State Management in React
Context API for Global State Management
In complex React applications, managing global state can become a challenge, especially when multiple components need to access and update the same state. React's Context API is an effective solution to this problem, allowing you to share global state between components without the need to manually pass props through each level of the component tree.
What is the Context API?
React's Context API provides a way to pass data through the component tree without having to pass props manually at every level. It is ideal for sharing data that needs to be accessible to many components, like the authenticated user, themes, app settings, etc.
Main Components of the Context API
1. React.createContext
Creates a Context
with a Provider
and a Consumer
. Provider
is a component that provides the state and Consumer
is a component that consumes the state.
jsx
2. Context.Provider
Wraps components that need to access the context and provides the state value to the child components.
3. Context.Consumer
Consumes the context value. With hooks, useContext
is generally used instead of Context.Consumer
.
Creating a Global Context
Step 1: Creating the Context
jsx
Step 2: Using the Context in Components
Parent Component
jsx
Child Components
jsx
jsx
Benefits of the Context API
- Simplicity: Provides a clear and easy way to share state between multiple components without manually passing props.
- Flexibility: Allows you to maintain state in a centralized location and update it from any component that consumes it.
- Readability: Reduces the amount of repetitive code and makes the data flow easier to understand.
Explanatory Image
[Placeholder: "Diagram illustrating the data flow with Context API, showing a Provider
wrapping several components and sharing the global state."]
Considerations and Best Practices
- Limit Global Context: Use Context for truly global data. For more localized states, it's preferable to keep using props or internal component states.
- Re-rendering: Every time the context value changes, all consumers within the
Provider
will re-render. Optimize context usage to avoid unnecessary re-renderings. - Context Division: If the application has multiple independent values, it's better to create several small contexts instead of one large one to improve efficiency and clarity.
The Context API is a powerful tool for managing global state in React applications, making it easier to share and update data efficiently. In the next section, we will explore Using Reducers and useReducer, providing a more structured way of handling complex states.
- Introduction to State Management in React
- Fundamentals of State in React
- Local State vs. Global State
- Using useState and setState
- Event Handling and State Updating
- Passing State between Components with Props
- Context API for Global State Management
- Using Reducers and useReducer
- Managing Asynchronous State with useEffect
- State Management with Redux
- Actions, Reducers, and the Store in Redux
- Integrating Redux with React
- Herramientas y Middleware para Redux
- Best Practices in State Management
- Conclusions and Best Resources for the Future
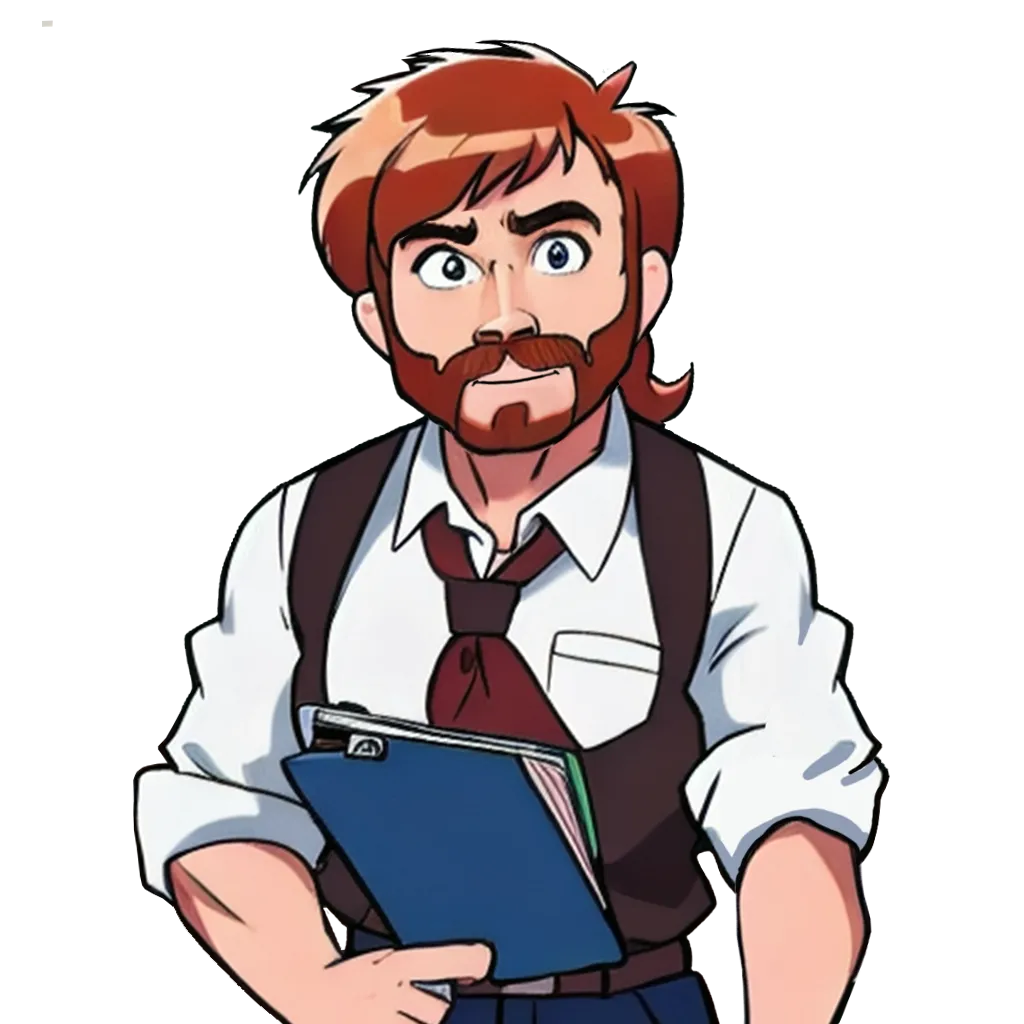