Testing JavaScript and DOM with Jest
User Interaction Testing with Jest
User Interaction Testing with Jest
In this chapter, we will learn how to simulate and test user interactions in our application using Jest along with additional tools such as Testing Library. These tests are essential to ensure that the user interface responds adequately to user actions.
Introduction to Testing Library
Testing Library is a collection of utilities for UI testing, designed to work effortlessly together with Jest. These tools provide a simple and powerful API to interact with DOM elements and verify their behavior.
First, install Testing Library if you haven't already:
bash
Basic Setup
To start, write tests that simulate user interactions such as clicks, text inputs, and form submissions. Below is an example of basic setup and integration of Testing Library with Jest:
javascript
Interaction Test Example
Suppose we have a simple form in our application:
html
The following script handles the form interactions:
javascript
Now, let's write tests to verify user interactions:
javascript
Testing Various Types of Interaction
Here are additional examples of how to simulate different user interactions:
- Clicks:
javascript
- Text Inputs:
javascript
- Selectors and Options:
javascript
- Checkboxes and Radio Buttons:
javascript
- Form Submission:
javascript
Placeholder for image: [A diagram illustrating the event flow of Testing Library interacting with the DOM]
With these tools and examples, you can start effectively testing user interactions in your application. In the next section, we will explore how to write accessibility tests with Jest to ensure our application is usable for all users.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
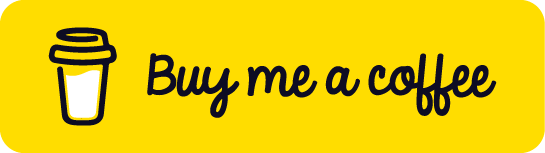
Chat with Chuck
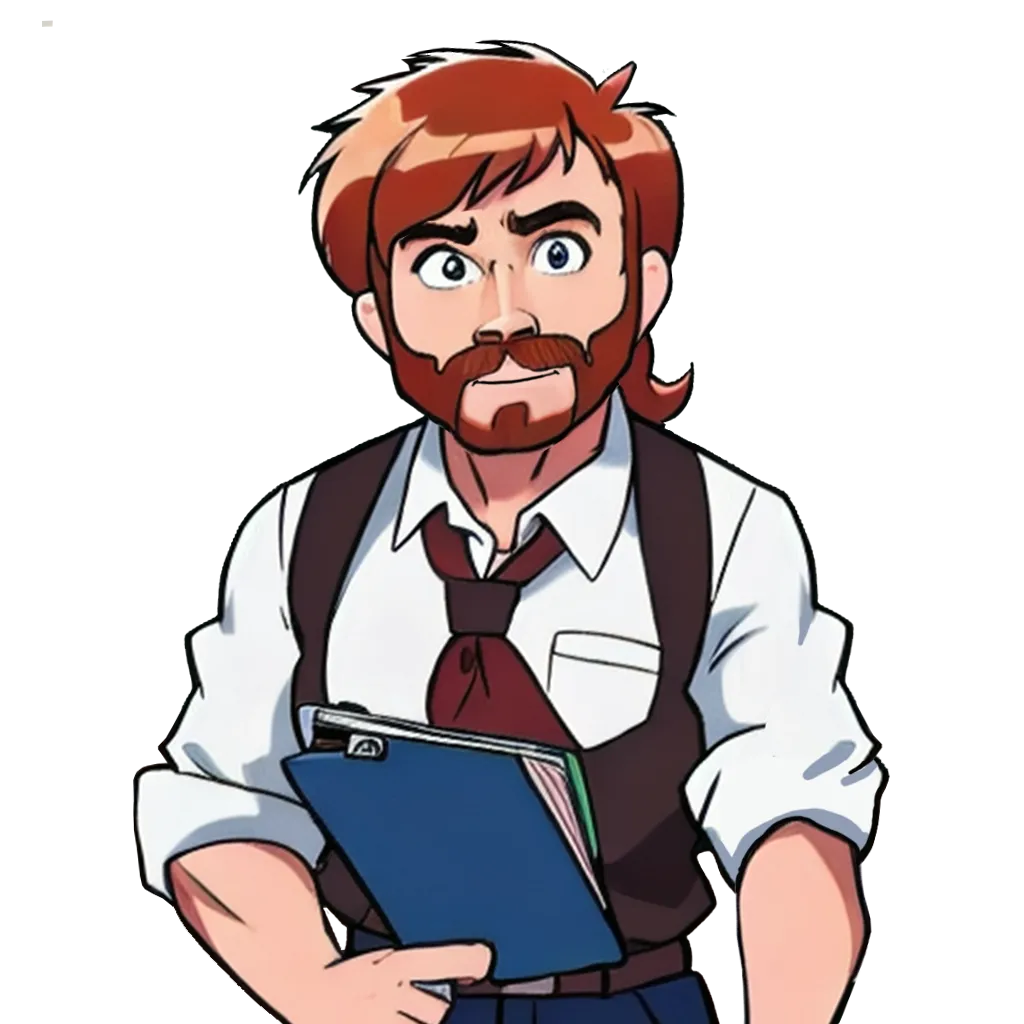
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest