GraphQL with Node
Development Environment Setup
To start working with GraphQL and Node.js, we first need to set up our development environment. In this chapter, we will guide you through the necessary steps to install Node.js, configure a new project, and add the basic dependencies needed to start building our GraphQL API.
Installing Node.js
If you don't have Node.js installed on your machine yet, you can download it from nodejs.org. It is recommended to install the LTS (Long Term Support) version for greater stability.
You can verify the installation by running the following commands in your terminal:
These commands should display the installed versions of Node.js and npm (Node Package Manager).
Creating a New Project
Once Node.js and npm are installed, you can create a new project. Open your terminal and run the following commands:
bash
These commands will create a new directory called my-graphql-project
and generate a package.json
file with the default settings.
Installing Dependencies
To set up a GraphQL server with Node.js, we will need the following dependencies:
- express: A minimalist web framework for Node.js servers.
- express-graphql: A GraphQL integration for Express.
- graphql: The reference implementation of GraphQL.
Install the dependencies by running the following command:
bash
Creating the Basic Project Structure
Create the following files in your project:
Server Configuration in server.js
First, let's set up a basic server in server.js
:
javascript
Schema Definition in schema.js
At this stage, we will create a basic schema. In later chapters, we will delve into defining types and resolvers.
javascript
This basic setup defines a single query called hello
that returns a string.
Running the Server
To run the server, use the following command:
bash
You should now see a message like "GraphQL Server running at http://localhost:4000/graphql". You can open this URL in your browser to access GraphiQL, an integrated development environment for working with GraphQL.
[Placeholder: Screenshot showing GraphiQL open at http://localhost:4000/graphql, with a "hello" query and its corresponding response]
By writing and executing the query
graphql
You should get the following response:
json
Summary
In this chapter, we set up our development environment, creating a project with Node.js, Express, and GraphQL. Now we are ready to delve into the basics of GraphQL in the next chapter.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
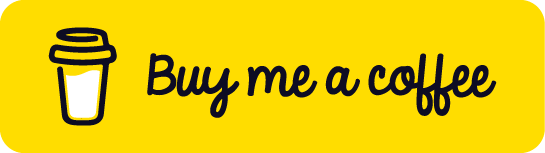
Chat with Chuck
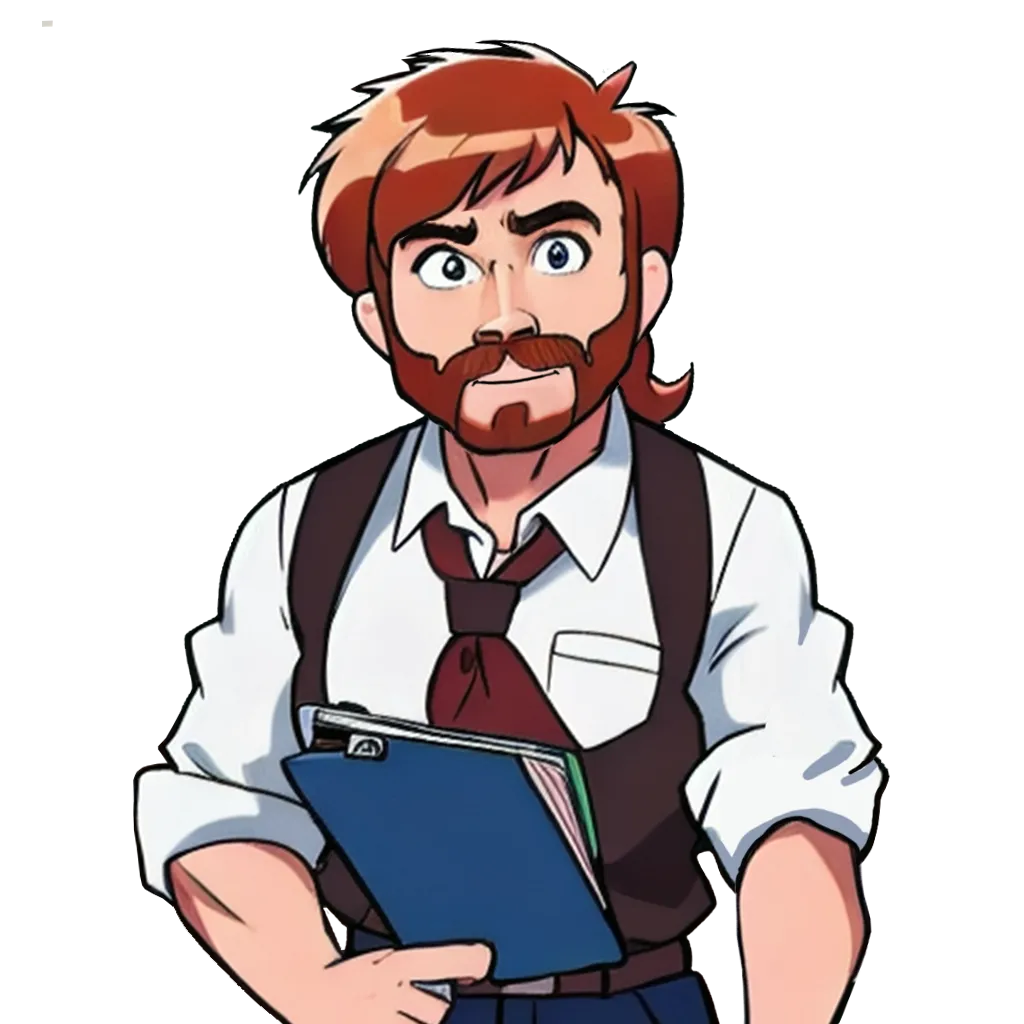
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps