GraphQL with Node
Creating Queries in GraphQL
Queries in GraphQL are one of the most powerful features of the language. Queries allow clients to request exactly the data they need, avoiding under-fetching and over-fetching commonly associated with traditional REST APIs. In this chapter, we will learn how to define and use queries in GraphQL.
Defining Queries in the Schema
In GraphQL, queries are defined as part of the Query
type in the schema. Fields of the Query
type represent the operations that clients can perform to read data.
Schema Example with Queries
Based on our library API example:
graphql
Implementing Resolvers for Queries
Each query defined in the schema must have a corresponding resolver. A resolver is a function that returns the data requested by the query.
Resolver Implementation Example
In our resolvers.js
file, we would implement the resolvers for the queries as follows:
javascript
Making Queries from the Client
Once the schema and resolvers are defined, clients can make queries to retrieve data. GraphiQL, a user interface for interacting with GraphQL APIs, makes it easy to write and execute queries.
GraphiQL Query Examples
- Query to get all books:
graphql
- Query to get a book by ID:
graphql
- Query to get all authors:
graphql
Pagination and Filtering
For more complex APIs, queries often need to support pagination and filtering. Although GraphQL does not enforce a specific way to implement pagination and filtering, here is a basic example using arguments in queries:
Schema Example with Pagination and Filtering
graphql
Resolver Implementation for Pagination and Filtering
javascript
Making Queries with Pagination and Filtering
graphql
Summary
In this chapter, we learned how to:
- Define queries in the GraphQL schema.
- Implement resolvers to handle queries.
- Make queries from the client using GraphiQL.
- Implement pagination and filtering in queries.
These skills allow us to structure queries efficiently, providing clients exactly the data they need. In the next chapter, we will explore creating mutations in GraphQL.
[Placeholder: Diagram showing how query resolvers connect to the schema and respond to client requests]
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps
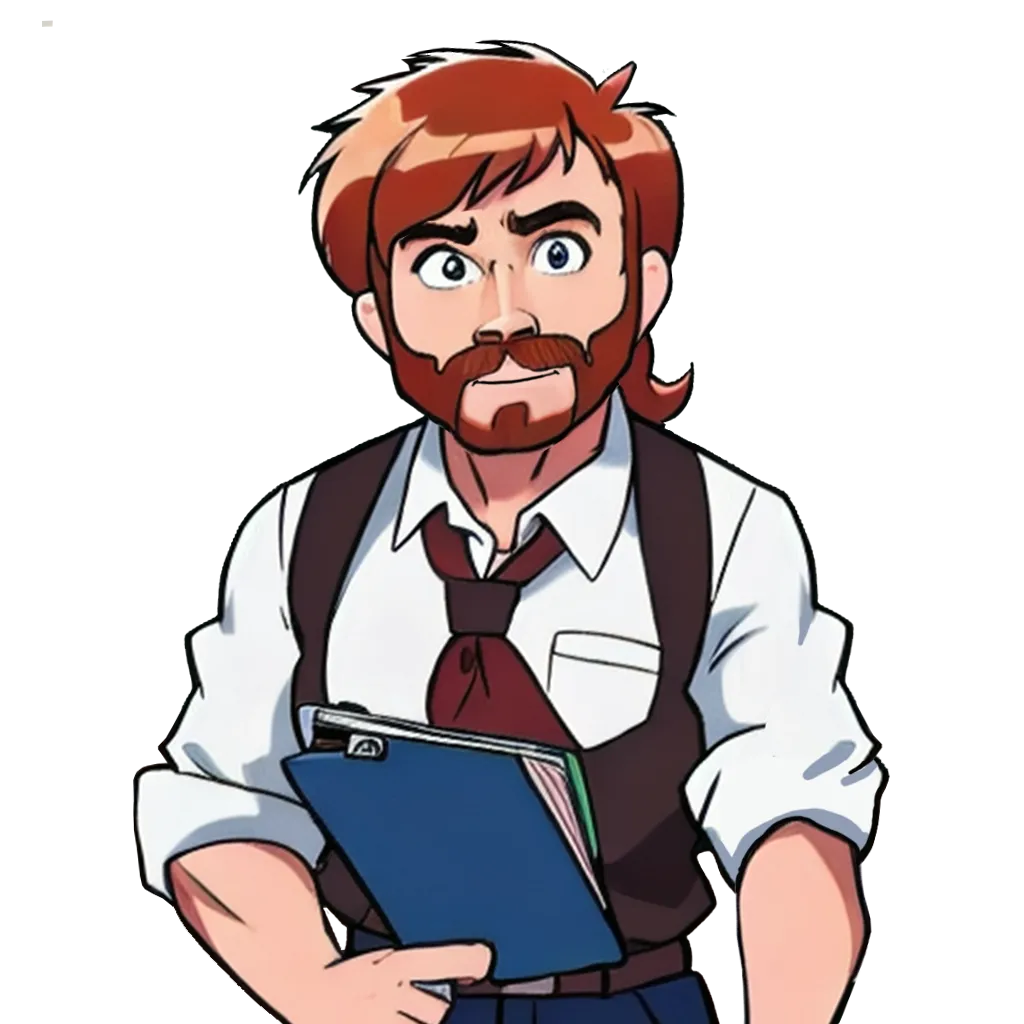