GraphQL with Node
Handling Errors in GraphQL
In any API, proper error handling is crucial to providing a robust and reliable user experience. In GraphQL, errors can occur in various parts, from resolvers to database operations. In this chapter, we will learn how to effectively handle errors and provide useful error messages to clients.
Common Types of Errors in GraphQL
- Validation Errors: These occur when the provided parameters are invalid or missing.
- Authentication Errors: These happen when an unauthenticated user tries to access protected resources.
- Authorization Errors: These happen when an authenticated user tries to perform an action they do not have permissions for.
- Server Errors: Unexpected errors that occur on the server, such as database issues or internal logic errors.
Handling Errors in Resolvers
We are going to update our resolvers to properly handle these types of errors.
Validation Errors
Let's validate the arguments provided by the client, especially in mutations.
Example in resolvers.js
javascript
Authentication and Authorization Errors
Let's ensure that authenticated and authorized users can perform certain operations.
Example in resolvers.js
javascript
Centralized Error Handling
We can also centralize error handling using middleware.
Middleware Example for Error Handling
javascript
Customizing Error Messages
We can customize error messages and add specific error codes for better client understanding.
Example with Custom Error Codes
javascript
With the custom error code, the client can handle errors in a more granular and specific manner.
Summary
In this chapter, we have learned:
- The common types of errors in a GraphQL API.
- How to handle errors in GraphQL resolvers.
- Implement middleware for centralized error handling.
- Customize error messages for better client understanding.
Proper error handling not only improves the stability and security of your API but also provides a better development and user experience.
[Placeholder: Diagram showing how errors are handled and customized at each layer of the GraphQL stack]
In the next chapter, we will explore how to test GraphQL APIs to ensure they work as expected.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
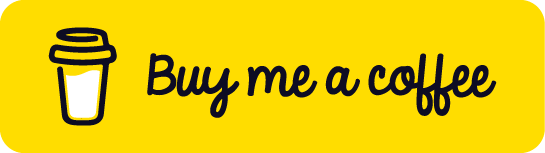
Chat with Chuck
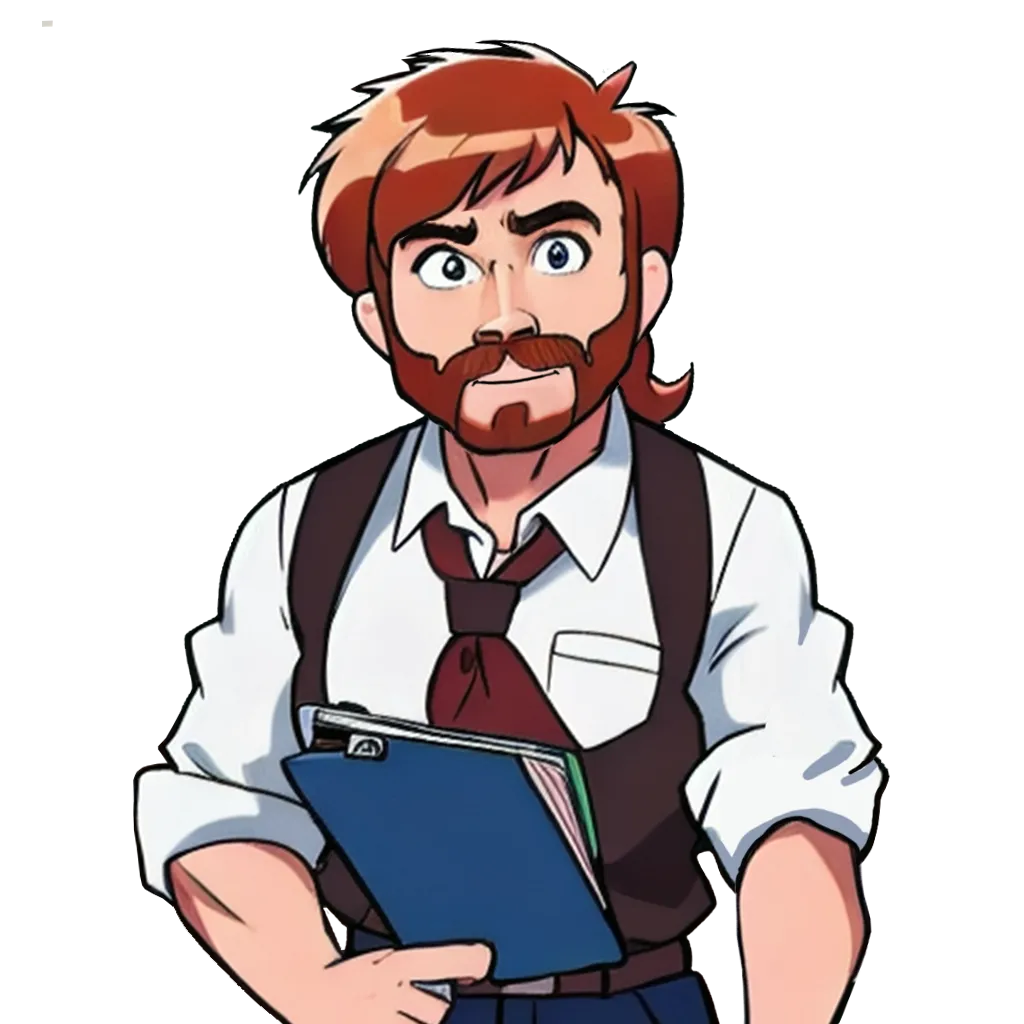
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps