State Management in React
Event Handling and State Updating
Events play a fundamental role in React applications, as they allow user interactions that can trigger state changes. In this section, we will learn how to handle events and how to update the state in response to these events in both functional and class components.
Events in React
React normalizes native browser events to ensure events behave consistently across all browsers. To handle events in React, camelCase event names are used, and functions are passed as event handlers.
Basic Syntax:
jsx
Event Handling in Functional Components
In functional components, events are handled using regular functions or arrow functions, and state updates are performed through hooks such as useState
.
Simple Example:
jsx
Event Handling in Class Components
In class components, events are typically handled with instance methods. It is necessary to bind these methods to ensure that this
refers to the correct component.
Simple Example:
jsx
Binding Methods in Class Components
It is important to remember that in class components, you must bind event handler methods to ensure that this
points to the appropriate component.
Binding Methods:
- In the Constructor:
jsx
- Arrow Function as Class Property:
jsx
Common Events in React
onClick
The onClick
event is triggered when an element is clicked.
jsx
onChange
The onChange
event is commonly used with inputs to capture changes in values.
jsx
onSubmit
The onSubmit
event is triggered when a form is submitted.
jsx
Practical Example: Registration Form
Functional Component:
jsx
Class Component:
jsx
Explanatory Image
Event handling and state updating are key pieces to creating interactive and dynamic React applications. In the next section, we will learn about Passing State Between Components with Props, which is crucial for sharing data and coordinating actions between different parts of a React application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
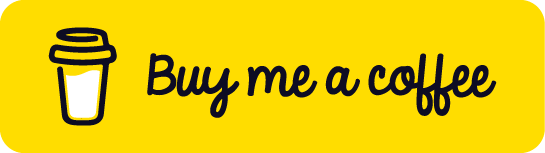
Chat with Chuck
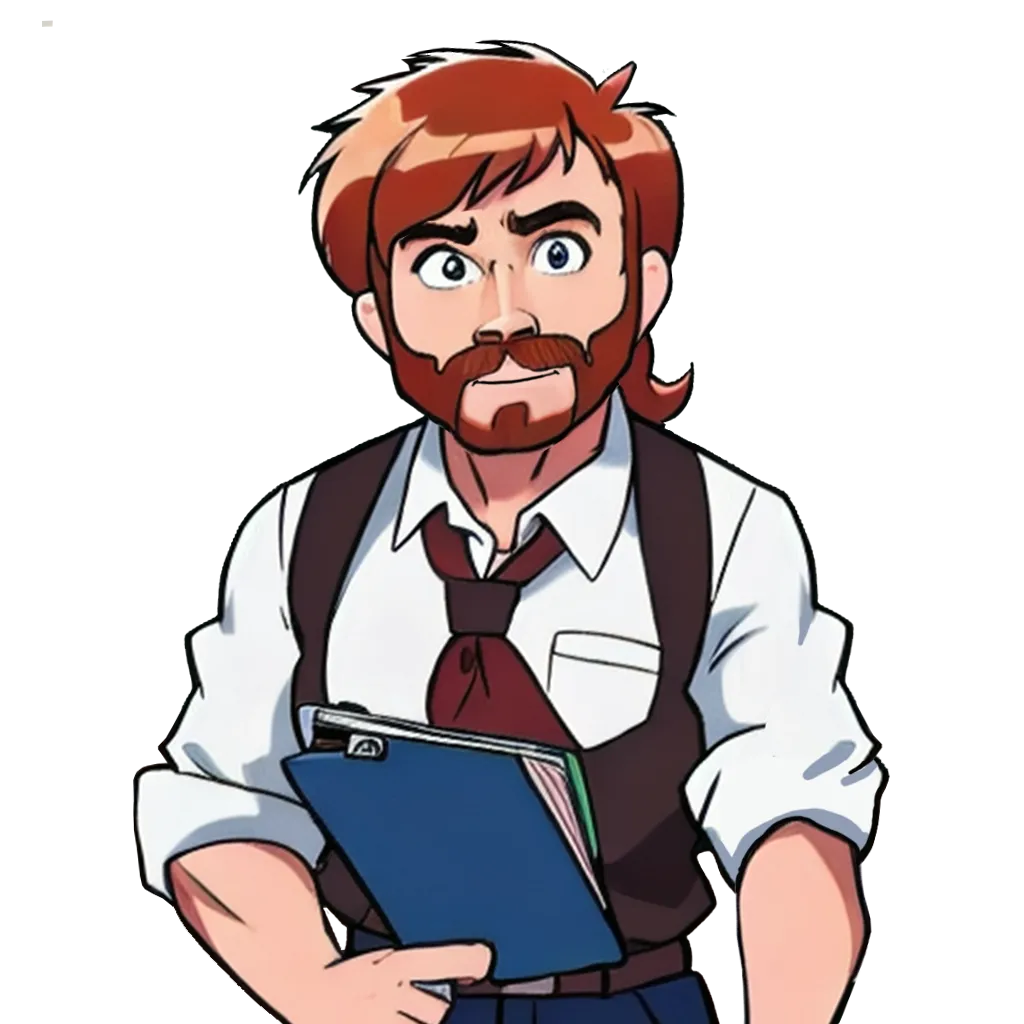
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future