Testing JavaScript and DOM with Jest
Installation and Configuration of Jest
Getting Ready to Use Jest: Installation and Configuration
In this section, we will learn how to install Jest and configure it properly to start writing and running tests in our JavaScript projects.
Prerequisites
Before continuing, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download and install Node.js from here.
Installing Jest
To install Jest, you can use npm. Open your terminal or command line and navigate to your project directory. Then, run the following command to install Jest as a development dependency:
bash
Alternatively, if you prefer to use yarn, you can install Jest with the following command:
bash
Basic Jest Configuration
Once Jest is installed, it's advisable to add test scripts in your package.json
file to facilitate running the tests. Open package.json
and add the following script in the "scripts" section:
json
This will allow you to run Jest using the command:
bash
Test File Structure
It is a good practice to keep the tests in a separate directory or follow a specific naming convention to differentiate them from the source code. Some common conventions include:
- Placing the test files in a
__tests__
directory. - Naming the test files with the same name as the file they are testing, followed by
.test.js
or.spec.js
.
For example:
Advanced Jest Configuration
Jest allows for extensive configuration through a jest.config.js
file or directly in the package.json
. Here is an example of a basic configuration in jest.config.js
:
javascript
Configuration in package.json
You can also configure Jest directly in your package.json
:
json
Complete Configuration Example
Below is a complete example that includes installation, configuration, and a basic test case to ensure Jest is working correctly.
- Initialize a new project:
bash
- Install Jest:
bash
- Configure Jest in package.json:
json
- Create directory and file structure:
- Write the function code to be tested in
sum.js
:
javascript
- Write the unit test in
sum.test.js
:
javascript
- Run the tests:
bash
[Jest will search for and execute all defined tests, producing a detailed report with the results].
With Jest installed and configured, we are ready to start writing our first unit tests. In the next section, we will explore how to write and run unit tests with Jest. Continue to deepen your testing experience with Jest!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
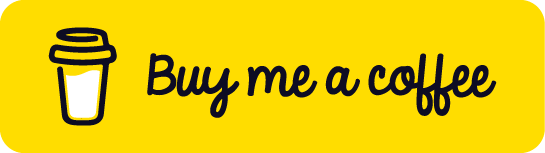
Chat with Chuck
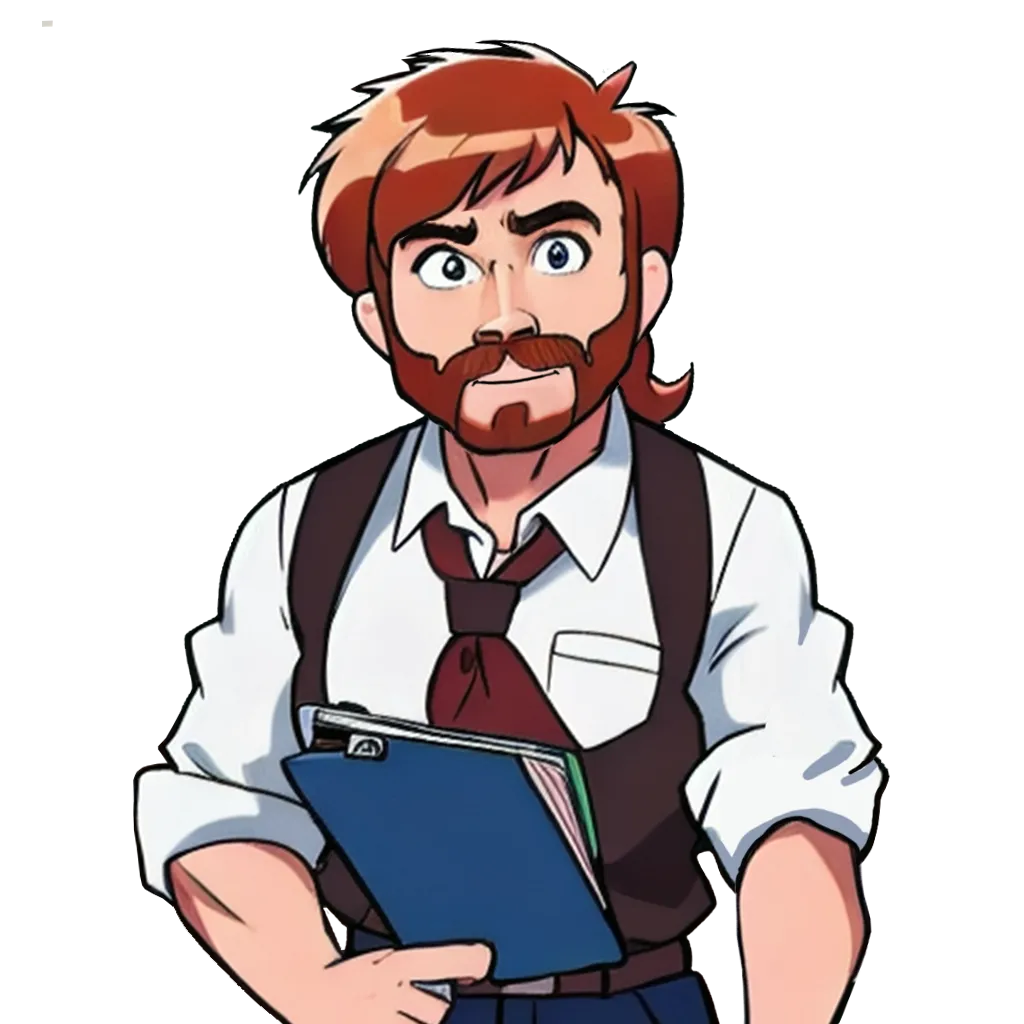
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest