Testing JavaScript and DOM with Jest
Writing Your First Unit Tests with Jest
Writing Your First Unit Tests with Jest
Now that you have installed and configured Jest, it's time to dive into writing unit tests. This chapter will guide you through the basics and teach you how to write and run your first unit tests.
What is a Unit Test?
A unit test is an automated test that verifies the behavior of a small "unit" of code, typically a function or a method. The goal is to ensure that the unit of code produces the expected results for a predefined set of conditions.
Skeleton of a Unit Test with Jest
Tests in Jest are organized using test
or it
functions. A basic test follows this structure:
javascript
Writing a Simple Unit Test
Let's write a unit test for a simple function that sums two numbers. Suppose we have the following code in a file called sum.js
:
javascript
Create a folder for tests and a test file:
Now, let's write the unit test in sum.test.js
:
javascript
This test verifies that the sum
function returns 3 when passed 1 and 2 as arguments.
Using Matchers
Jest provides multiple matchers to verify different types of values. Here are some common matchers:
- toBe: Exact equality.
- toEqual: Object or array equality.
- toBeNull: Checks if the value is
null
. - toBeDefined: Checks if the value is defined.
- toBeTruthy: Checks if the value is truthy in a boolean context.
- toContain: Checks if an array or string contains a specific value.
Example of using different matchers:
javascript
Grouping Tests with Describe
To better organize your tests, you can group them using the describe
block. This is useful for grouping related tests:
javascript
Setup and Teardown
Sometimes, you need to perform some setup before a test runs or cleanup after a test finishes. Jest provides beforeEach
, afterEach
, beforeAll
, and afterAll
methods for these purposes:
javascript
Running Tests
To run the tests, simply use the command:
bash
[Jest will search for and execute all defined tests and generate a detailed report with the results].
Generating Code Coverage Reports
To generate a code coverage report, you can use the --coverage
flag:
bash
[This will generate a coverage report showing which parts of your code are covered by the tests].
With these basic concepts and examples, you are ready to start writing and running your own unit tests with Jest. In the next section, we will cover testing DOM components using Jest and how you can verify the correct manipulation of the user interface.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
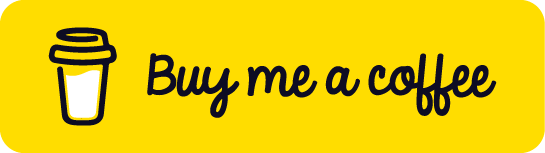
Chat with Chuck
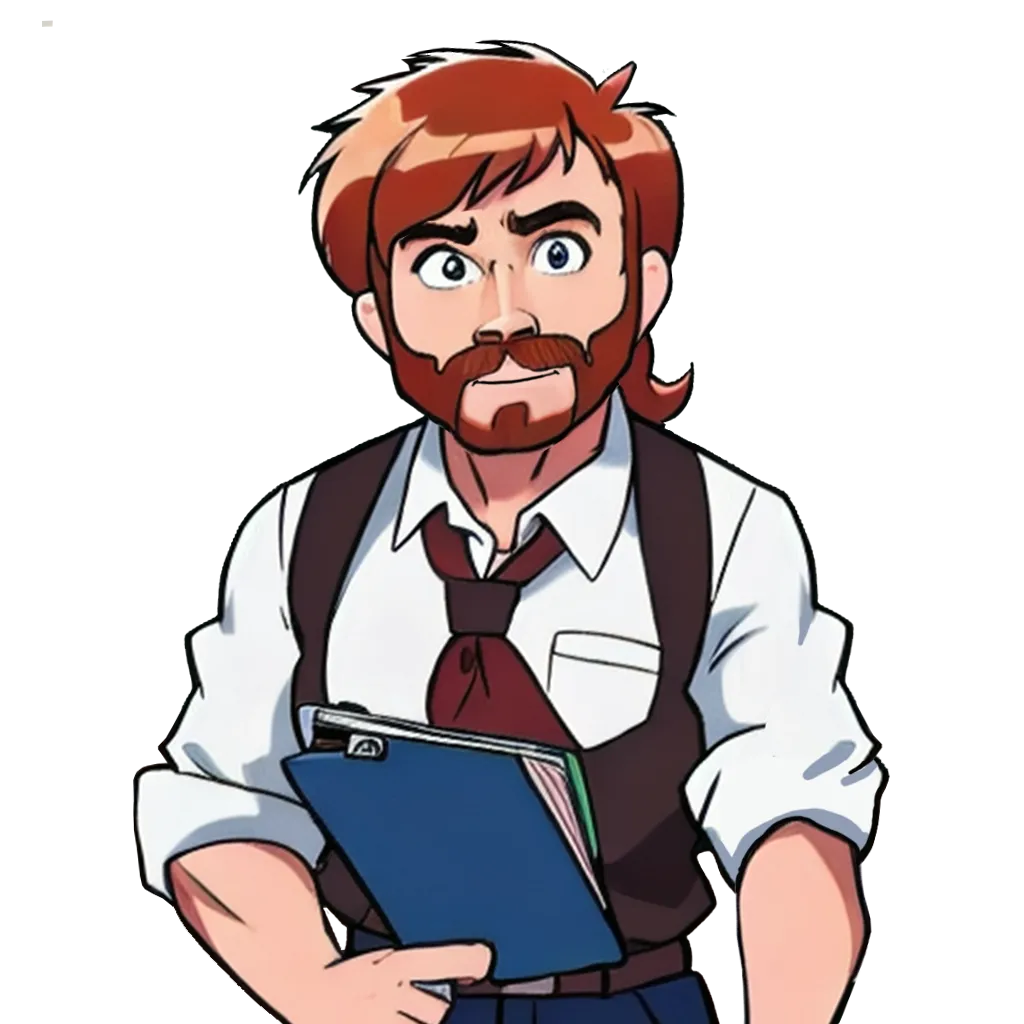
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest