DOM Testing Library
Accessibility Testing with Testing Library
Accessibility is a crucial aspect of web development as it ensures that applications are usable by people with diverse abilities. In this chapter, we will learn how to perform accessibility testing using DOM Testing Library to ensure our applications meet accessibility standards.
What is Web Accessibility?
Web accessibility refers to the practice of designing and developing web applications that can be used by anyone, regardless of their physical or cognitive abilities. This includes ensuring that applications are navigable via keyboard, that content is readable by screen readers, and that appropriate semantic tags are used.
Introduction to Jest-DOM
DOM Testing Library works well in conjunction with jest-dom
, an extension of Jest that provides a series of conveniences for accessibility-specific assertions. To use jest-dom
, you need to install it first:
bash
Then, add it to your Jest configuration. You can do this in your Jest config file or directly in the test files:
File setupTests.js
:
javascript
Make sure to import this file in your Jest configuration:
json
Verifying ARIA Roles and Attributes
Accessible applications often use roles and ARIA attributes (aria-*
) to provide additional information to screen readers and other assistive devices.
File index.js
(component with ARIA attributes):
javascript
File accessibility.test.js
:
javascript
Simulating Accessible Interactions
Accessibility tests should also include simulating accessible interactions, such as keyboard navigation.
File navigation.test.js
:
javascript
Integration with Axe for Automatic Validations
Axe-core is a robust library for automatically verifying accessibility best practices. It can be integrated with Jest and DOM Testing Library using jest-axe
.
-
Installation:
bash -
Usage in tests: File
axe.test.js
:
javascript
[Placeholder for explanatory image: Diagram illustrating how jest-axe
analyzes the DOM and discovers potential accessibility issues]
Benefits of Accessibility Testing
- Usability Improvement: Ensures that all users can efficiently use the application.
- Regulatory Compliance: Helps comply with accessibility standards and regulations like WCAG.
- SEO Improvement: Accessible web applications often see improved performance in search engines.
Conclusion
In this chapter, we learned how to perform accessibility testing using DOM Testing Library along with tools like jest-dom
and jest-axe
. Ensuring your application is accessible is crucial for offering an inclusive experience and meeting web standards.
In the next chapter, we will explore how to handle asynchronous testing with DOM Testing Library.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
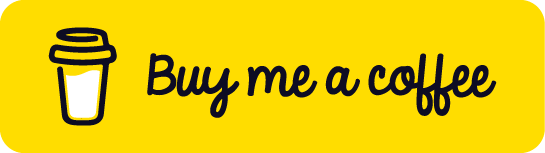
Chat with Chuck
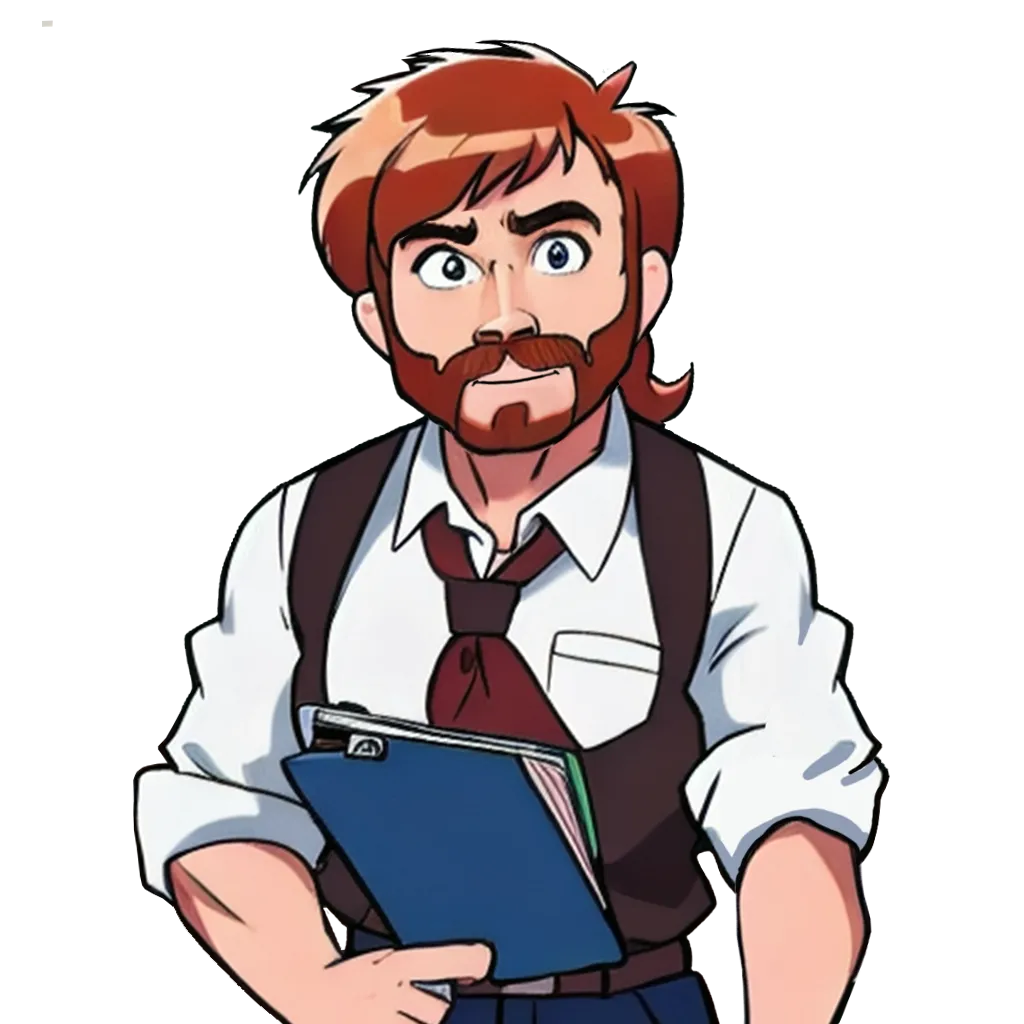
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library