GraphQL with Node
Basic Concepts of GraphQL
In this chapter, we will delve into the fundamental concepts of GraphQL. Understanding these concepts is crucial for building efficient and flexible APIs. The topics we will cover include schema, types, queries, mutations, and resolvers.
Schema
The schema is the central piece of any GraphQL API. It is a description of the types of data that can be queried and manipulated in your API. It defines what queries and mutations are available and how they relate to each other.
Example of a basic schema:
graphql
Types
In GraphQL, everything is strongly typed. Types define the structure of the data you can query. The main types include:
- Scalar Types: Represent unique values like
Int
,Float
,String
,Boolean
, andID
. - Objects: Groupings of fields that can be other scalar types or objects.
- Lists: Sequences of types.
Example of type definitions:
graphql
Queries
Queries are requests for data. They are equivalent to GET methods in a REST API. A query specifies what data you want to retrieve according to the defined schema.
Example of a query in GraphQL:
graphql
Mutations
Mutations are operations that allow you to create, update, or delete data. They are equivalent to POST, PUT, DELETE methods in a REST API.
Example of a mutation:
graphql
Resolvers
Resolvers are the functions that execute to get the requested data in queries and mutations. They are responsible for fetching the data either from a database, an external service, or any other data source.
Example of a resolver in Node.js:
javascript
Putting it all together
To integrate these concepts into a single example, we will update our schema.js
and server.js
files:
schema.js
javascript
server.js
javascript
Summary
In this chapter, we covered the basic concepts of GraphQL, including schema, types, queries, mutations, and resolvers. With this foundation, we are ready to move on to setting up a GraphQL server with Node.js in the next chapter.
[Placeholder: Diagram illustrating the relationship between schema, types, queries, and mutations in GraphQL]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
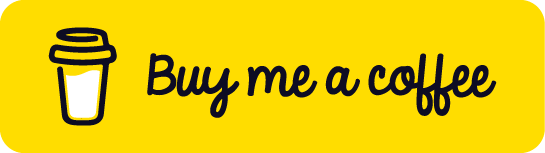
Chat with Chuck
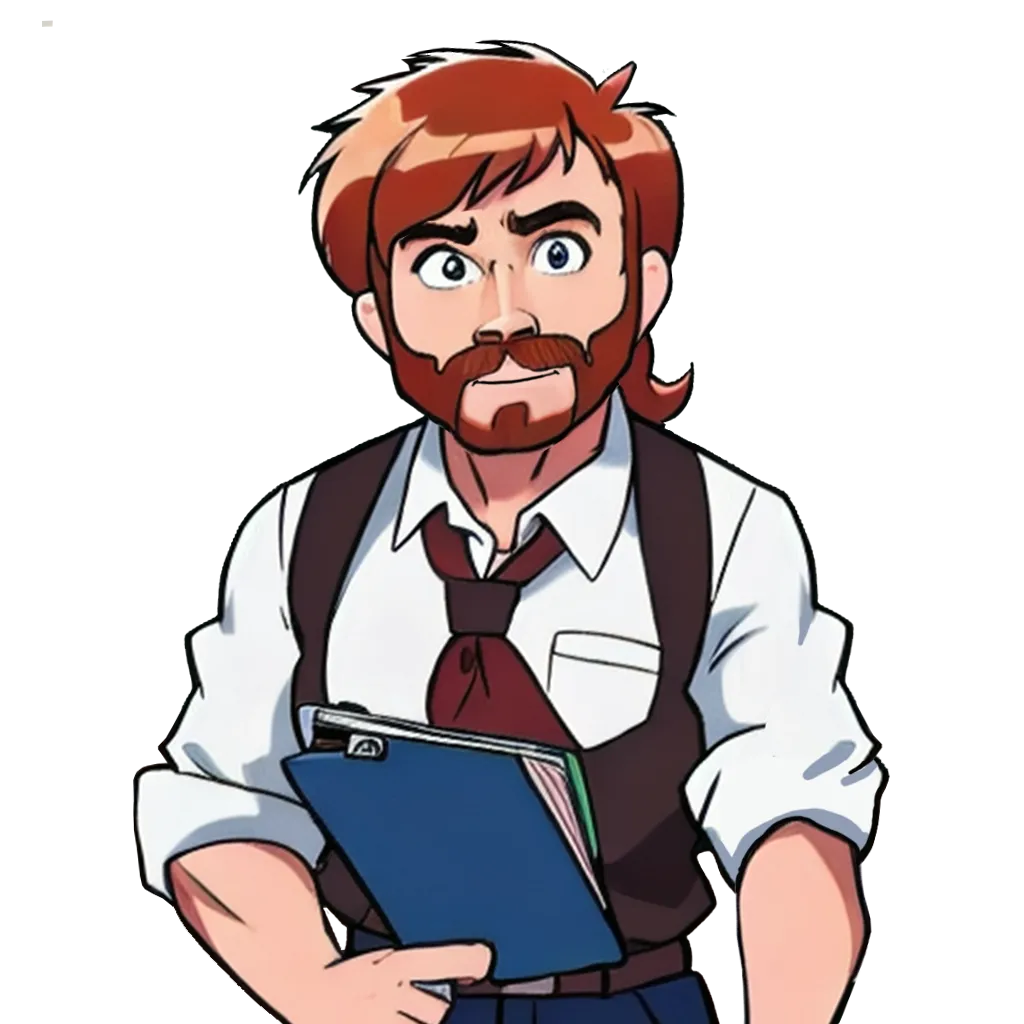
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps