State Management in React
Using Reducers and useReducer
useReducer
is a React hook that offers an alternative way to manage state in functional components. It is particularly useful for handling complex states and update logic, providing a structure similar to state management with Redux
.
What is a Reducer?
A reducer is a function that determines how the state will change in response to an action. This function receives two parameters: the current state and an action. The action is an object that contains at least a type
property indicating the type of action to perform.
Basic Reducer Example:
jsx
Using useReducer
useReducer
takes three parameters:
- reducer: The reducer function that handles state updates.
- initialState: The initial state.
- init: (Optional) An initialization function used for complex cases.
The call to useReducer
returns two values:
- state: The current state.
- dispatch: A function to send actions to the reducer.
Basic Syntax:
jsx
Basic Example with useReducer
Let's implement a simple counter using useReducer
:
jsx
Handling Complex States
For more complex states, useReducer
provides a clearer and more manageable structure compared to useState
.
Form Example with useReducer
:
jsx
When to Use useReducer
- Complex States: When your component state involves multiple sub-values or deep changes based on different actions.
- Complex State Logic: When the state update logic is complicated and requires a structured and clear handling.
- Readability and Maintainability: To improve code readability and maintainability in larger applications.
Benefits of useReducer
- Clear Structure: It offers a clear structure for handling state changes based on actions.
- Efficiency: It improves code efficiency by handling more complex states without needing multiple
useState
calls. - Consistency: It facilitates the implementation of consistent and predictable design patterns for state handling.
Explaining Image
[Placeholder: "Diagram showing how useReducer
manages state compared to useState
, illustrating the flow of actions through the reducer function."]
Conclusion
Using useReducer
in React is a powerful technique for managing complex states and update logic clearly and efficiently. Understanding when and how to use useReducer
will enable you to create more robust and maintainable React applications. In the next section, we will explore Handling Asynchronous State with useEffect
, which is fundamental for working with side effects in functional components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
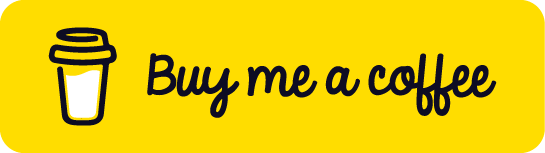
Chat with Chuck
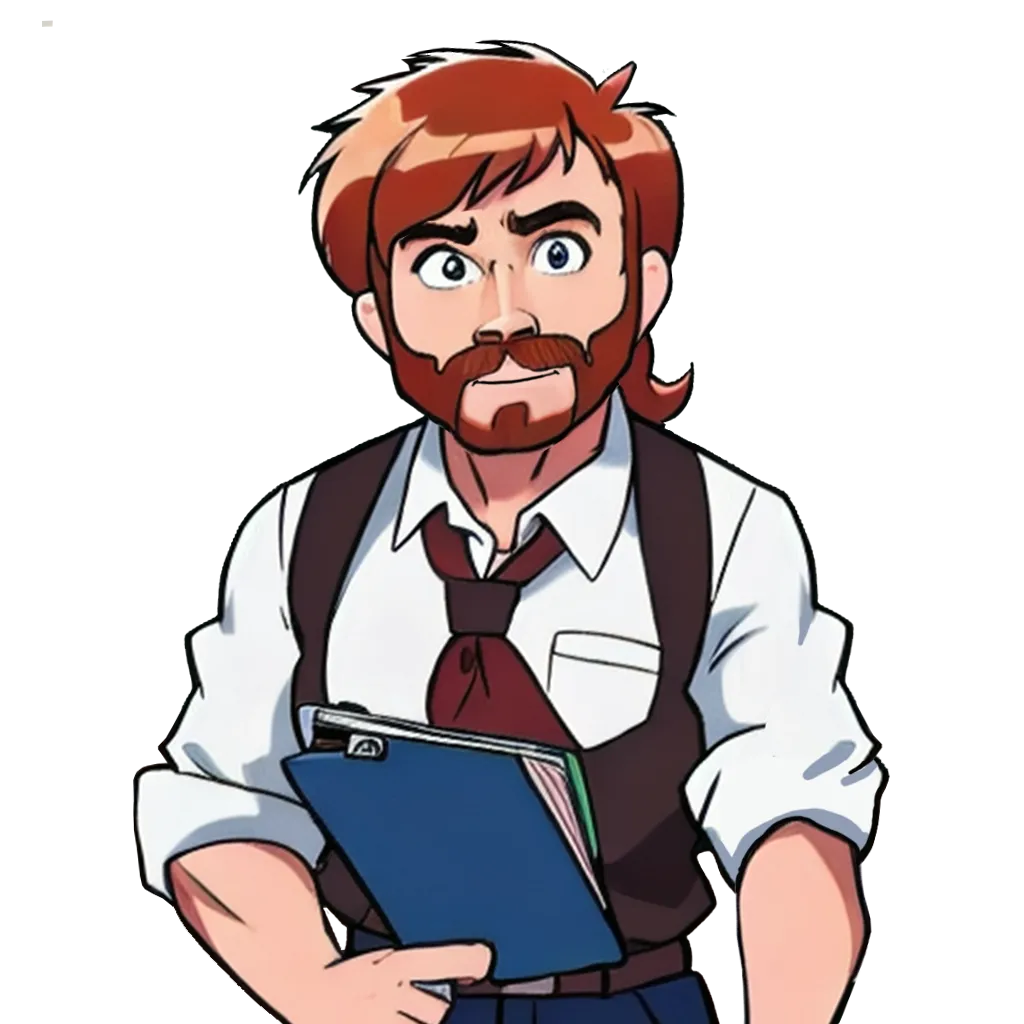
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future