Testing JavaScript and DOM with Jest
Organization and Structure of Tests in Jest
Organization and Structure of Tests in Jest
Maintaining good organization and structure in your test suite is crucial for facilitating the maintenance, scalability, and clarity of the project. In this section, we will learn the best practices for organizing and structuring your tests in Jest.
Divide and Conquer
A general rule is to keep test files close to the files they are testing. This makes it easier to find the tests when working with the code. There are several common strategies:
- Place tests in a
__tests__
folder:
- Place tests next to the source files:
Both structures are valid; the choice depends on the team's preferences and the project scope.
Test Files
Properly naming test files is essential. Some common conventions include:
- Naming test files with the same name as the files they are testing, followed by
.test.js
or.spec.js
.- Example:
component.js
->component.test.js
- Example:
Using Describe to Group Tests
Jest's describe
block allows you to group related tests, making organization and clarity easier.
javascript
Test Lifecycle Hooks
Jest's hooks beforeAll
, beforeEach
, afterEach
, and afterAll
allow you to set up and clean up the state before and after each test or test suite.
javascript
Creating Configuration Files
To keep your Jest configuration organized, use a separate configuration file (jest.config.js
), especially for large projects with custom settings.
javascript
Setup Files Configuration
Setup files are ideal for global configurations you need to execute before all tests.
javascript
Sample Project Directory Structure
A well-organized directory structure might look like this:
Placeholder for image: [A diagram presenting a sample project structure with directories and files organized for testing with Jest]
Using Plugins and Extensions
Jest has a variety of plugins and extensions that can enhance your workflow:
-
jest-extended: Adds additional matchers.
bashjavascript -
jest-serializer: Serializes objects the way you want.
-
jest-dom: Offers DOM-specific matchers for testing library.
Organization Exercises
- Create tests for a calculation module:
javascript
javascript
With these organizational practices, you will enhance the clarity, maintainability, and scalability of your test suite in Jest. In the next section, we will explore how to automate tests with CI/CD using Jest, ensuring a continuous and efficient development workflow.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
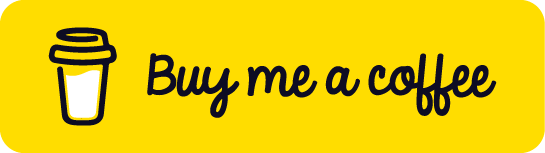
Chat with Chuck
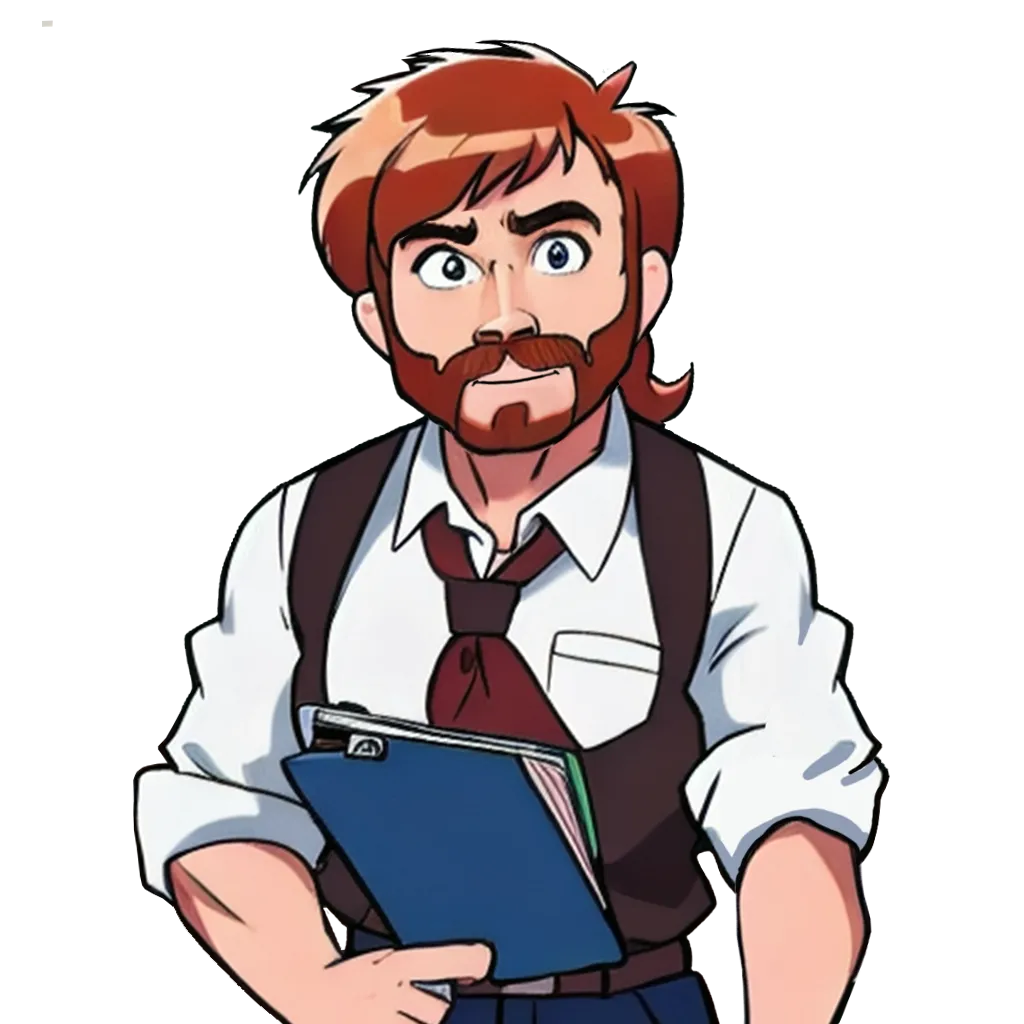
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest