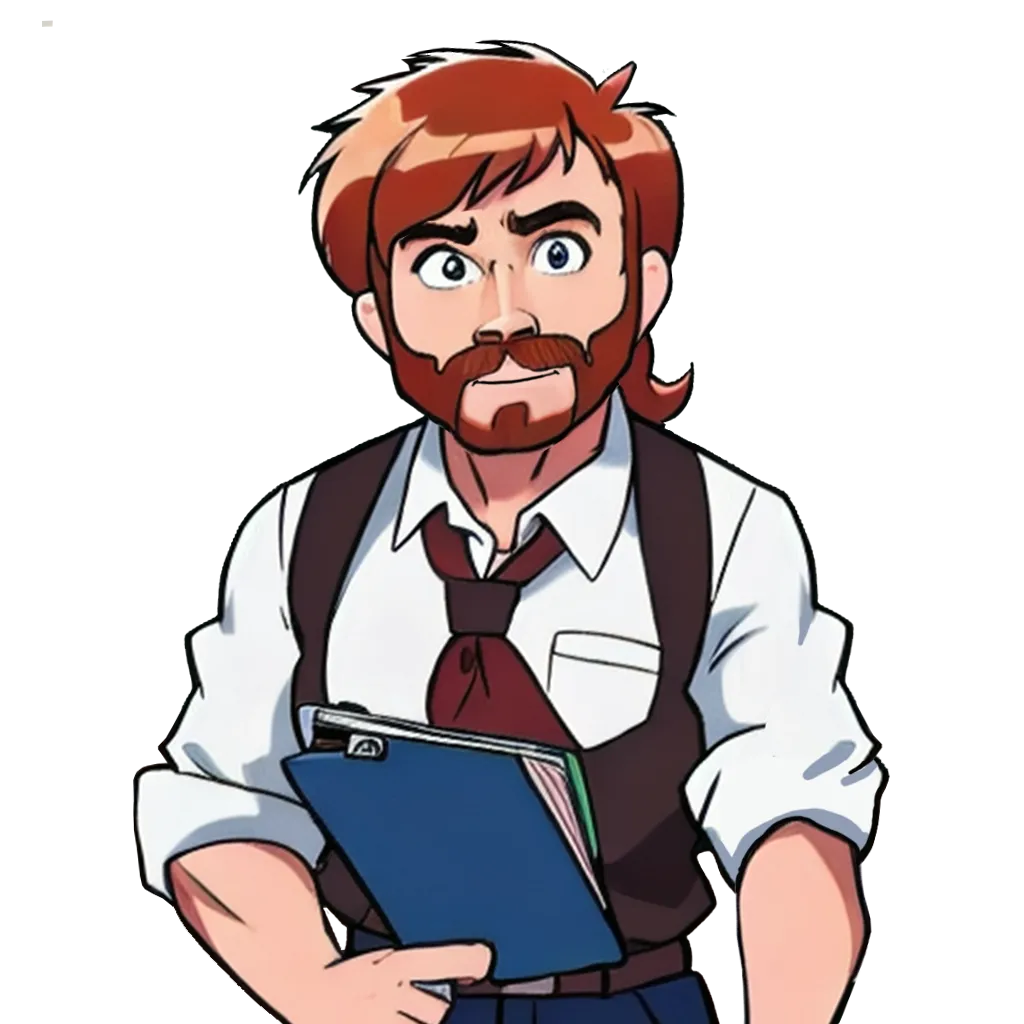
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest