State Management in React
Local State vs. Global State
In React, state management can be done at different levels: locally within a component or globally across the entire application. Understanding when and how to use local and global state is crucial to designing well-structured and maintainable applications.
Local State
Definition: Local state is the state that belongs to and is managed by a single component. Only the component that defines the local state can access or modify it.
Characteristics:
- Encapsulation: The state is encapsulated within the component, making it easier to understand and maintain.
- Controlled: Only changes within the component affect its state.
- Specific Use: Suitable for temporary and component-specific data.
Example of Local State:
In a functional component:
jsx
In this example, the state name
is local to the LocalStateComponent
component.
Global State
Definition: Global state is shared among multiple components of an application. Unlike local state, global state can be accessed and modified by different components at various levels of the component tree.
Characteristics:
- Shared: Multiple components can read and update the global state.
- Centralized: Usually managed in a central location to facilitate access and consistency.
- Complex Applications: Needed in applications where several components need to synchronize or share data.
Example of Global State:
One way to manage global state in React is by using the Context API:
jsx
In this example, the state user
is global and accessible to any component that consumes AppStateContext
.
Explanation Image
When to Use Local State vs. Global State?
Local State:
- Data that is specific to a component.
- Does not need to be shared with other components.
- Examples: Forms, inputs, specific UI controls.
Global State:
- Data that needs to be accessed by multiple components.
- Requires consistency across various parts of the application.
- Examples: User information, application settings, global themes.
Understanding the difference between these two types of state and using them appropriately will help make your React application more scalable and maintainable. In the next section, we will delve deeper into using useState and setState to manage state in functional and class components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
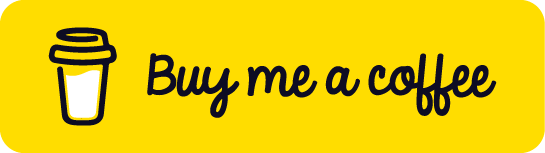
Chat with Chuck
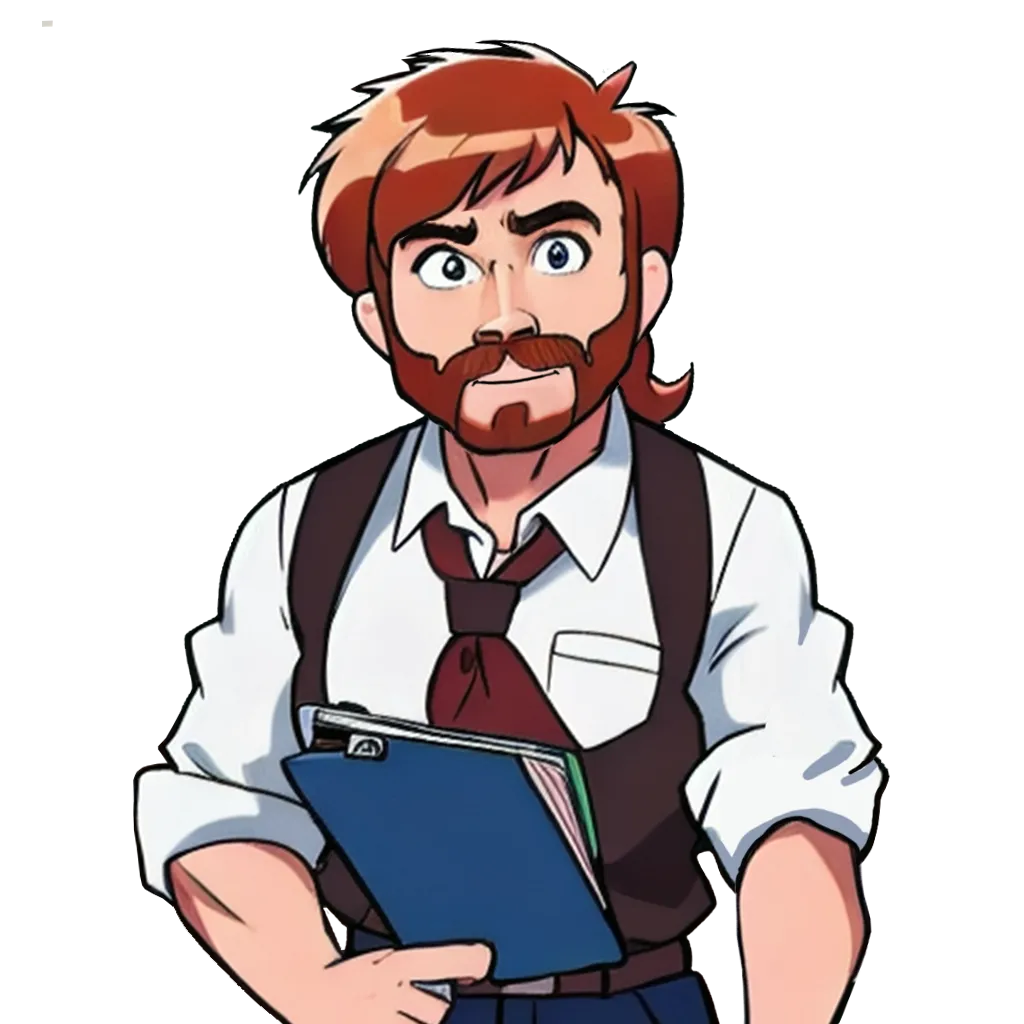
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future