State Management in React
Introduction to State Management in React
In modern web application development, one of the most challenging tasks is managing application state. React, a JavaScript library for building user interfaces, provides several powerful tools to efficiently manage state. Proper state management is crucial for creating applications that are interactive, efficient, and easy to maintain.
In this section, we will introduce the basic concepts of state management in React. We will see what state is, why it is important, and how React facilitates its management in both functional and class components.
What is State in React?
State in React refers to a structure that holds information that can change during the lifetime of a component. State allows React components to respond to user interactions and other events; in other words, it allows a React application to be dynamic and interactive.
Why is State Important?
State management is fundamental because:
- Interactivity: It allows the user interface to react to user inputs.
- Reactivity: With state, whenever the information changes, React automatically updates the UI to reflect those changes.
- Synchronization: It helps keep different parts of the application's interface synchronized.
How Does React Manage State?
React offers two main ways to manage state:
- Class Components: Used
this.state
andthis.setState
to manage state. - Functional Components: Use hooks like
useState
anduseReducer
to manage state in a simpler and more concise manner.
Basic Example: State in a Functional Component
Below is a simple example using React and the useState
hook to manage a counter:
jsx
In this example:
- We import the
useState
hook from React. - We declare a
count
variable and asetCount
function to update that state. - We use the state in the user interface and allow the button to increment the counter.
Explanatory Image
This introduction prepares us to delve into the details of state management with React, exploring from basic concepts to advanced techniques and best practices.
In the next section, we will dive into the Fundamentals of State in React and see how the state serves as a cornerstone for developing interactive applications with this library.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
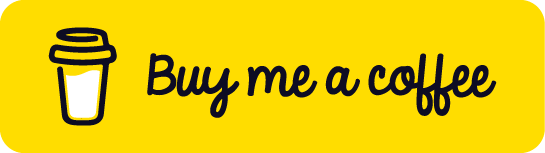
Chat with Chuck
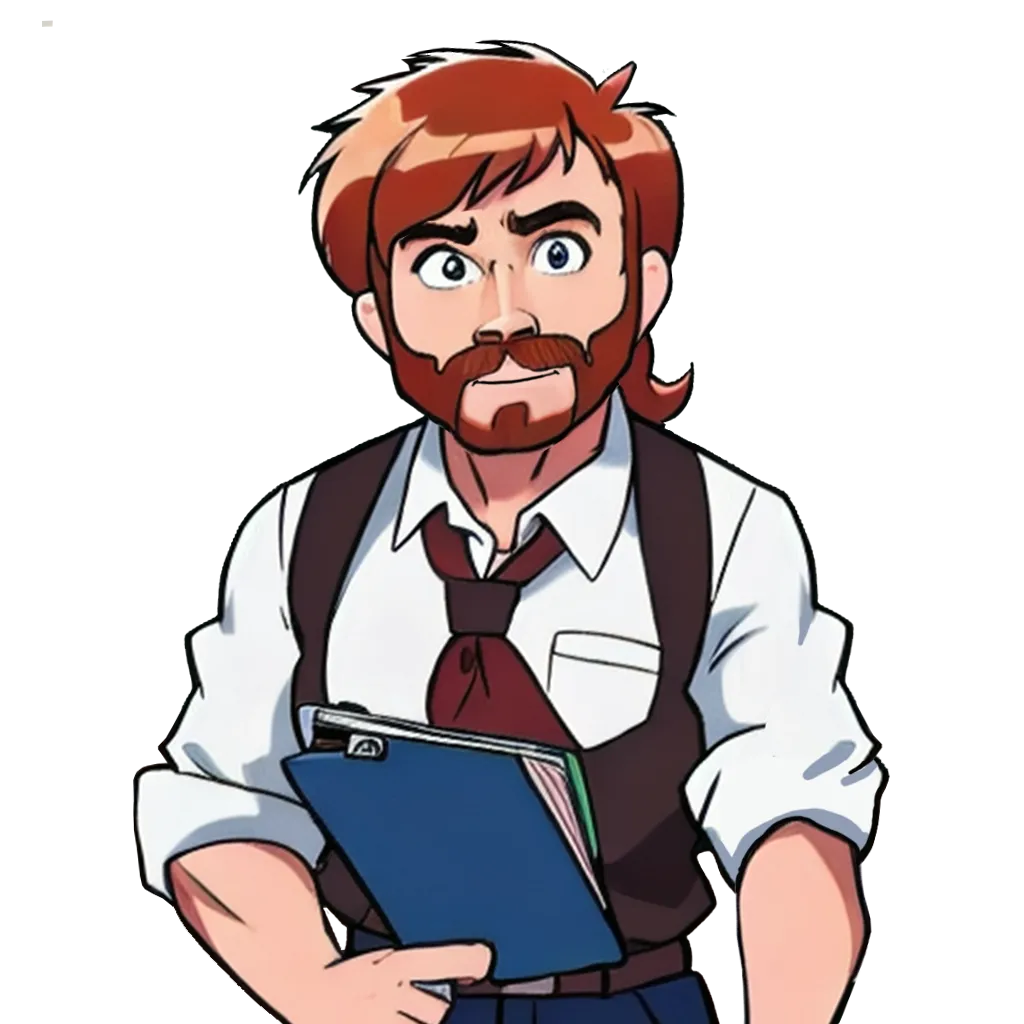
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future