State Management in React
Conclusions and Best Resources for the Future
Throughout this course, we have deeply explored the essential concepts and techniques for state management in React, both with React's native tools and advanced libraries like Redux. We have covered everything from the fundamentals to best practices and the use of middleware to handle asynchronous operations. In this final section, we will recap the key points and provide additional resources to continue your learning.
Summary of Contents
1. Introduction to State Management in React
- Importance of state management in interactive applications.
- Difference between local and global state.
2. Fundamentals of State in React
- Concept and properties of state.
- Basic examples in class and functional components.
3. Local State vs. Global State
- Definition and proper use of local and global state.
- Examples and design patterns such as state lifting.
4. Using useState and setState
- State management in functional and class components.
- Key differences and practical examples.
5. Event Handling and State Update
- Event handling in React.
- Updating state in response to user events.
6. Passing State Between Components with Props
- Using props to share state between components.
- State lifting pattern.
7. Context API for Global State Management
- Implementation and use of Context API.
- Practical examples for sharing global state.
8. Using Reducers and useReducer
- Concept of reducers and using
useReducer
in React. - Practical examples and design patterns.
9. Managing Asynchronous State with useEffect
- Using
useEffect
to handle asynchronous operations. - Practical examples of data fetching and timer handling.
10. State Management with Redux
- Introduction to Redux and its principles.
- Configuring the store and concepts of actions and reducers.
11. Actions, Reducers, and the Store in Redux
- Structure of actions and reducers in Redux.
- Complete examples and best practices.
12. Integrating Redux with React
- Using
react-redux
to connect Redux with React. - Examples of connected components and global state management.
13. Tools and Middleware for Redux
- Using middleware like
redux-thunk
andredux-saga
. - Debugging tools like Redux DevTools.
14. Best Practices in State Management
- Dividing local and global state.
- Maintaining state immutability and using selectors.
Additional Resources
Continuing learning and staying updated is key to mastering state management in React and Redux. Here are some recommended resources:
Official Documentation
- React Documentation: https://reactjs.org/docs/getting-started.html
- Redux Documentation: https://redux.js.org/introduction/getting-started
- React-Redux Documentation: https://react-redux.js.org/introduction/getting-started
Tutorials and Articles
- FreeCodeCamp Guide to React: https://www.freecodecamp.org/news/the-react-handbook-b71c27b0a795/
- Redux Fundamentals by Redux Team: https://redux.js.org/tutorials/fundamentals/part-1-overview
- Overreacted Blog by Dan Abramov: https://overreacted.io/
Courses and Video Tutorials
- Egghead.io - The Beginner's Guide to React: https://egghead.io/courses/the-beginner-s-guide-to-react
- Udemy - Modern React with Redux: https://www.udemy.com/course/react-redux/
- Frontend Masters - Complete Intro to React: https://frontendmasters.com/courses/complete-intro-react/
Utility Tools
- Redux DevTools Extension: https://github.com/reduxjs/redux-devtools
- React Developer Tools: https://github.com/facebook/react-devtools
Conclusion
Mastering state management in React and Redux may seem challenging initially, but with practice and following best practices, you can build robust and maintainable applications. This course has provided you with a solid foundation, and with the mentioned resources, you can continue to hone your skills and stay updated with the best techniques in modern front-end development.
Congratulations on completing the course and best of luck on your ongoing journey with React and Redux!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
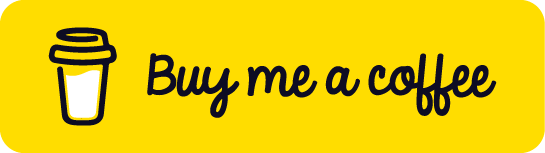
Chat with Chuck
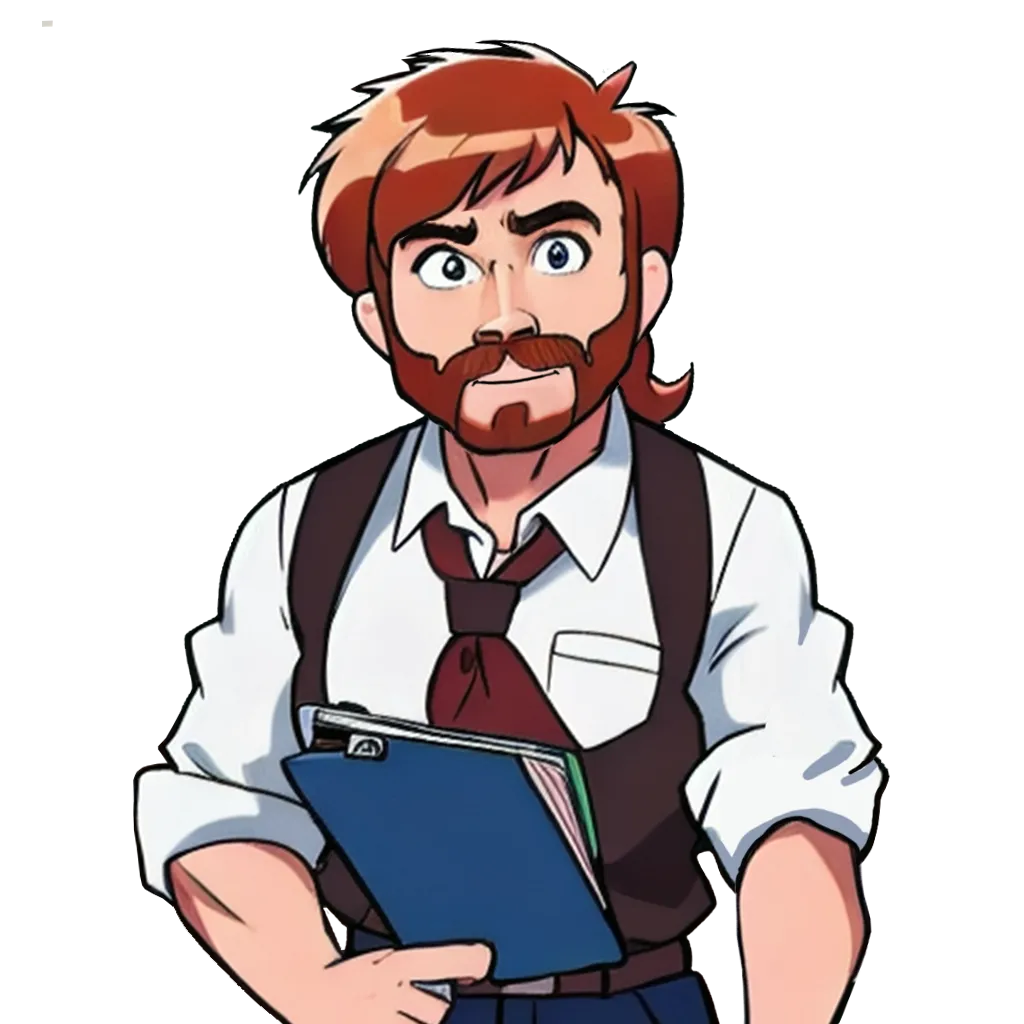
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future