GraphQL with Node
Testing GraphQL APIs
Testing GraphQL APIs is crucial to ensure they function correctly and that modifications do not introduce new errors. In this chapter, we will learn how to write tests for GraphQL APIs using tools such as Jest and Supertest.
Dependency Installation
First, we'll install the necessary dependencies for testing:
bash
Ensure you add a test script in your package.json
:
json
Basic Jest Configuration
Create a jest.config.js
file in the root of your project for Jest configuration:
javascript
Test Structure
We will organize our tests in a __tests__
directory.
GraphQL Query Tests
We will write tests to ensure our queries work correctly.
Books Test (__tests__/books.test.js
):
javascript
GraphQL Mutation Tests
Let's add tests to create, update, and delete books using GraphQL mutations.
Books Test (__tests__/books.test.js
) (continued):
javascript
Authentication and Authorization Tests
We must also test authentication and authorization scenarios to ensure only authorized users can perform certain actions.
Auth Test (__tests__/auth.test.js
):
javascript
Summary
In this chapter, we have learned to:
- Configure Jest for testing our GraphQL API.
- Test queries and mutations.
- Test authentication and authorization in resolvers.
- Simulate interactions and verify responses using Supertest.
Testing GraphQL ensures that your API behaves correctly under different scenarios, providing a solid foundation for future improvements and maintenance.
[Placeholder: Flowchart diagram showing the testing process from simulating requests to verifying responses in Jest]
In the next chapter, we'll explore optimization strategies and best practices in GraphQL.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
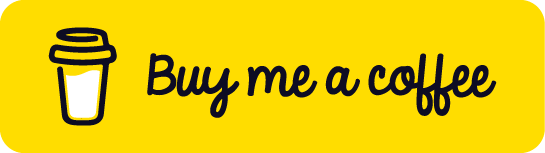
Chat with Chuck
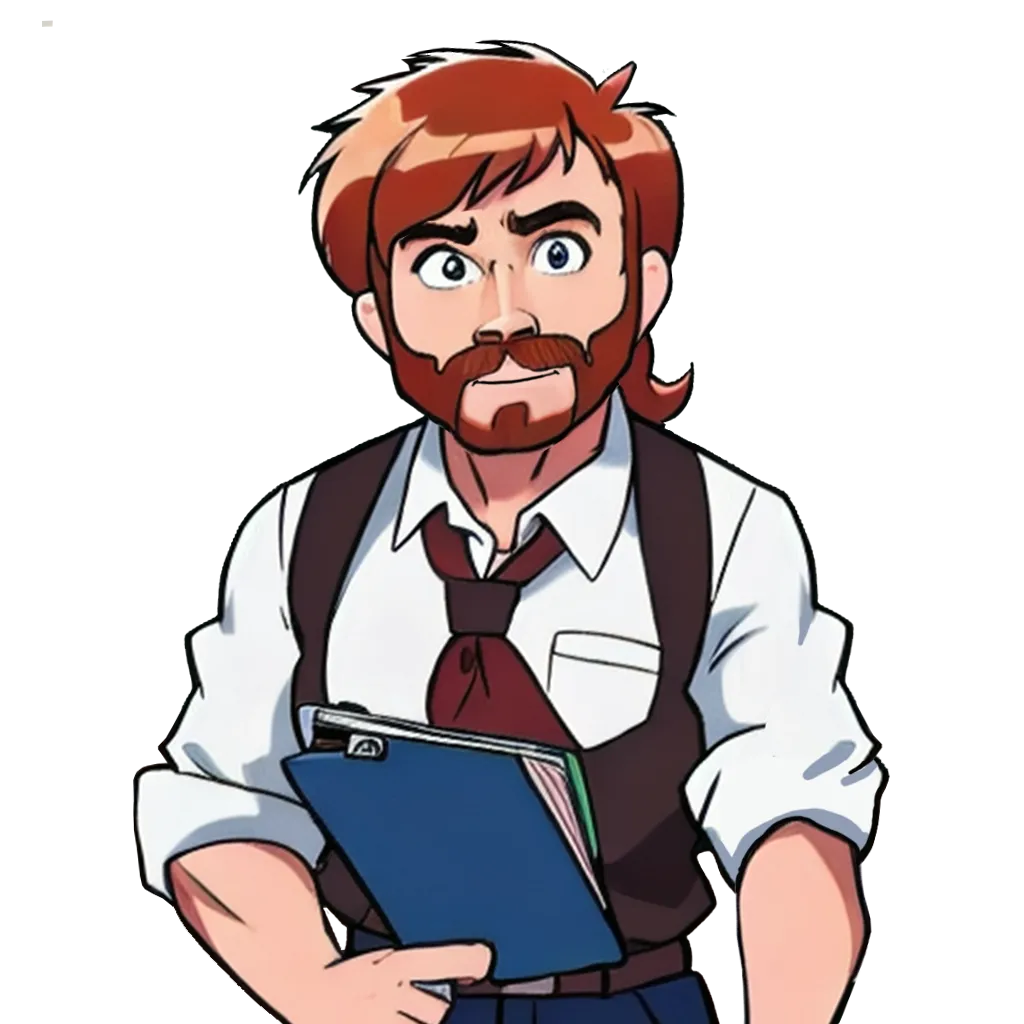
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps