Testing JavaScript and DOM with Jest
DOM Component Testing with Jest
DOM Component Testing with Jest
In this section, we will learn how to write and execute tests that interact with the DOM using Jest along with a test environment that simulates the browser. This is essential to verify that our components behave as expected on a web page.
Configuring the Test Environment for the DOM
Jest uses jsdom
by default, which simulates a browser environment within Node.js. This allows you to interact with the DOM in your tests without the need for a real browser.
First, make sure you have a basic project structure with Jest installed. If you haven't done so yet, follow the Jest installation and configuration steps mentioned earlier.
Create a Simple Component to Test
Suppose you have a simple HTML component that contains a button and a text box. When the button is clicked, the content of the text box changes:
html
javascript
Writing Tests for the DOM Component
To test this component, you need to simulate the browser environment. You can create a test file that sets up a simulated DOM and performs interactions on it.
First, set up your test file:
javascript
Interacting with DOM Elements
In tests involving DOM components, you will often need to interact with elements, such as simulating clicks, entering text, or changing the state of checkboxes. Here is a more detailed example:
javascript
Placeholder for image: [A diagram showing how Jest and jsdom work together to simulate the DOM and run tests]
Plugins and Utilities for DOM Testing
To facilitate testing of more complex components, you can use libraries like @testing-library/react
(for React), which provides an intuitive API for interacting with the DOM in your tests:
bash
Here is an example of how you could use these libraries to test a React component:
javascript
javascript
With these examples and principles, you now have the tools to write effective tests that interact with the DOM. In the next section, we will learn about testing DOM events with Jest and how to ensure our events work correctly within the user interface.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
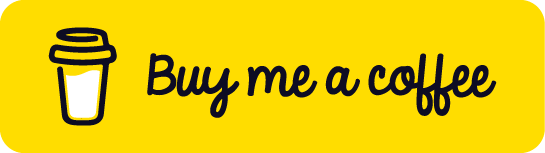
Chat with Chuck
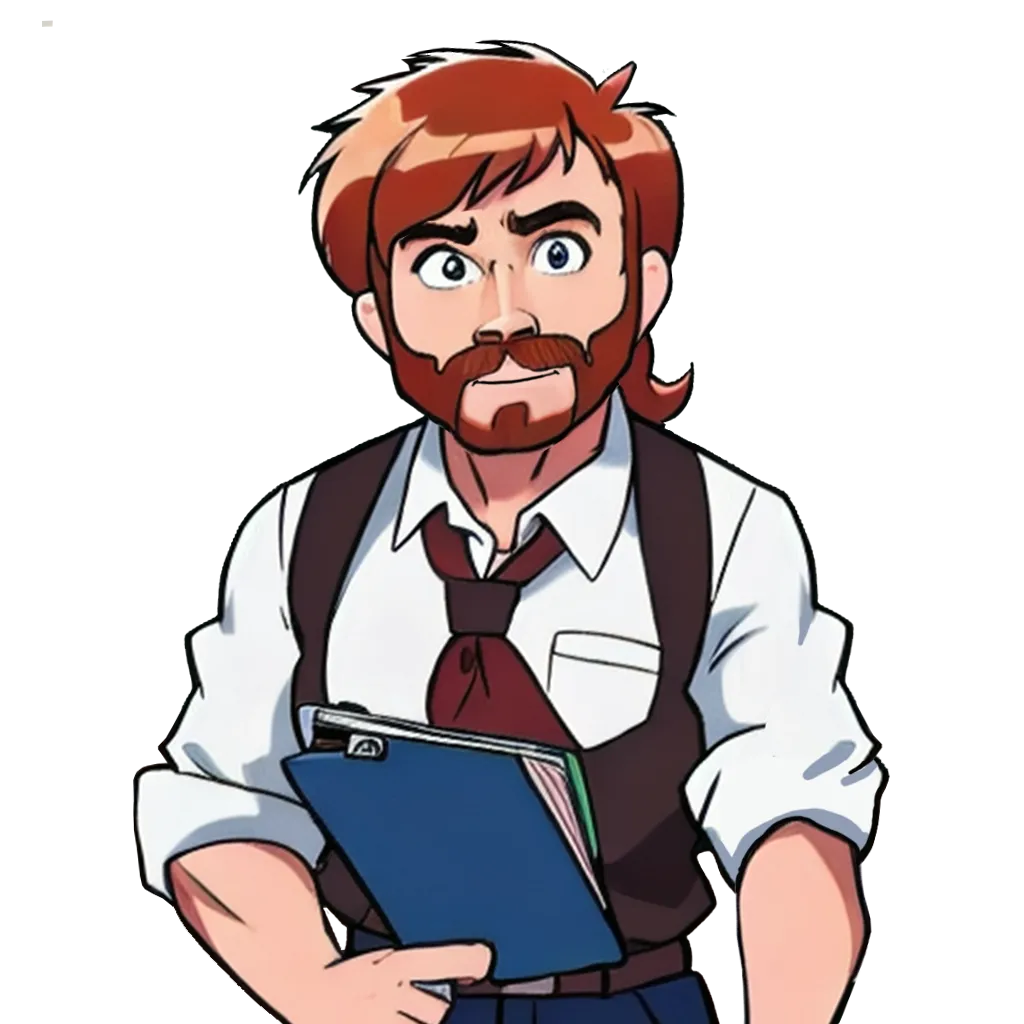
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest