State Management in React
Integrating Redux with React
Integrating Redux with React allows you to efficiently manage global state in React applications, providing a clear structure for data flow and ease of debugging. In this section, we will learn how to connect Redux with React and how to use react-redux
to handle state in your React components.
Installing React-Redux
If you haven't already, install react-redux
in your project:
sh
Store Provider
To connect Redux to your React application, wrap your application with the Provider
component from react-redux
. The Provider
makes the Redux store available to the entire application.
Example:
jsx
Connecting Components with useSelector
and useDispatch
react-redux
provides two main hooks to connect components to Redux: useSelector
and useDispatch
.
useSelector
: Allows extracting data from the store.useDispatch
: Allows sending actions to the store.
Basic Example:
jsx
In this example:
useSelector
selects thecount
property from the global state.useDispatch
returns thedispatch
function to send actions.
Complete Example: To-Do List
Let's create a To-Do List application to fully illustrate how to integrate Redux with React.
Actions:
jsx
Reducers:
jsx
Store:
jsx
Components:
jsx
jsx
Benefits of Integrating Redux with React
- Centralized State: Simplifies managing and synchronizing global state across the application.
- Predictability: Each state change is handled through predefined actions and reducers, making the data flow predictable.
- Scalability: Redux's structure facilitates the scalability of large applications by keeping state logic and updates in one place.
Considerations and Best Practices
- Action and State Management: Keep actions and state clear and descriptive.
- Using Selectors: Use
useSelector
to select only the necessary parts of the state to avoid unnecessary re-renders. - Reducer Splitting: Split reducers into smaller, specific functions to improve maintainability.
Explanation Image
[Placeholder: "Diagram illustrating how react-redux
connects React components with the Redux store, including the use of Provider
, useSelector
and useDispatch
."]
Integrating Redux with React provides a clear and efficient structure for handling complex states in React applications. In the next section, we will explore Tools and Middleware for Redux, which extend Redux's capabilities and make developing and debugging applications easier.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
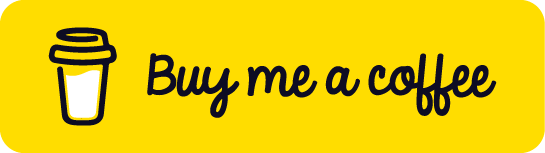
Chat with Chuck
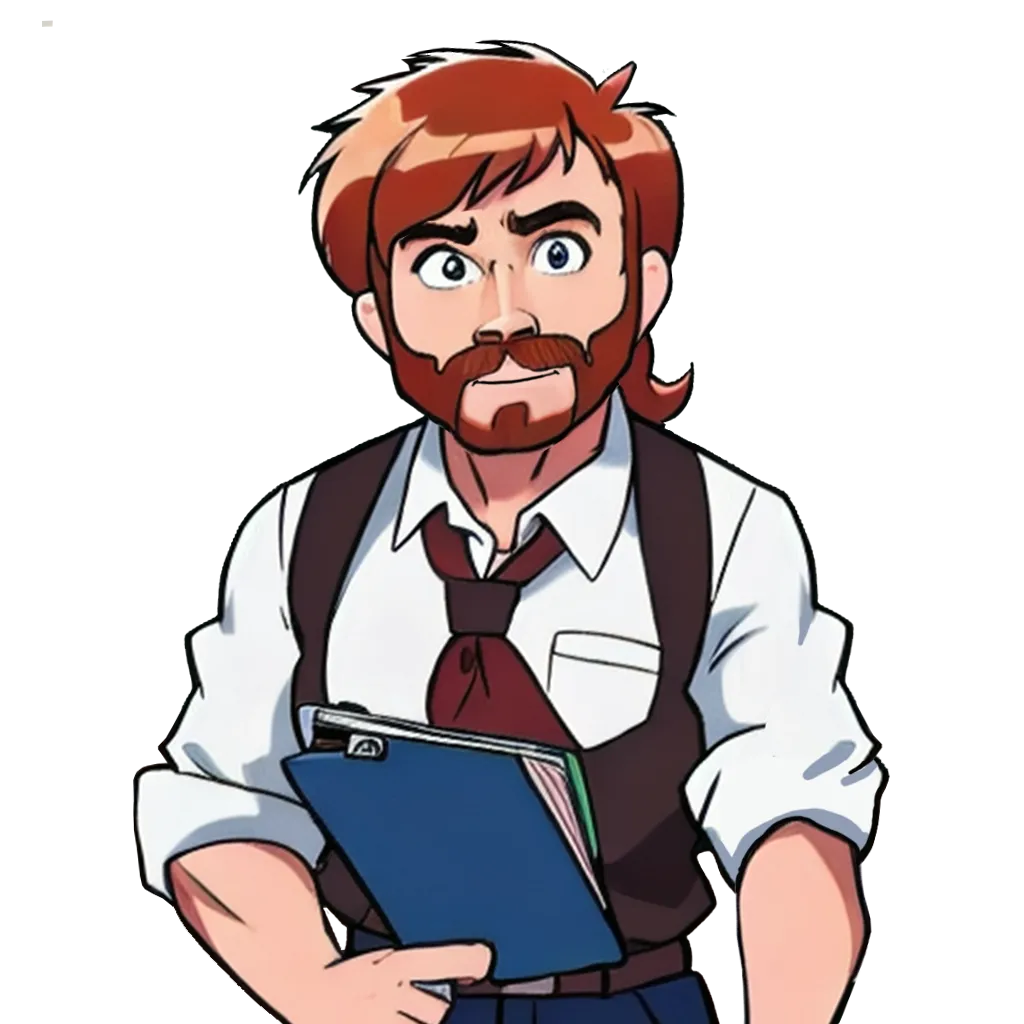
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future