State Management in React
Fundamentals of State in React
Now that we have introduced the importance of state management in React, it's crucial to understand its fundamentals. This section will cover the core concepts that underpin how React handles state and how you can efficiently use it in your applications.
What is State?
State is a representation of the internal data of a component that can change throughout the component's lifecycle. Each component in React can maintain its own local state. When a component's state changes, React efficiently updates the DOM to reflect those changes.
Properties of State
1. State Initialization:
State can be initialized in the constructor of a class component or via hooks in functional components.
Example in Class Component:
jsx
Example in Functional Component:
jsx
2. State Update:
State must be updated using specific functions (setState
for classes, useState
setters for functions) to ensure React knows when to re-render the component.
Example:
jsx
3. State Immutability:
State should be treated as immutable. It should never be modified directly, but rather, a new copy of the state should be created and set.
Component Lifecycle Methods Related to State
In class components, there are several lifecycle methods that manage state:
- componentDidMount(): Called once the component has been mounted. Ideal for initializing state that depends on external data.
- componentDidUpdate(prevProps, prevState): Called after the component has been updated. Allows for comparison of previous and current state.
- componentWillUnmount(): Called just before the component is unmounted. Useful for cleaning up resources.
Practical Example: Timer
Let's look at an example of a timer, first in a class component and then in a functional component.
Class Component:
jsx
Functional Component:
jsx
Explanatory Image
By understanding these fundamentals, you'll be able to manage the state of any component in React more clearly and effectively. In the next section, we'll explore the differences between Local State and Global State and when to use each.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
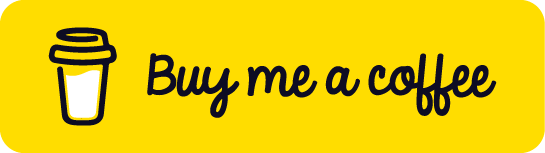
Chat with Chuck
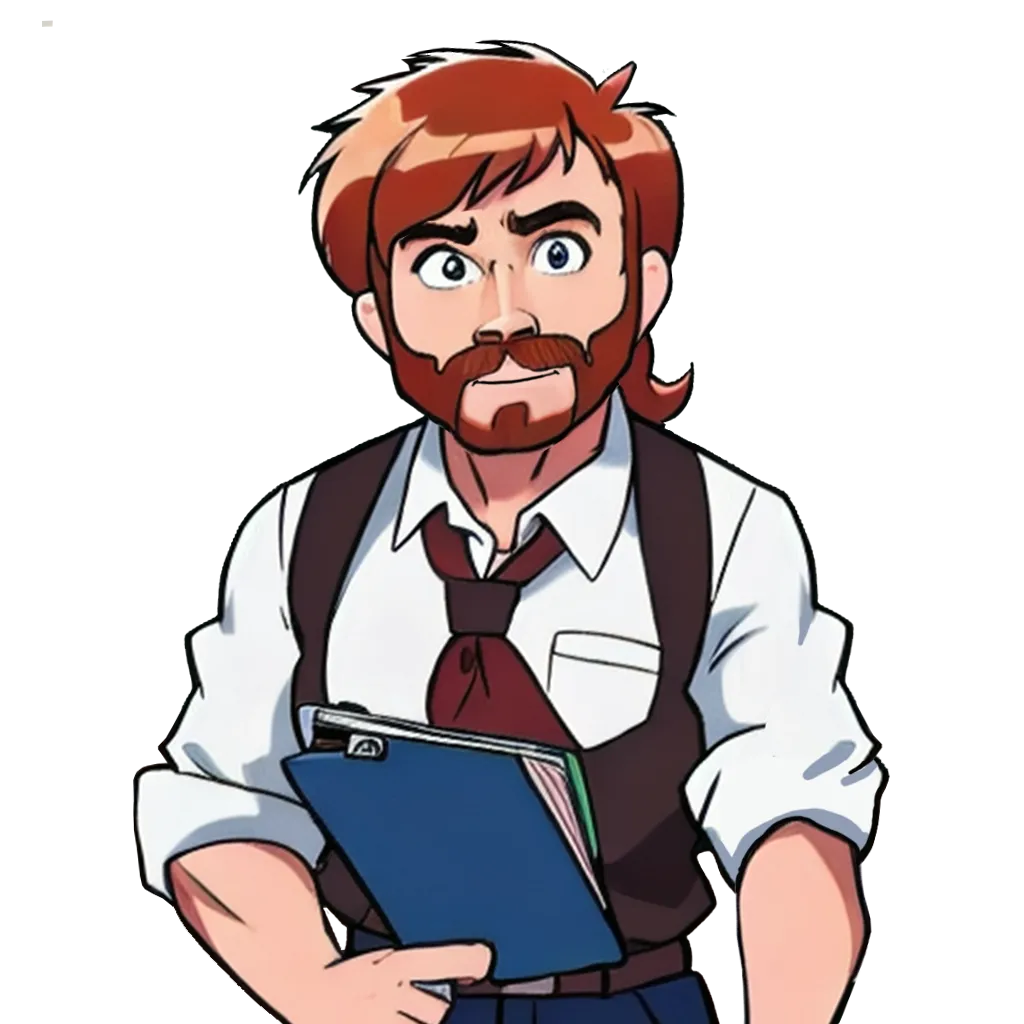
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future