State Management in React
Best Practices in State Management
Proper state management is crucial to keep a React application predictable, efficient, and easy to maintain. By following best practices, you can avoid common issues and make development easier as your application grows. In this section, we will explore some best practices to consider when managing state in React and Redux.
Splitting Local and Global State
Not all state needs to be global. Dividing state between local and global can lead to a simpler and more manageable application.
Local State:
- Use it for data specific to a component.
- It can be easily managed with hooks like
useState
anduseReducer
.
Global State:
- Use it for data that needs to be accessible across multiple components.
- Ideal to handle with Context API or Redux.
State Structure
Maintaining a clear and flat structure for states can ease their management and updating.
Avoid Deep Nesting:
jsx
Immutability
Always treat state as immutable. Not mutating the state directly ensures changes are predictable and debugging is easier.
Example with useState
:
jsx
Example with Redux:
jsx
Splitting Reducers
Splitting reducers into smaller, specific functions can lead to more modular and maintainable code. Use combineReducers
to combine them into a root reducer.
jsx
Using Selectors
Selectors are functions that extract and transform state from the Redux store. Using selectors can improve encapsulation and code reusability.
Selector Example:
jsx
Usage in Component:
jsx
Error Handling
Implementing proper error handling when working with asynchronous operations and global states can enhance user experience and application robustness.
Async Action with Error Handling (Thunk) Example:
jsx
Error Handling in Components Example:
jsx
Documenting State and Actions
Maintain clear documentation of the state and actions handled by your application. This helps other developers (or yourself in the future) better understand interactions within the code.
Action Documentation Example:
jsx
Explanatory Image
Conclusion
Applying best practices in state management will help you keep your React application more organized, efficient, and maintainable. These practices not only improve code quality but also facilitate collaboration and application scalability. In the next section, we will conclude with a recap of the material covered and explore Best Resources for the Future.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
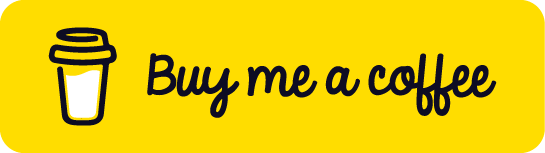
Chat with Chuck
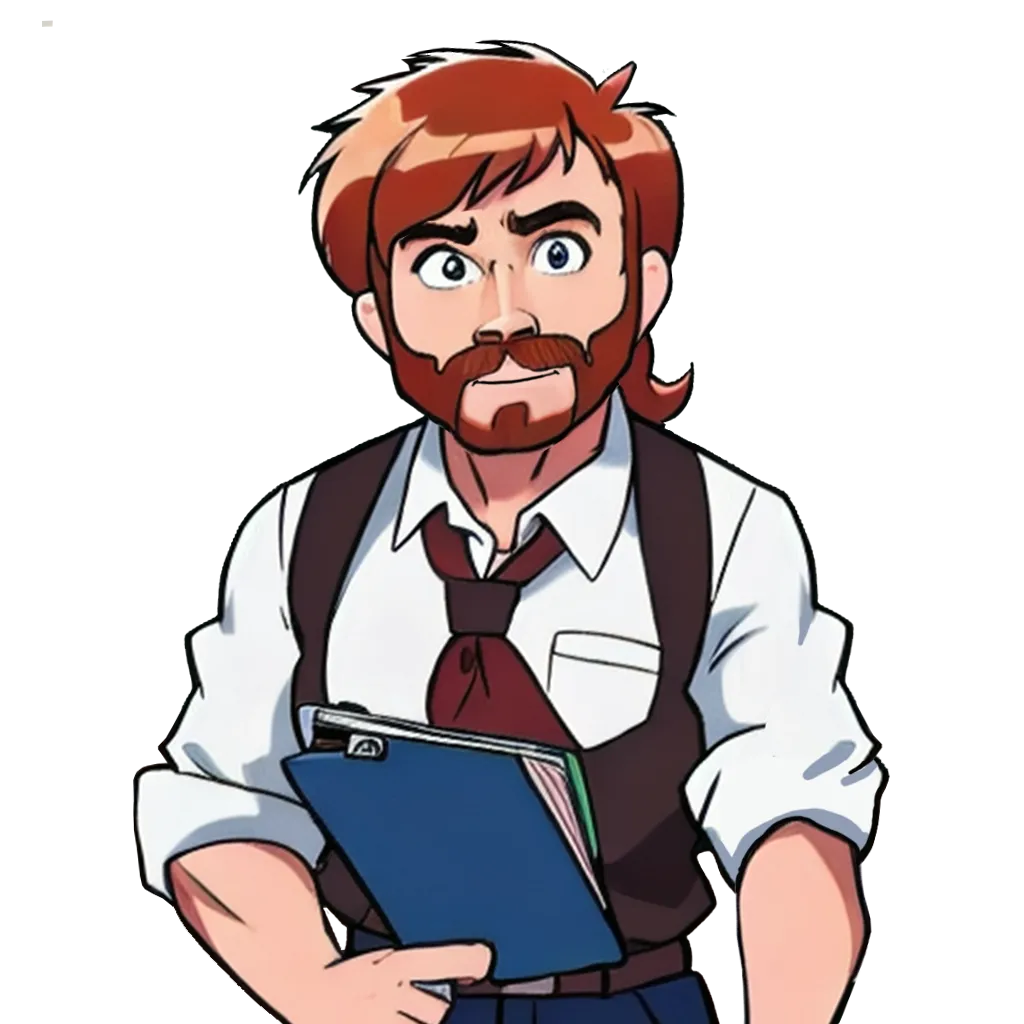
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future