GraphQL with Node
Using Resolvers in GraphQL
Resolvers are the key component in GraphQL that allow your API to handle any query or mutation made by the client. They are functions that fetch or modify data from various sources and return the results to the client. In this chapter, we will deeply explore how resolvers work and how they can be used effectively.
What is a Resolver?
A resolver is a function that receives four basic arguments:
- parent: The object that contains the result of the parent field's resolver.
- args: An object that contains the arguments passed in the query or mutation.
- context: An object that is shared across all resolvers that are executing for a particular operation.
- info: Information about the execution, including the schema and the current operation.
Basic Resolver Example
Using our previous schema as a reference:
graphql
Implementing Resolvers in Node.js
We will implement resolvers for the books
and book
queries.
Mock Data
First, define some mock data:
javascript
Implementing the Resolvers
javascript
Nested Resolvers
Often, you will need to nest resolvers to handle relationships between different types. In our example, a book has an associated author.
The resolver for the author
field in the Book
type looks like this:
javascript
Using the Context (context
)
The context is an object that can contain useful global information for the resolvers, such as user authentication or the database connection.
Example of Context with Authentication
Suppose you have a function to get the authenticated user's information:
javascript
Set up the server to include the user in the context:
javascript
In the resolvers, you can access the user from the context:
javascript
Using info
The info
argument provides information about the current query, schema, and more. Generally, you won't need this argument in basic resolvers, but it is useful for advanced cases.
Example of using info
:
javascript
Complete Example Integrating Context and Resolvers
javascript
Summary
In this chapter, we have deeply explored the use of resolvers in GraphQL, including:
- The definition and basic implementation of resolvers.
- How to handle relationships between types using nested resolvers.
- The use of context to pass global information to the resolvers.
- The use of the
info
argument to get information about the current query.
With this knowledge, you can create efficient and secure resolvers to handle queries and mutations in your GraphQL API. In the next chapter, we will explore database integration.
[Placeholder: Diagram showing how resolvers interact with schema, data, and context]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
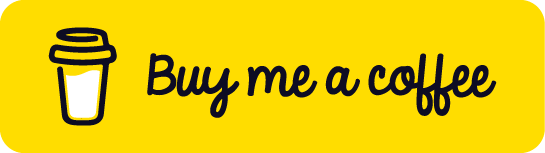
Chat with Chuck
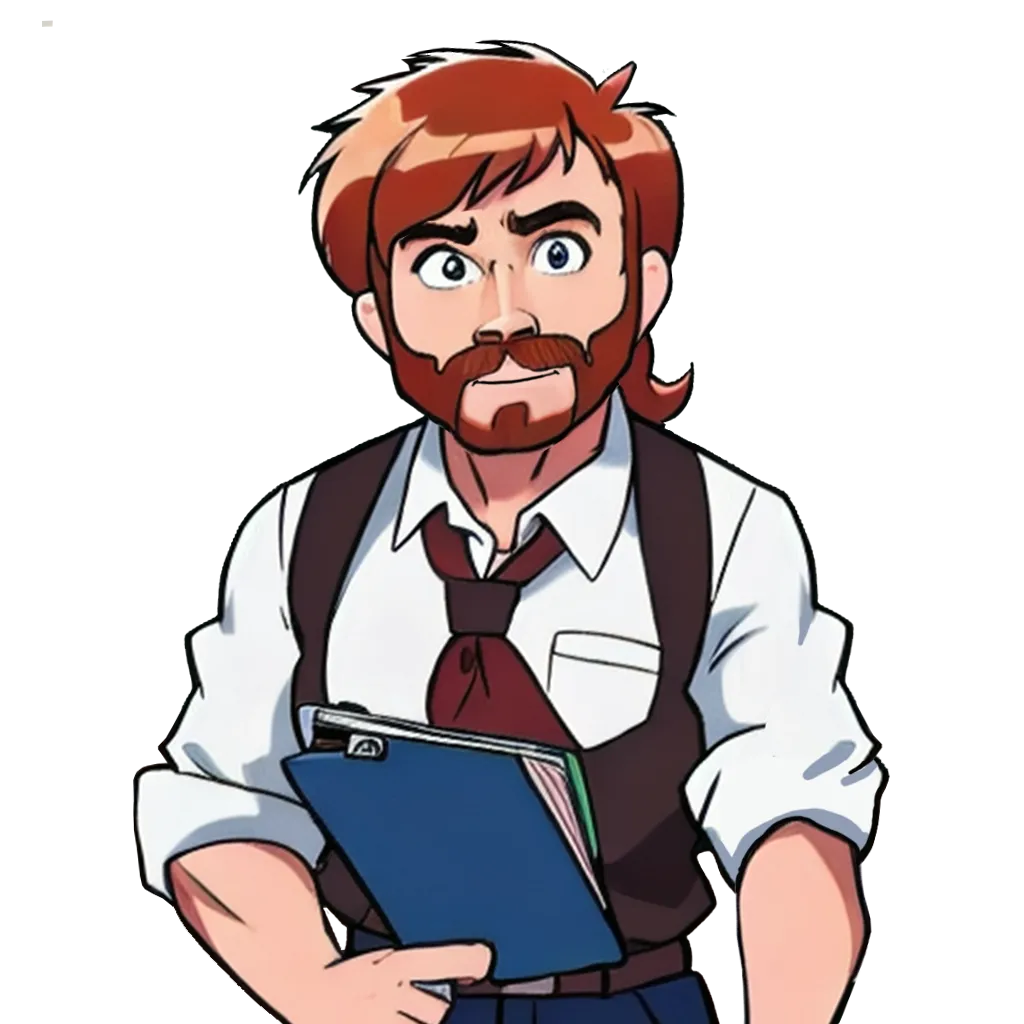
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps