Testing JavaScript and DOM with Jest
Async Testing with Jest
Async Testing with Jest
Modern applications often rely on asynchronous operations such as HTTP requests, timers, and promises. Testing these behaviors is crucial to ensure your code handles asynchrony properly. In this section, we will learn how to perform asynchronous tests using Jest.
Testing Promises
Jest provides several ways to handle promises and asynchronous tests effectively.
- Testing with
.then()
andcatch()
:
javascript
javascript
- Using
async/await
:
javascript
- Handling Rejected Promises:
If you want to test a rejection, you can use expect.assertions
to ensure that at least one assertion is called in your tests:
javascript
Testing HTTP Calls
Simulating HTTP calls is a common practice in async testing. You can use jest.mock
to mock modules like axios
or fetch
.
- Using
jest.mock
to Mock Modules:
javascript
javascript
Testing Timers and Asynchronous Functions
Jest allows you to control time in your tests using methods like jest.useFakeTimers()
and jest.runAllTimers()
.
- Simulating Timers:
javascript
javascript
Testing Asynchronous Operations in React
With React components, you can use @testing-library/react
along with Jest to test asynchronous effects.
- Simulating Fetch in React Components:
javascript
- Testing React Components with Async Data:
javascript
Placeholder for image: [A flowchart showing how Jest handles asynchronous operations with promises, async/await, and timers]
With these examples, you now have a solid understanding of how to handle asynchronous tests in Jest. In the next section, we will explore organizing and structuring tests in Jest to keep your test suite clean and manageable.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
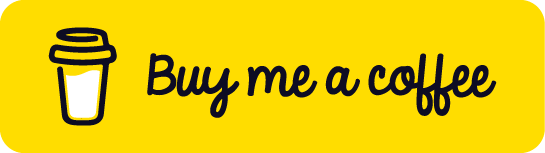
Chat with Chuck
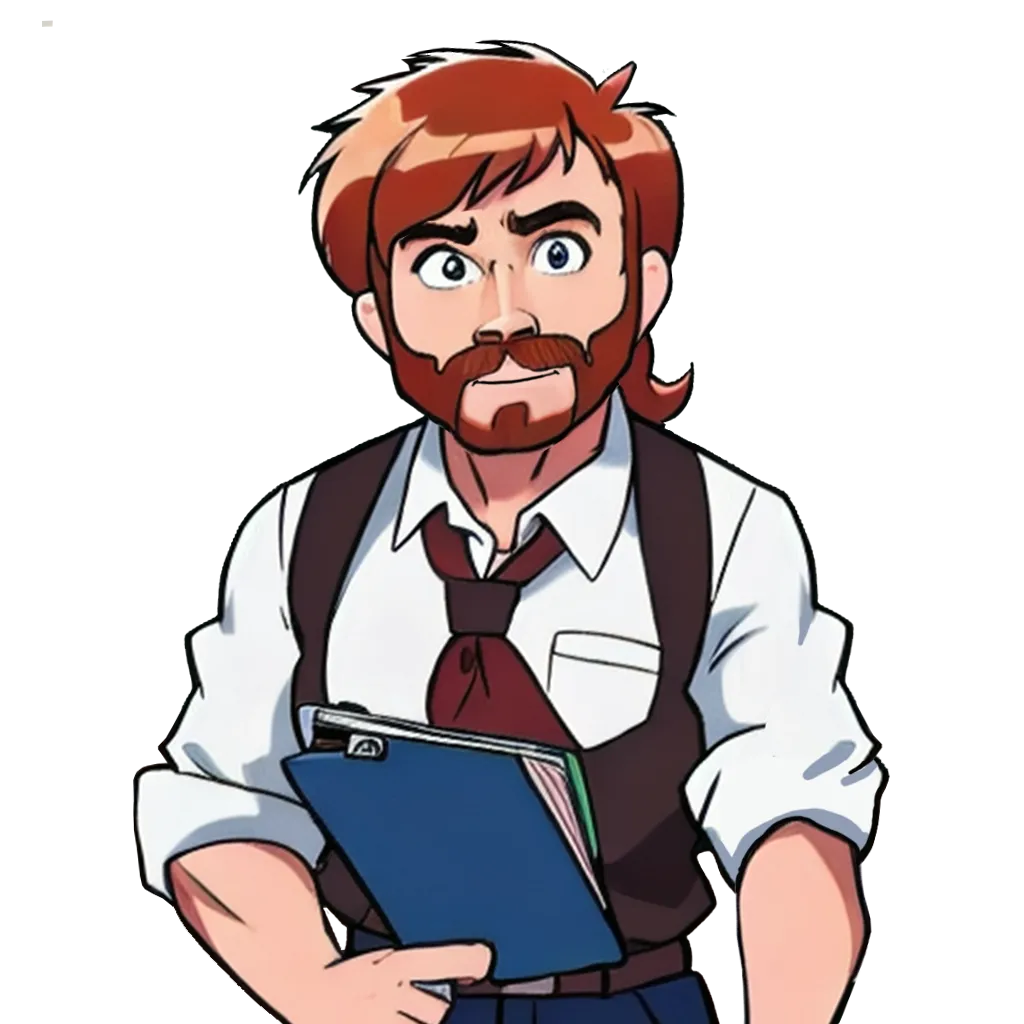
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest