GraphQL with Node
Optimization and Best Practices
Optimizing your GraphQL API and following best practices is fundamental to ensuring that your application is fast, scalable, and easy to maintain. In this chapter, we will explore various optimization strategies and some recommended best practices for working with GraphQL.
Optimization Strategies in GraphQL
1. Batching and Caching with DataLoader
DataLoader is a popular tool to avoid n+1 queries and batch requests in GraphQL. It helps to group multiple requests into a single one to improve performance.
Installation:
bash
Implementation:
DataLoader (dataloaders.js
):
javascript
Using within Resolvers (resolvers.js
):
javascript
2. Efficient Use of Context
Context is a powerful tool to share information among resolvers, helping to reduce redundancies and improve efficiency.
Example of Global Context (server.js
):
javascript
3. Database Query Optimization
Ensure to use indexes and optimize your database queries to improve performance.
Example of Indexes in Mongoose (models/Book.js
):
javascript
Best Practices in GraphQL
1. Avoid Costly Queries
Limit the amount of data a client can request in a single query to prevent abuse and improve performance.
Example of Query Limitations (schema.js
):
graphql
In the resolver, make sure to use the limit
argument:
javascript
2. Document the Schema
Document each type and field in your GraphQL schema to provide a clear reference.
Example of Documentation (schema.js
):
graphql
3. Use Fragments and Directives
Use fragments and directives to avoid repetition of fields and efficiently handle conditional queries.
Example of Fragments:
graphql
Example of Directives:
graphql
Summary
In this chapter, we explored various optimization strategies and best practices in GraphQL:
- Using DataLoader for batching and caching.
- Efficient use of context to share data among resolvers.
- Optimizing database queries using indexes.
- Implementing limitations and clear documentation in the schema.
- Using fragments and directives to improve query efficiency and clarity.
These practices will help you build efficient, scalable, and easy-to-maintain GraphQL APIs.
[Placeholder: Diagram showing best optimization practices in a GraphQL server, including the use of DataLoader, context, and database query optimization]
In the next chapter, we will explore the conclusion and next steps for further learning about GraphQL.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
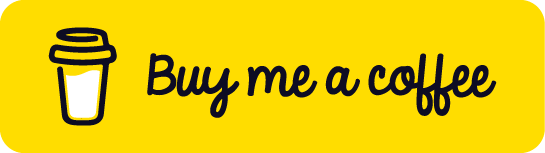
Chat with Chuck
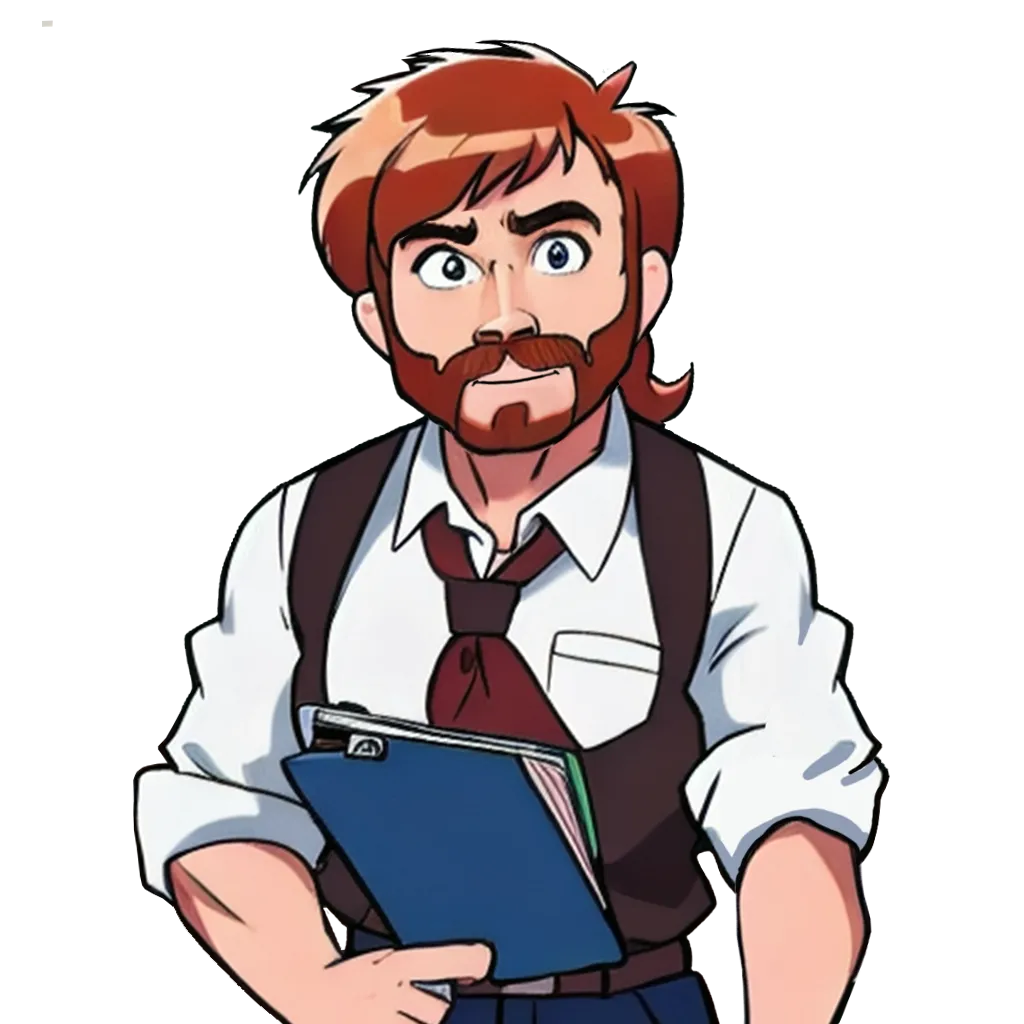
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps