GraphQL with Node
Pagination and Filtering in GraphQL
Pagination and filtering are essential techniques for handling large datasets in an API. In GraphQL, these techniques allow clients to request exactly the data they need efficiently. In this chapter, we will learn how to implement pagination and filtering in our GraphQL API.
Pagination
Pagination divides large datasets into manageable parts, making data transfer and manipulation easier. In GraphQL, there are several ways to implement pagination. In this example, we will use limit-offset based pagination.
Defining Schema for Pagination
We will add limit
and offset
arguments to our book query.
Schema (schema.js
):
graphql
Implementing Resolvers for Pagination
We will update the corresponding resolver to handle limit
and offset
.
Resolvers (resolvers.js
):
javascript
Pagination Example in GraphiQL
graphql
Filtering
Filtering allows clients to obtain a specific subset of data based on certain criteria.
Defining Schema for Filtering
We will add authorId
and publishedYear
arguments to our book query to allow filtering.
Schema (schema.js
):
graphql
Implementing Resolvers for Filtering
We will update the books
resolver to handle the filters.
Resolvers (resolvers.js
):
javascript
Filtering Example in GraphiQL
- Filtering by author:
graphql
- Filtering by published year:
graphql
Combining Pagination and Filtering
You can combine pagination and filtering to obtain finer control over the requested data.
Combined Example in GraphiQL
graphql
Summary
In this chapter, we learned how to implement pagination and filtering in a GraphQL API. We covered:
- Definition of
limit
andoffset
arguments for pagination. - Implementation of filters using additional arguments.
- Combining pagination and filtering for even more precise queries.
These techniques will allow you to efficiently handle large datasets and provide clients the ability to request exact data based on their needs.
[Placeholder: Diagram showing the flow of a GraphQL query with pagination and filtering, from client request to the server response]
In the next chapter, we will explore how to handle errors in GraphQL effectively.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
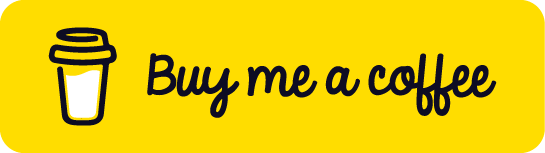
Chat with Chuck
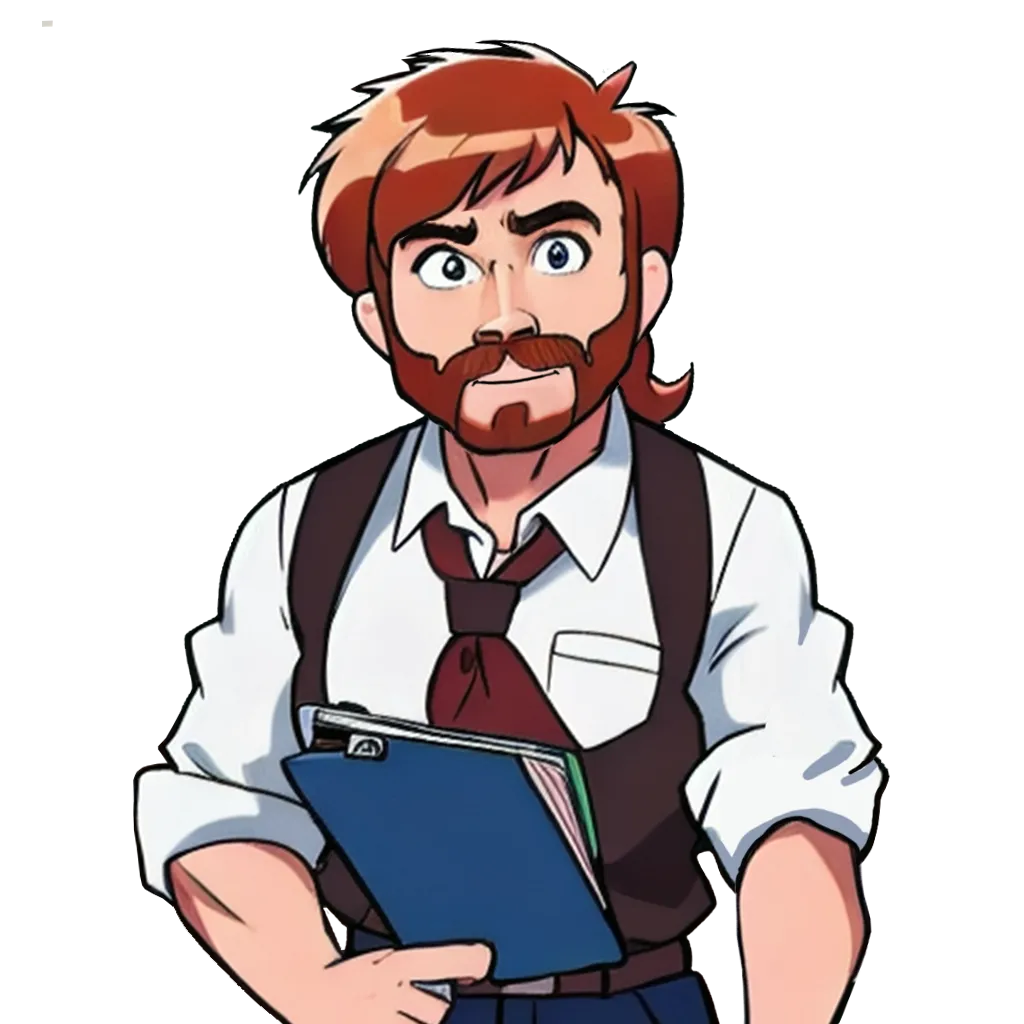
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps