State Management in React
State Management with Redux
Redux is a popular library for managing the global state of JavaScript applications, including React applications. It provides a predictable and structured architecture for handling complex states, making your application easier to understand, debug, and test. In this section, we will learn how to integrate Redux into a React application and how to manage the global state using this powerful tool.
What is Redux?
Redux is based on three fundamental principles:
- Single state tree: The entire application has a unique state object that is stored in a "store."
- State is read-only: The only way to change the state is to emit an action, an object describing what happened.
- Changes are made with pure functions: To specify how the state changes in response to an action, pure functions called "reducers" are used.
Installing Redux and React-Redux
To start using Redux in your React application, you first need to install the necessary libraries:
sh
Store Configuration
A "store" in Redux is the only place where the application state is stored. It can be created using Redux’s createStore
function.
Basic Example:
jsx
Store Provider
Provider
is a component from react-redux
that makes the Redux store available to your entire application. We wrap our application structure within this component.
Example:
jsx
Connecting Components to Redux
To connect React components to the Redux store, we use the useSelector
and useDispatch
hooks from react-redux
.
Example of Connected Component:
jsx
In this example:
useSelector
is used to extract the value ofcount
from the store state.useDispatch
is used to dispatch actions to the store.
Actions and Reducers
Actions are objects that describe a state change in the application, and reducers specify how the state changes when an action is received.
Example of Actions and Reducers:
jsx
jsx
Explaining Image
Advantages of Using Redux
- Centralized State: All application state is stored in a single tree, making management and debugging easier.
- Predictable: Redux architecture makes your application behavior more predictable and easier to understand.
- Debugging Tools: Redux DevTools provides powerful tools for inspecting and debugging the application state.
- Scalability: It facilitates scalability of large applications thanks to its clear and modular structure.
Considerations and Best Practices
- Maintain Simplicity: It's not necessary to use Redux for small applications where
useState
anduseContext
would suffice. - Clear Actions: Keep actions clear and descriptive to facilitate maintenance and debugging.
- State Normalization: Structure the state in a way that is easy to update and maintain, avoiding deep nesting.
Redux provides a robust and scalable way to handle state in React applications. In the next section, we will explore the internal components of Redux more deeply, specifically Actions, Reducers, and the Store in Redux.
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future
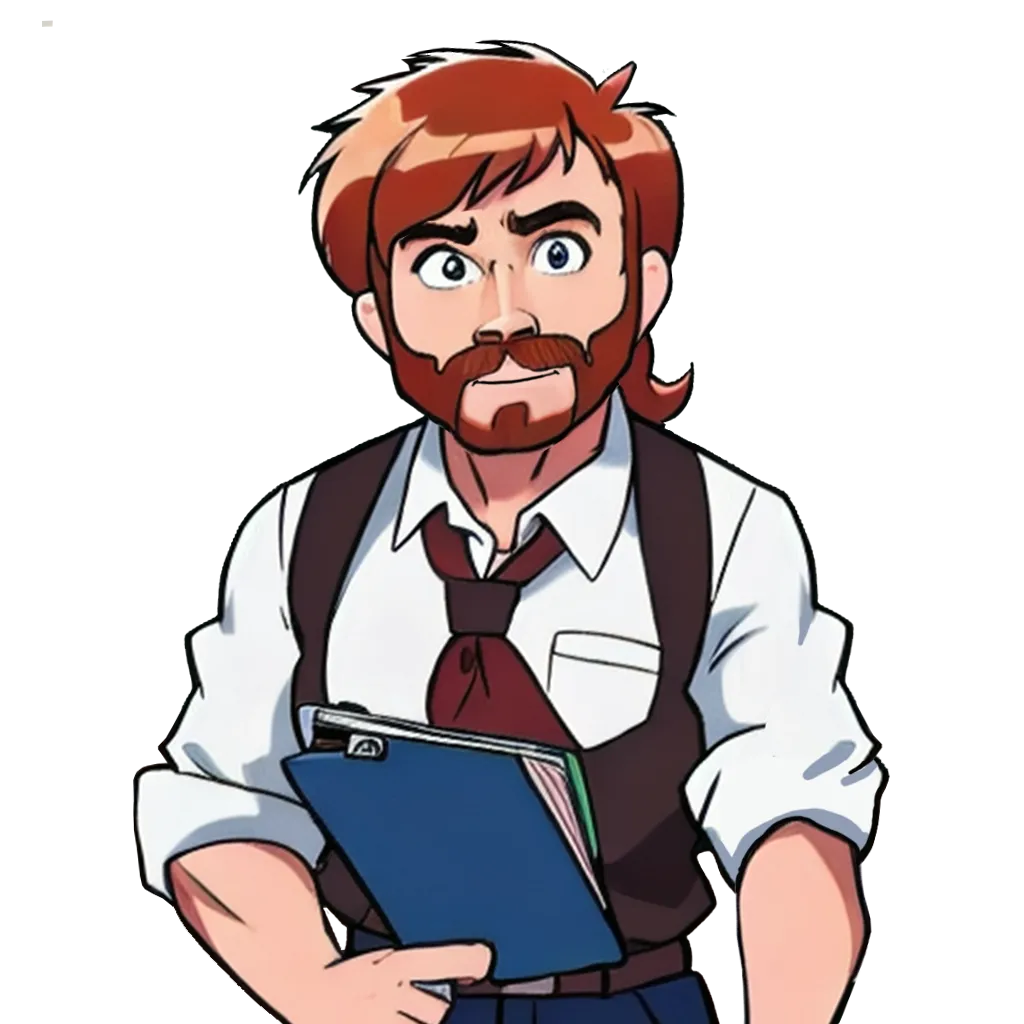