Testing JavaScript and DOM with Jest
Mocking and Stubbing in Jest
Mocking and Stubbing in Jest
In the world of testing, mocking and stubbing are essential techniques for isolating the code under test and controlling its dependencies. This section will explain what these techniques are and how to use them with Jest.
What is Mocking and Stubbing?
- Mocking: Involves creating fake objects that simulate the behavior of real objects. This is used to test components in isolation.
- Stubbing: Similar to mocking, but focuses more on replacing specific functions within objects with controlled implementations.
Mock Functions in Jest
Jest provides mocking functions that allow you to spy on the behavior of existing functions or replace them with simulations.
- Creating a Mock Function:
javascript
- Mocking Functions with Simulated Implementations:
You can provide a simulated implementation to a mock function using mockImplementation
.
javascript
- Mocking Entire Modules:
You can mock entire modules using jest.mock
. For example, suppose you have an api.js
module:
javascript
You can mock this module in your tests:
javascript
Mocking Asynchronous Functions
You can mock asynchronous functions to simulate the resolution or rejection of promises:
javascript
Mocking Instance Methods
If you want to mock methods of a specific class, you can use jest.spyOn
or overwrite the method directly:
javascript
Stubbing in Jest
To stub functions, you can temporarily replace their implementations:
javascript
Using Mock Clear and Reset
To avoid conflicts between tests, you can clear and reset mocks using mockClear
or mockReset
.
javascript
Full Example
Below is a complete example demonstrating mocking and stubbing for a function that depends on an API call:
javascript
With these mocking and stubbing techniques, you can isolate the code under test and simulate different controlled scenarios. In the next section, we will address user interaction testing with Jest, thus increasing our ability to ensure the quality and interactiveness of our application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
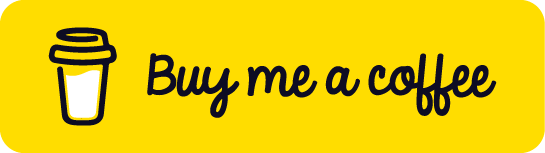
Chat with Chuck
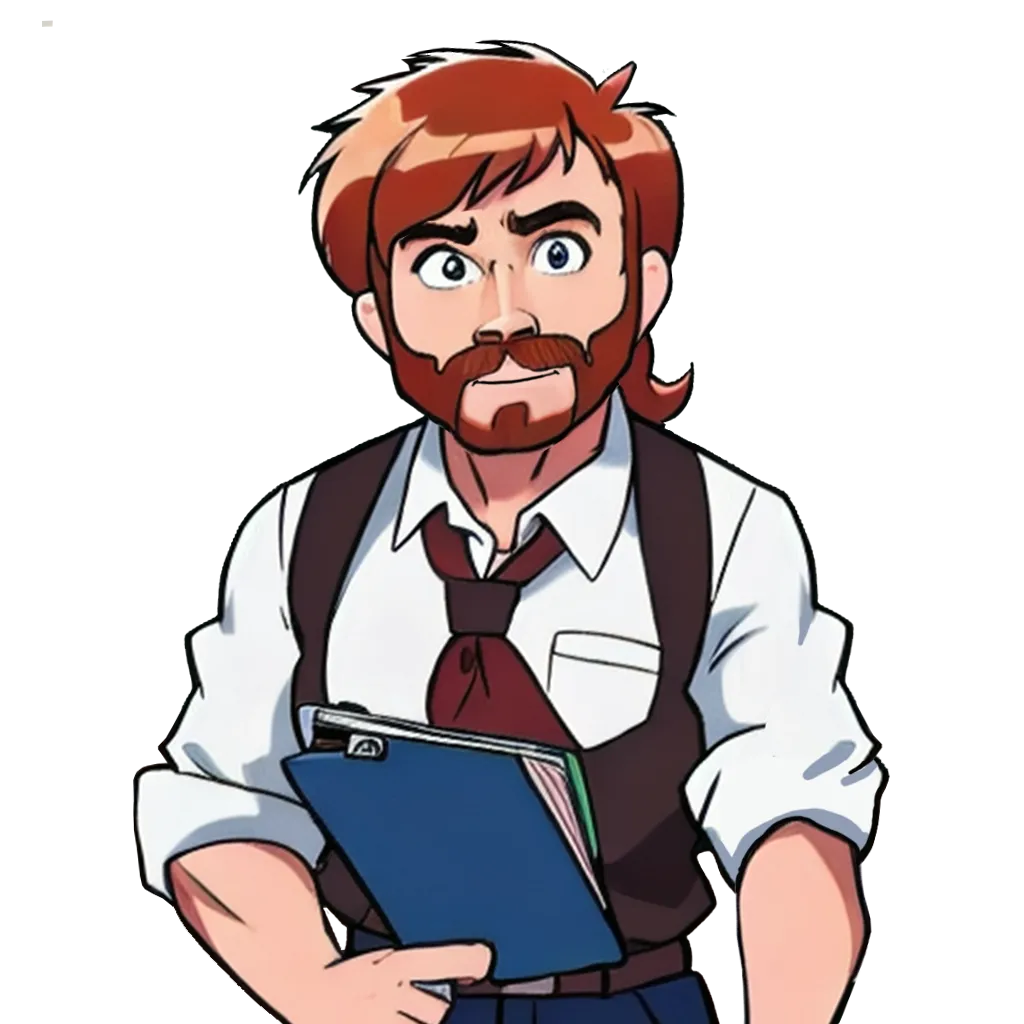
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest