Testing JavaScript and DOM with Jest
DOM Event Testing with Jest
DOM Event Testing with Jest
Events are fundamental pieces in the development of interactive web applications. In this section, we will learn how to test DOM events with Jest to ensure our application responds correctly to user interaction.
Setting Up the Environment
Let's make sure our testing environment is ready to handle DOM events. If you don't have Jest set up in your project yet, review the previous chapters for Jest installation and basic configuration.
Creating a Component with Events
Suppose we have an HTML component with a button that, when clicked, changes the content of a text box:
html
javascript
Writing Tests for DOM Events
Let's write a test to verify that the click
event works as expected. Creating a test file and setting up the simulated DOM is essential:
javascript
Using fireEvent from Testing Library
For more comprehensive and descriptive event handling, you can use fireEvent
from @testing-library/dom
. Install the library if you haven't already:
bash
Let's see how to use fireEvent
to test our component:
javascript
Testing Other Types of Events
Jest and @testing-library/dom
allow testing a wide variety of DOM events, such as input changes, form submissions, mouse movements, etc. Here are some examples:
- Change event on an input:
javascript
- Submit event on a form:
javascript
Placeholder for image: [A flowchart diagram showing event simulation using Jest and @testing-library/dom
]
With the knowledge of how to test DOM events, you are well-equipped to ensure your application responds appropriately to user interaction. In the next section, we will explore the use of mocking and stubbing in Jest for more sophisticated and controlled tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
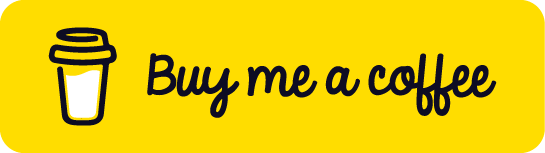
Chat with Chuck
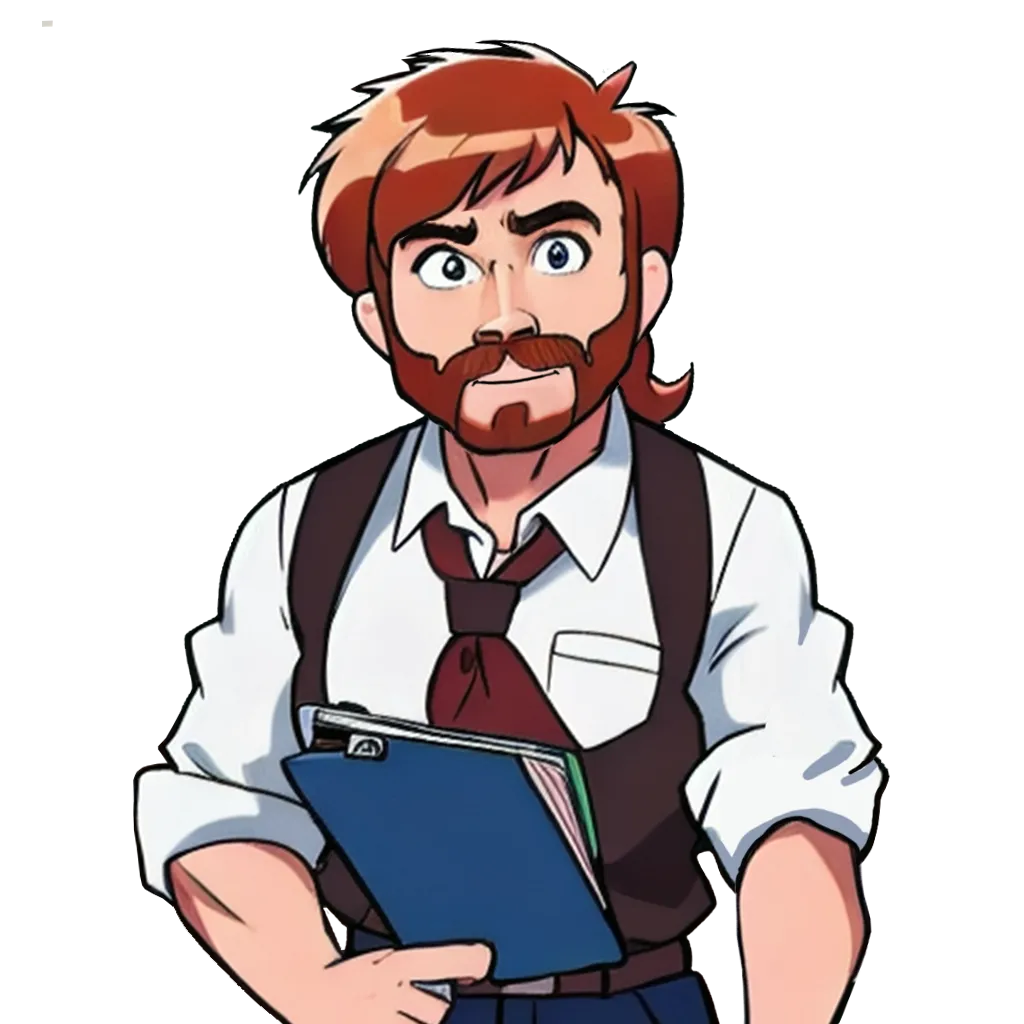
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest