GraphQL with Node
Creating Mutations in GraphQL
Mutations in GraphQL allow clients to modify data on the server, similar to how POST, PUT, and DELETE methods work in REST APIs. In this chapter, we will learn how to define and use mutations in GraphQL.
Defining Mutations in the Schema
In GraphQL, mutations are defined as part of the Mutation
type. Mutations allow performing write operations such as creating, updating, or deleting records.
Schema Example with Mutations
Using our library API as an example:
graphql
In this schema, we define three mutations:
createBook
: To create a new book.updateBook
: To update an existing book.deleteBook
: To delete a book.
Implementing Resolvers for Mutations
Each mutation in the schema must have a corresponding resolver that handles the logic of the write operation.
Example Implementation of Resolvers
In our resolvers.js
file, we would implement the resolvers for the mutations as follows:
javascript
Performing Mutations from the Client
Once mutations and resolvers are defined, clients can invoke mutations to modify the data on the server. GraphiQL again facilitates this process.
Example of Mutations in GraphiQL
- Mutation to create a new book:
graphql
- Mutation to update an existing book:
graphql
- Mutation to delete a book:
graphql
Error Handling
It is important to handle errors properly in the mutation resolvers to provide useful feedback to clients. You can throw custom errors using throw
in JavaScript.
Example of Error Handling
javascript
Summary
In this chapter, we have learned to:
- Define mutations in the GraphQL schema.
- Implement resolvers to handle the mutations.
- Perform mutations from the client using GraphiQL.
- Handle errors in mutation resolvers.
With these skills, you can create GraphQL APIs that not only read data but also modify it safely and efficiently. In the next chapter, we will explore the use of resolvers in GraphQL in more detail.
[Placeholder: Diagram showing how mutation resolvers connect to the schema and modify data in response to client requests]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
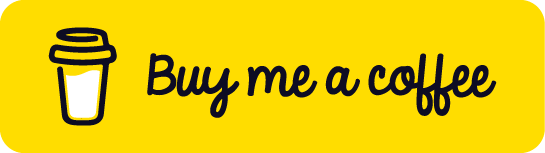
Chat with Chuck
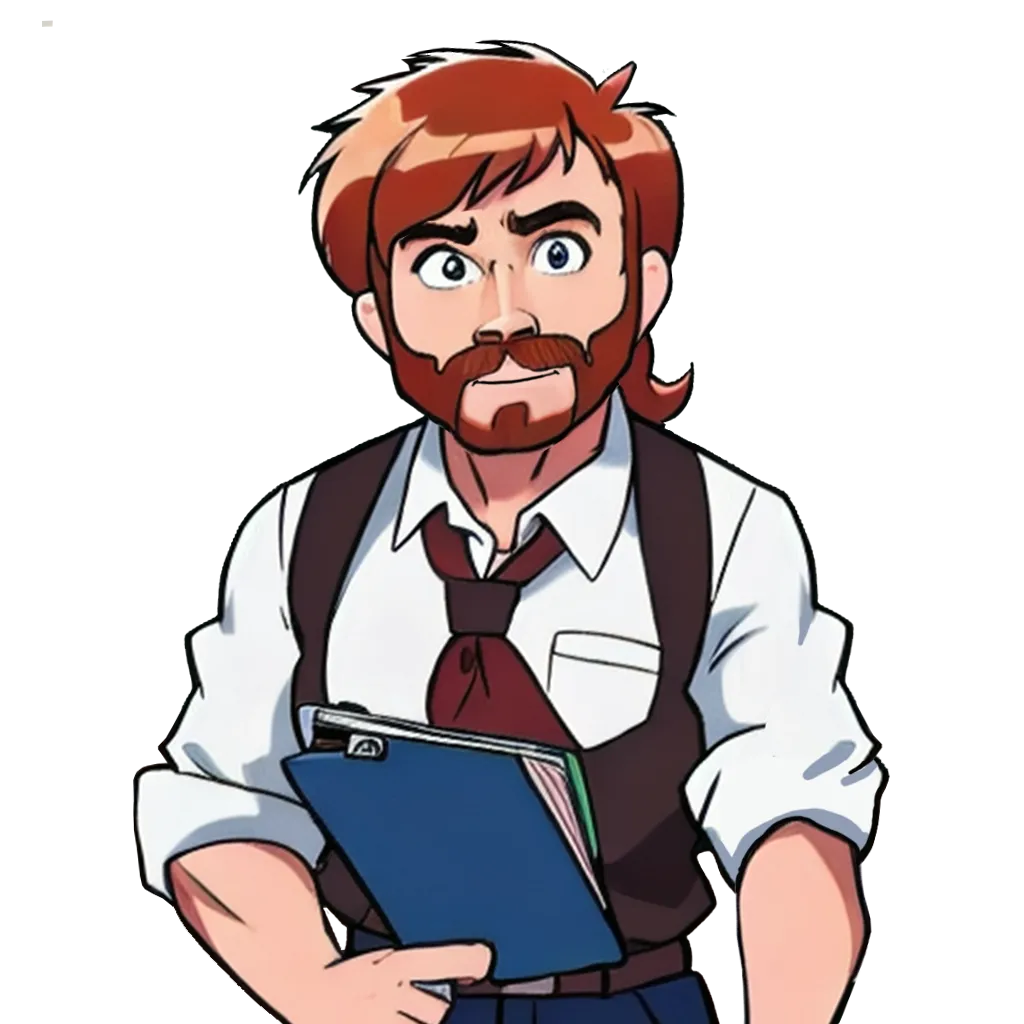
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps