State Management in React
Using useState and setState
To manage state in React, different methods are used depending on whether you are working with functional components or class components. useState
is the hook used to manage state in functional components, while setState
is the traditional method used in class components.
Using useState
in Functional Components
useState
is a hook that allows you to add state to functional components in React. You can declare multiple states by calling useState
multiple times.
Basic Syntax:
jsx
state
: The variable that contains the state value.setState
: The function used to update the state.initialValue
: The initial state value.
Simple Example:
jsx
In this example:
- We initialize
count
to0
usinguseState(0)
. - We update the state by calling
setCount
when the button is clicked.
Using setState
in Class Components
setState
is the method used to update state in class components. Unlike useState
, setState
performs a shallow merge of objects, making partial state updates easier.
Basic Syntax:
jsx
partialState
: An object representing the changes in the state.callback
: (Optional) A function executed after the state has been updated.
Simple Example:
jsx
In this example:
- We initialize
count
to0
in the component constructor. - We update the state by calling
this.setState
with the partial state.
Key Differences
-
Syntax and Usage:
useState
is specific to functional components.setState
is specific to class components.
-
State Management:
useState
is simpler and allows managing multiple localized states.setState
performs a shallow merge, making it easier to update nested objects.
-
State Update:
useState
returns a new copy of the state.setState
can partially update the state.
Practical Example: Controlled Form
Functional Component:
jsx
Class Component:
jsx
Explanatory Image
By understanding the use of useState
and setState
, you can efficiently manage state in your React components, whether you prefer using functional or class components. In the next section, we will explore Event Handling and State Update, which is crucial for creating interactive React applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
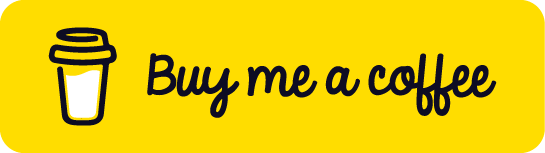
Chat with Chuck
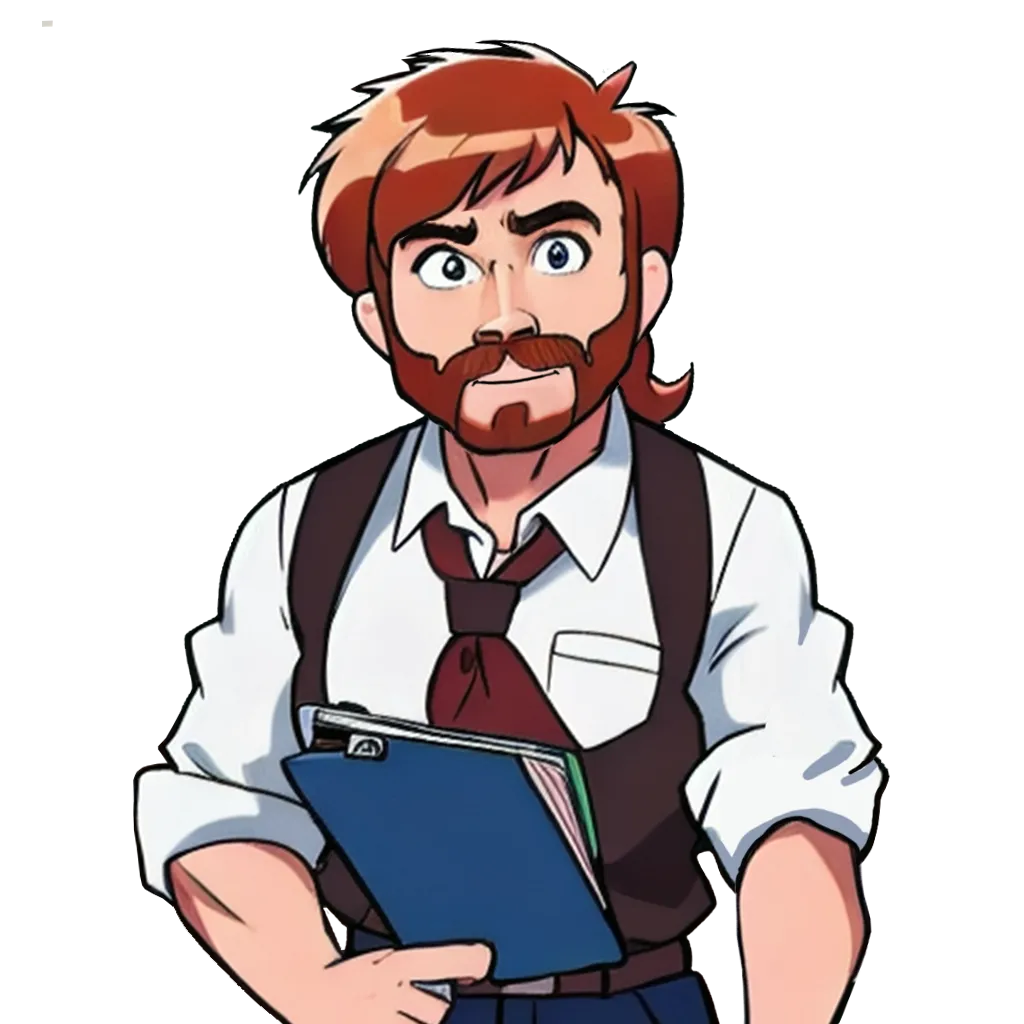
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future