DOM Testing Library
Writing Your First Unit Tests with Testing Library
In this chapter, we will focus on writing our first unit tests using DOM Testing Library. Unit tests allow you to verify small parts of your code in isolation, ensuring each component works as expected.
Creating a Simple Component
To start, let's create a basic component in HTML and JavaScript:
File index.html
:
html
File index.js
:
javascript
Writing a Unit Test
Let's write a unit test to verify that the button is rendered correctly in the DOM.
File button.test.js
:
javascript
Understanding the Test
-
Initial Setup:
beforeEach
: Runs before each test, creating adiv
container and appending it to thebody
.afterEach
: Cleans up the DOM by removing the container after each test.
-
Writing the Test:
- We call the
renderButton
function passing the container. - We use the
getByText
function from DOM Testing Library to find the button by its text. - We use Jest's
expect
to verify that the button is in the DOM.
- We call the
[Placeholder for an explanatory image: A simple diagram showing the test flow from creating the container, rendering the button, to verifying]
Additional Tests
Let's look at other unit tests that can be applied to the same component.
Verify the Existence of an Attribute
We will verify that the button has the id
attribute set correctly.
javascript
Verify Click Behavior
Suppose we want to add a click behavior to our button.
Modified file index.js
:
javascript
Add test in button.test.js
:
javascript
Conclusion
In this chapter, we learned how to write our first unit tests using DOM Testing Library. We saw how to verify the presence of elements in the DOM and how to simulate events to validate component behavior.
These initial unit tests lay the groundwork for more advanced tests that we will explore in upcoming chapters, where we will look at how to test more complex components and their interactions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
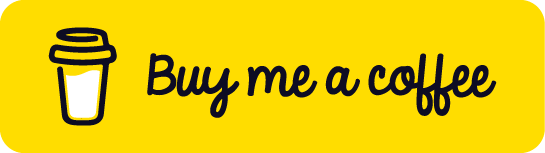
Chat with Chuck
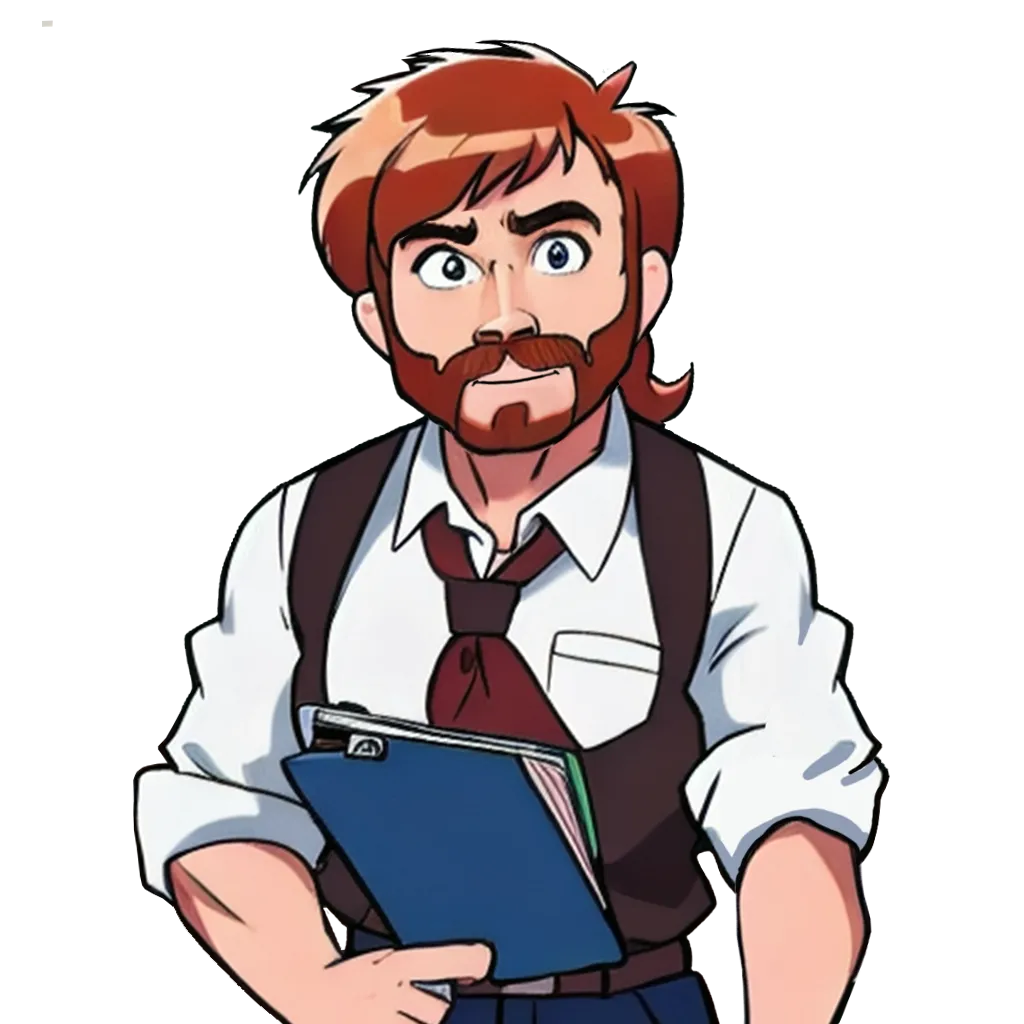
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library