GraphQL with Node
Setting Up a GraphQL Server with Node.js
In this chapter, you will learn how to set up a GraphQL server using Node.js and Express. We have already covered some basic concepts and carried out an initial setup in previous chapters, but now we will delve deeper into how to build a complete and functional server.
Installing Essential Dependencies
Make sure you have the following dependencies installed, which are necessary to set up a GraphQL server:
bash
Creating a Project from Scratch
If you haven't created a project before, follow these steps to set up a basic environment:
bash
Installing Babel
To write modern JavaScript code, we will install Babel:
bash
Next, create a .babelrc
file in the root of your project with the following configuration:
json
Now you can use Babel to run your server from the command line:
bash
Basic Server Setup
Create the main directories and files:
Defining the Schema in schema.js
We are going to create a basic schema that includes queries and mutations to handle users:
javascript
Defining the Resolvers in resolvers.js
The resolvers will be the functions that handle the logic for the queries and mutations defined in the schema:
javascript
Server Configuration in server.js
Set up the server to use GraphQL and the resolvers:
javascript
Running the Server
To run the server with Babel, use the following command:
bash
You should see a message like "GraphQL server running at http://localhost:4000/graphql". You can open this URL in your browser to access GraphiQL.
[Placeholder: Screenshot showing GraphiQL open at http://localhost:4000/graphql, with example queries and mutations]
Functionality Test
Test some queries and mutations from GraphiQL to verify that everything is working correctly.
Queries
graphql
graphql
Mutations
graphql
graphql
graphql
Summary
In this chapter, we set up a complete GraphQL server using Node.js and Express, defined a basic schema, implemented resolvers, and verified their functionality. With this basic server, we are ready to explore more in-depth schema and type definitions in GraphQL in the next chapter.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
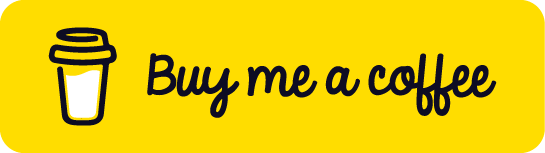
Chat with Chuck
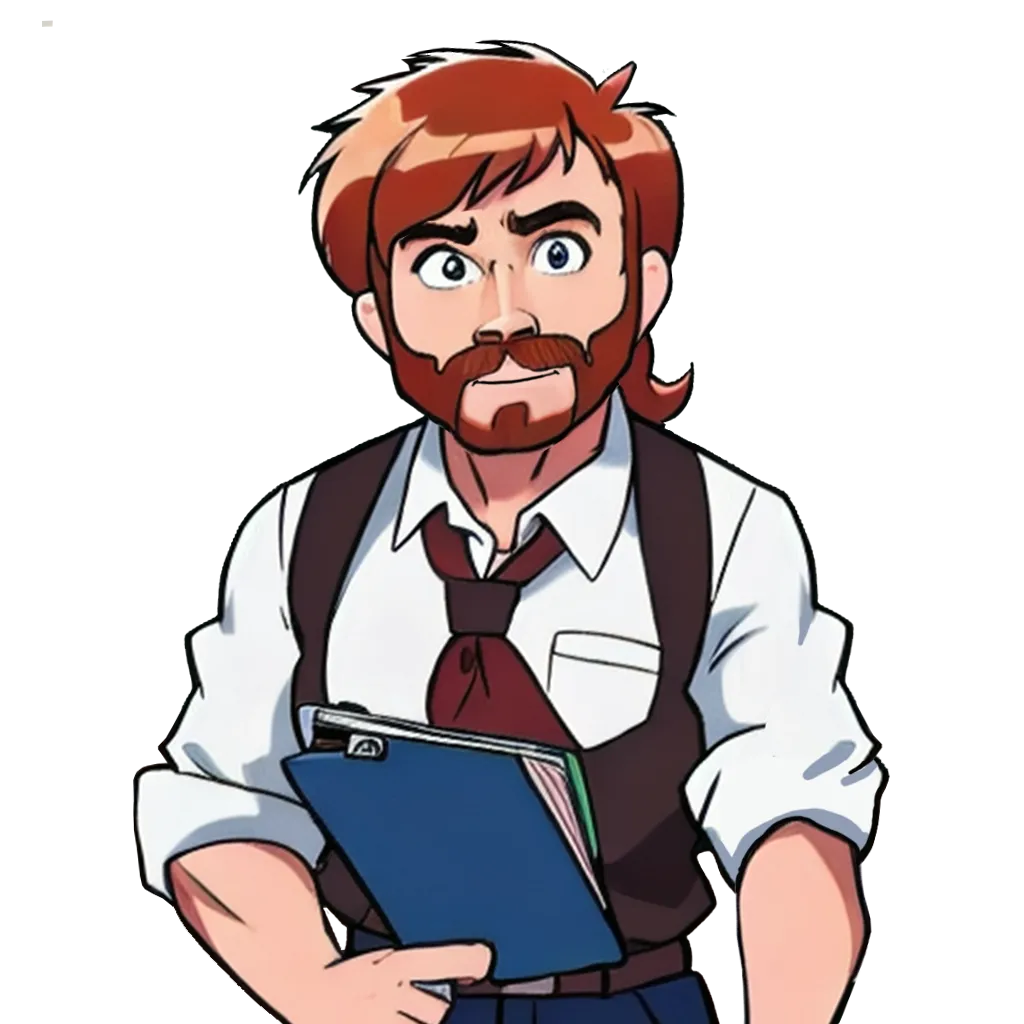
- Introduction to GraphQL
- Development Environment Setup
- Basic Concepts of GraphQL
- Setting Up a GraphQL Server with Node.js
- Definition of Schemas and Types in GraphQL
- Creating Queries in GraphQL
- Creating Mutations in GraphQL
- Using Resolvers in GraphQL
- Integration with Databases
- Authentication and Authorization in GraphQL
- Pagination and Filtering in GraphQL
- Handling Errors in GraphQL
- Testing GraphQL APIs
- Optimization and Best Practices
- Conclusion and Next Steps