Testing JavaScript and DOM with Jest
Debugging Failed Tests in Jest
Debugging Failed Tests in Jest
Failed tests can be frustrating, but understanding how to debug them efficiently is crucial to maintaining your application's health and your test suite. In this section, we will learn various techniques and tools for debugging failed tests in Jest.
Understanding Error Messages
-
Clear Error Messages: When a test fails, Jest provides a clear error message, which includes the test name, the expected and received difference, and a stack trace to track where the error occurred.
bash
Using console.log
and console.error
Inserting console.log
or console.error
inside your functions and tests can help you trace the state and flow of data.
javascript
Describing Multiple Cases with describe
and test
Use test groups (describe
) and break down test cases into smaller units. This helps isolate the cause of failing tests.
javascript
Using test.only
and test.skip
During debugging, you can isolate a particular test using test.only
. On the other hand, test.skip
temporarily skips irrelevant tests.
javascript
Debugging with Development Tools
You can use JavaScript debuggers like Chrome, VSCode, or any other environment that supports Node.js.
-
VSCode Debugger:
- Configure the debug file
launch.json
:
json- Add a
debugger
in your code:
javascript- Run the debug configuration from VSCode, set breakpoints, and inspect variables.
- Configure the debug file
Using jest.spyOn
and jest.fn
Spies and mock functions can help you better understand how functions are being executed and ensure they are called with the correct arguments.
javascript
Code Coverage Analysis
Use code coverage analysis to identify parts of the code that are not being tested and that might be causing errors.
bash
This command will generate a coverage report, showing the lines of code that have been executed during tests. You can find the report files in the coverage
directory.
Debugging Asynchronous Tests
Ensure that asynchronous tests do not finish before asynchronous operations are completed by using async/await
or returning promises in your tests.
javascript
Complete Debugging Example
Below is a comprehensive example incorporating various debugging techniques:
javascript
Placeholder for Image: [An illustrated diagram showing the flow from running tests in Jest to debugging using different techniques like console.log
, breakpoints in VSCode, and spies.]
With these debugging techniques, you can identify and resolve issues more efficiently in your Jest tests. In the next section, you will recap what you've learned and see the next steps to further improve your expertise in testing with Jest.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
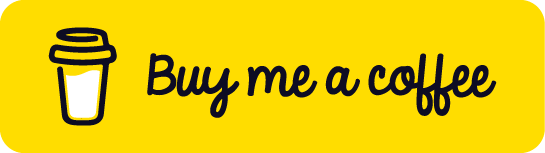
Chat with Chuck
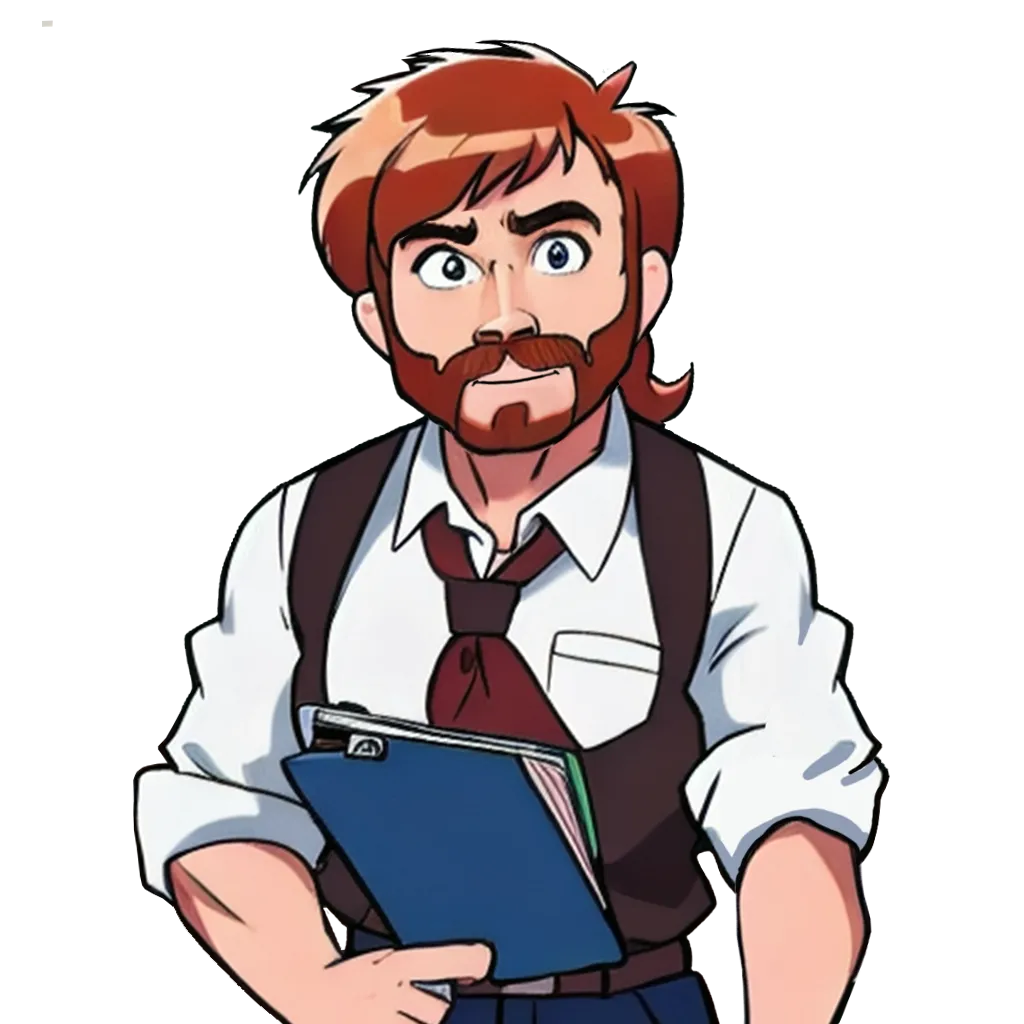
- Introduction to Testing in JavaScript with Jest
- Fundamentals of the DOM
- Installation and Configuration of Jest
- Writing Your First Unit Tests with Jest
- DOM Component Testing with Jest
- DOM Event Testing with Jest
- Mocking and Stubbing in Jest
- User Interaction Testing with Jest
- Accessibility Testing with Jest
- Async Testing with Jest
- Organization and Structure of Tests in Jest
- Test Automation with CI/CD using Jest
- Best Practices for Testing with Jest
- Debugging Failed Tests in Jest
- Conclusions and Next Steps in Testing with Jest