GraphQL with Node
Authentication and Authorization in GraphQL
Authentication and authorization are crucial aspects for the security of any API. Authentication verifies the identity of the user, while authorization checks the permissions to perform certain actions. In this chapter, we will learn how to implement both in a GraphQL API using JWT (JSON Web Tokens).
Installing Dependencies
First, we will install jsonwebtoken
and bcryptjs
to handle JWT tokens and password encryption, respectively.
bash
Configuring Authentication with JWT
1. Creating Users and Login
First, we define a User
model to store user data.
User Model (models/User.js
):
javascript
2. Defining the Schema with Authentication Mutations
We update the schema to include register
and login
mutations.
Schema (schema.js
):
graphql
3. Implementing Authentication Resolvers
We implement the resolvers for the register
and login
mutations.
Resolvers (resolvers.js
):
javascript
Middleware for Authentication
Update server.js
to include middleware that will authenticate requests and add user data to the context.
Server (server.js
):
javascript
Example Usage in GraphiQL
Registering a New User
graphql
Logging In
graphql
Use the obtained JWT token in subsequent requests for authentication:
graphql
Creating a Book (Authenticated)
graphql
Summary
In this chapter, we have learned to:
- Configure authentication using JWT in GraphQL.
- Create and authenticate users through mutations.
- Add middleware to handle authentication and authorization.
- Use JWT tokens to protect our mutation resolvers.
These concepts are fundamental for creating secure applications and maintaining data integrity. In the next chapter, we will explore pagination and filtering in GraphQL.
[Placeholder: Diagram showing the authentication and authorization flow in a GraphQL application, from the submission of credentials to performing authenticated queries and mutations]
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps
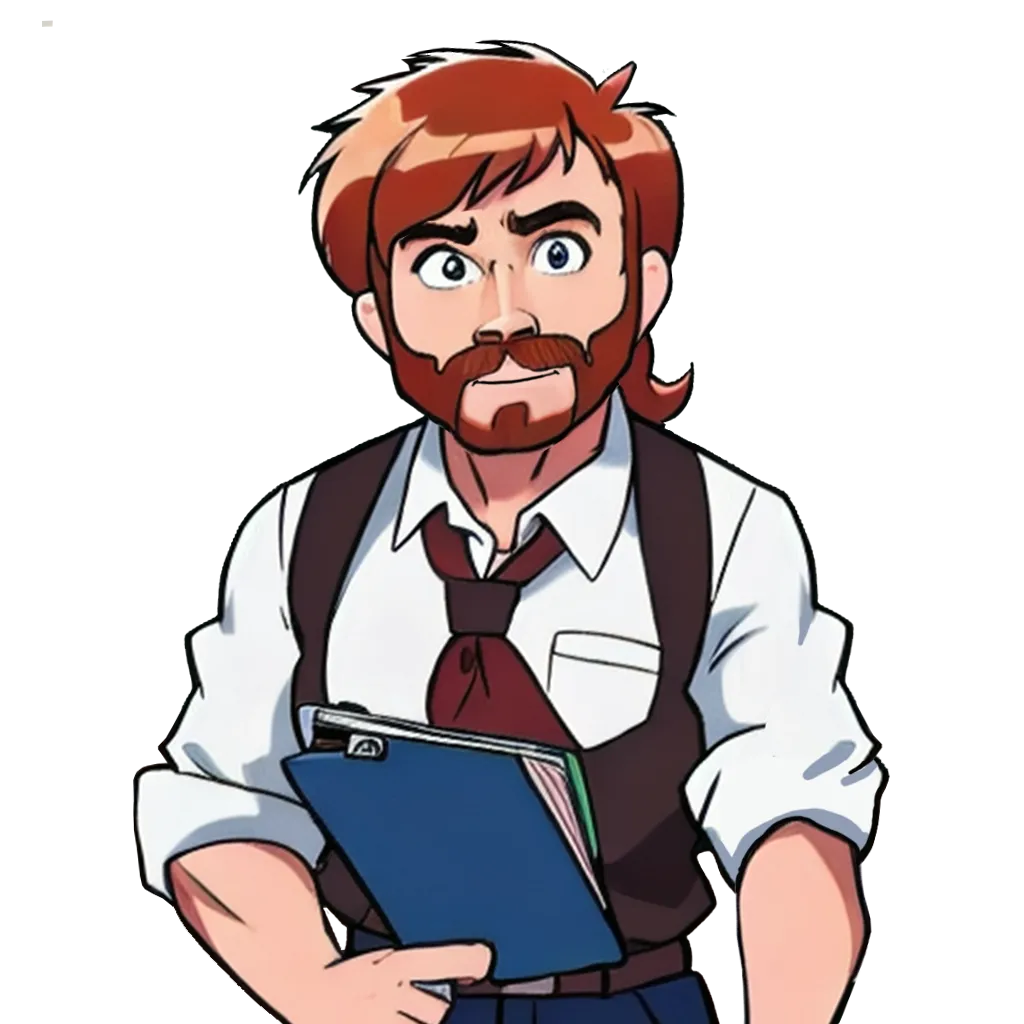