GraphQL with Node
Definition of Schemas and Types in GraphQL
In GraphQL, schemas and types form the backbone of any API. Correctly defining the schema and types is crucial for establishing how clients can interact with your data. In this chapter, we will delve into how to order and structure your schema and the various types that GraphQL offers.
The Schema
The schema in GraphQL defines the structure of your data and the operations that can be performed on it. A GraphQL schema consists of the following main elements:
Root Operations
- Query: Represents reading and retrieving data operations.
- Mutation: Represents writing operations, such as creating, updating, or deleting data.
- Subscription: Allows subscribing to events that occur in real-time.
Example of a basic schema:
graphql
Definition of Types
GraphQL is a strongly typed language. Here we explore some of the most important types you can use:
Scalar Types
Scalar types are primitive data types that do not split into subfields. The main scalar types are:
- Int: Integers.
- Float: Floating-point numbers.
- String: Text strings.
- Boolean: Boolean values (
true
orfalse
). - ID: Unique identifier, typically used to uniquely identify a resource.
Object Types
Object types allow describing a more complex data structure. An object type contains a set of fields, each with its own type.
Example of an object type:
graphql
Lists and Non-Nulls
GraphQL allows specifying that a field is a list of values or that it cannot be null (null
).
- Lists: Use square brackets
[]
. For example,[User]
means a list of users. - Non-Nulls: Use the exclamation mark
!
. For example,String!
means that the field cannot be null.
Example combining lists and non-nulls:
graphql
Complete Schema Example
Let’s define a more detailed schema for a library API:
graphql
In this example, we have two object types (Book
and Author
) and several queries (books
, book
, authors
, author
). There is also a mutation to create a new book.
Resolvers for the Schema
Next, we define the corresponding resolvers for this schema in resolvers.js
:
javascript
Server Configuration
Ensure that your server.js
is correctly configured to handle these new types and resolvers:
javascript
Summary
In this chapter, we have explored how to define schemas and types in GraphQL. We defined queries and mutations, and created resolvers that correspond to those types. With this knowledge, you are ready to write more sophisticated queries and mutations, which we will cover in the following chapters.
[Placeholder: Diagram illustrating how a GraphQL schema defines data types and their relationships]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
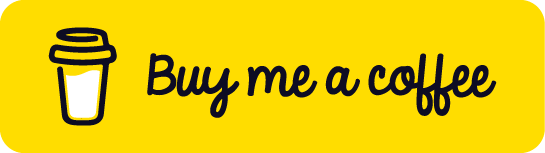
Chat with Chuck
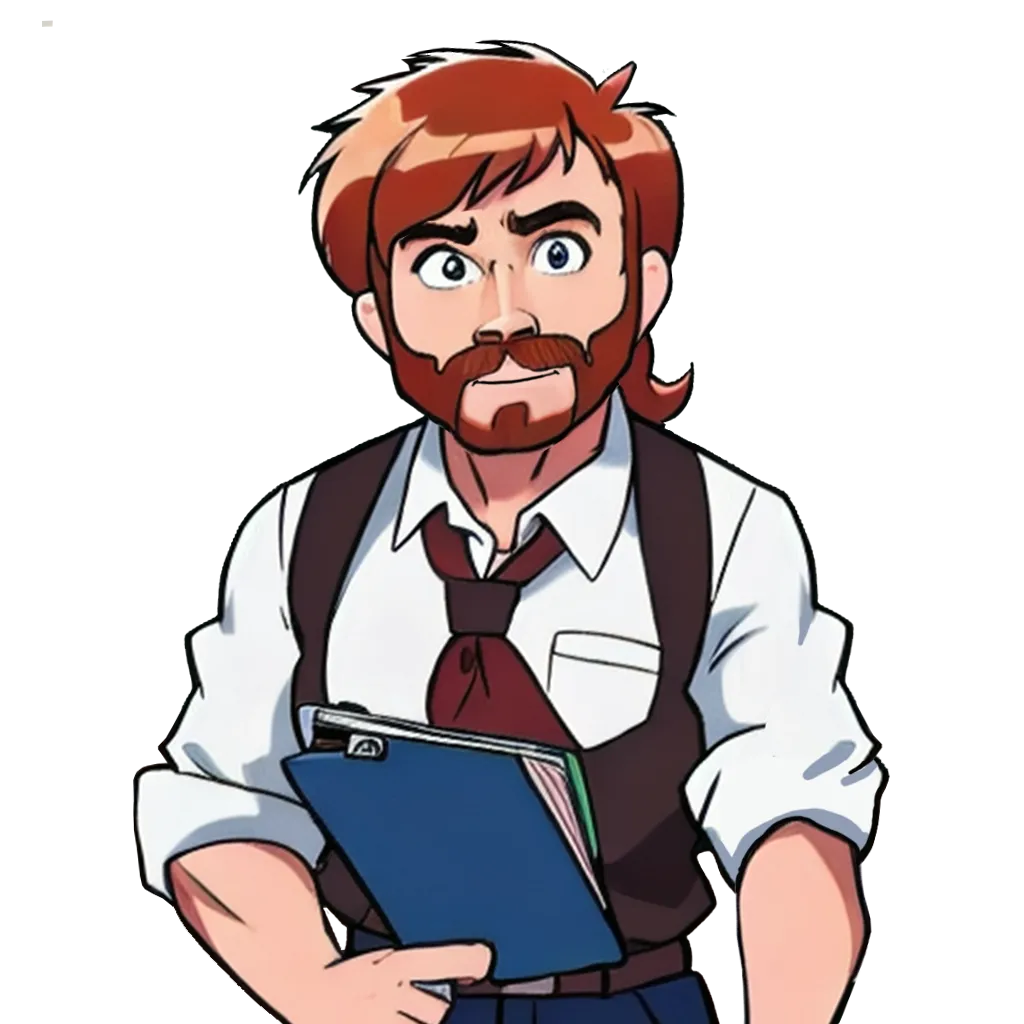
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps