State Management in React
Managing Asynchronous State with useEffect
When developing React applications, we often need to work with asynchronous operations such as API calls, timers, and other side effects. useEffect
is the React hook designed to handle these side effects in functional components. In this section, we will explore how to use useEffect
to manage asynchronous state efficiently and effectively.
Introduction to useEffect
useEffect
is a hook that allows you to perform side effects in functional components. It runs after the component renders and can be used to handle operations such as:
- Fetching data
- DOM manipulations
- Subscriptions
- Timers
Basic Syntax:
jsx
- Side effect: The function that contains the side effect code.
- Cleanup: (Optional) The function that cleans up the previous effects before the component updates or unmounts.
- Dependencies: (Optional) An array of dependencies that determines when the effect will re-run.
Basic Example with useEffect
To illustrate the use of useEffect
, let's consider a simple example where the document title is updated:
jsx
In this example:
useEffect
updates the document title each timecount
changes.- The dependency
[count]
ensures that the effect only runs whencount
changes.
Managing Asynchronous Calls with useEffect
One of the most common applications of useEffect
is to handle API calls. Let's look at an example where we fetch data from an API.
Example: Fetching Data
jsx
In this example:
useEffect
runs once when the component mounts, due to the empty dependency array[]
.fetchData
is an asynchronous function that fetches data from the API and updates the state.
Using Cleanup in useEffect
When working with side effects, it's important to clean up those effects when the component unmounts or updates to avoid memory leaks or unwanted behaviors.
Example: Timer with Cleanup
jsx
In this example:
setInterval
is used insideuseEffect
to update theseconds
state every second.- The cleanup function
clearInterval
ensures the timer is stopped when the component unmounts.
Common Patterns with useEffect
- Effect only on Mount: Use
[]
as dependencies to run the effect only when the component mounts. - Dependent Effect: List of dependencies that control when the effect should re-run.
- Effect with Cleanup: Perform cleanup to avoid memory leaks or unwanted effects.
Illustrative Image
[Placeholder: "Diagram illustrating the lifecycle of a component with useEffect
, showing when effects and cleanups are executed."]
Conclusion
useEffect
is a versatile and powerful tool for handling side effects and asynchronous states in React functional components. Understanding how and when to use useEffect
will allow you to manage asynchronous operations efficiently and keep your code clean. In the next section, we will explore State Management with Redux, a popular library for managing global state in React applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
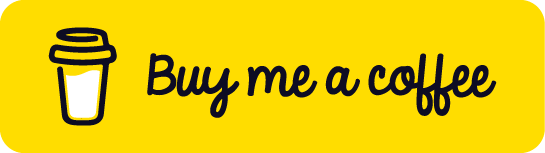
Chat with Chuck
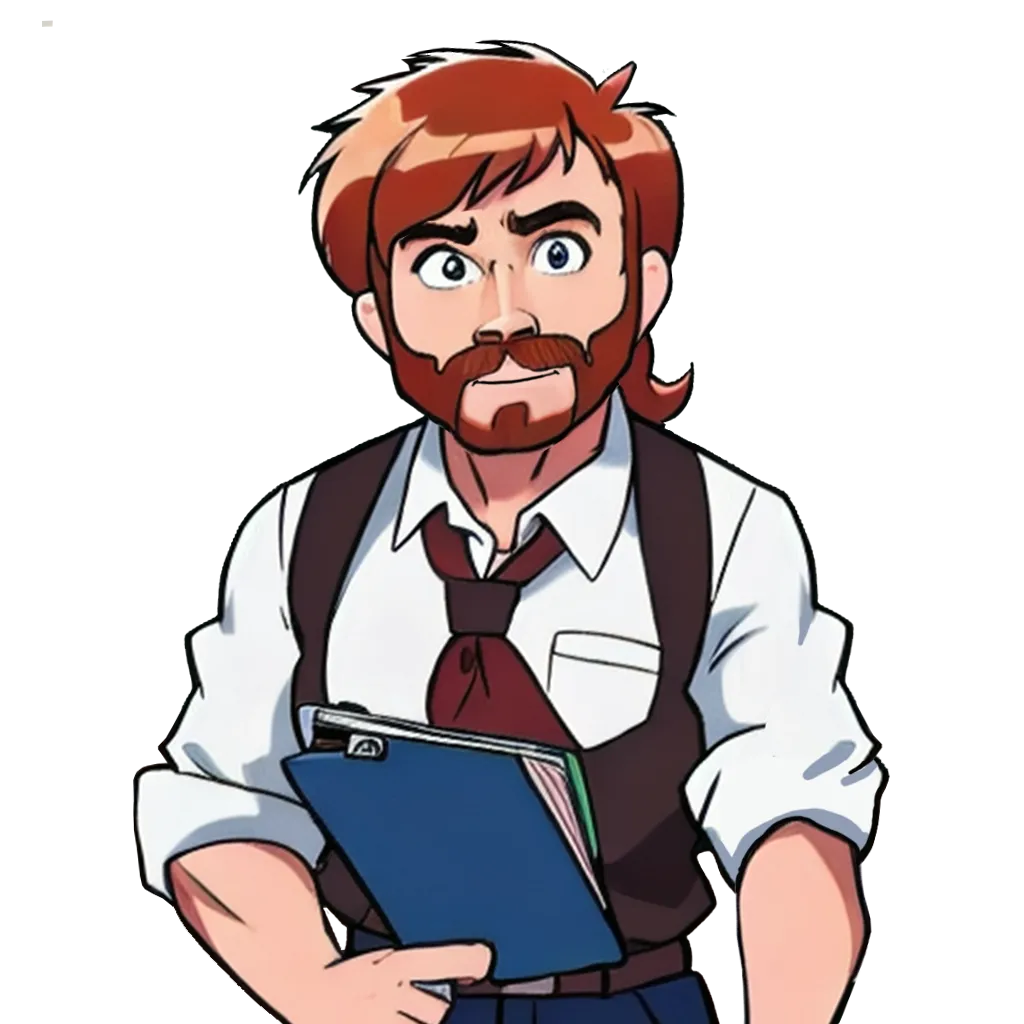
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future