Testing JavaScript and DOM with DOM Testing Library
Mocking and Stubbing in Testing Library
When writing tests, it is often necessary to mimic certain behaviors or dependencies to isolate the code we are testing. This process is known as "mocking" and "stubbing". In this chapter, we will learn how to perform these techniques using DOM Testing Library along with Jest.
What is Mocking and Stubbing?
- Mocking: Creating fake implementations of functions or modules to simulate their behavior.
- Stubbing: Replacing a specific function with a predefined version that simulates a response or behavior.
Example Scenario
We will use a scenario where a component makes an API call to fetch data and displays the result. Instead of making a real API call, we will use mocking and stubbing to simulate the response.
Create the Component
index.js
file:
javascript
Testing with Mocking using Jest
We will write a test that simulates the API call and verifies that the component correctly displays the data.
component.test.js
file:
javascript
Stubbing with Jest
In some cases, we may not need to mock an entire function or module, but simply replace a specific function.
Example of Stubbing:
javascript
[Placeholder for explanatory image: Diagram showing how mocking and stubbing are done in Jest, from simulating the function to verifying results in the DOM]
Benefits of Mocking and Stubbing
- Test Isolation: Allows testing components in isolation without relying on external services.
- Consistency: Ensures consistent test results by controlling responses.
- Time Reduction: Mocking and stubbing can make tests faster by avoiding real network calls.
Conclusion
In this chapter, you have learned how to use mocking and stubbing in your tests with DOM Testing Library and Jest. These techniques are essential to ensure that you can test your code in isolation, guaranteeing reliable and consistent results regardless of external dependencies.
In the upcoming chapters, we will delve into testing complex user interactions and accessibility testing.
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library
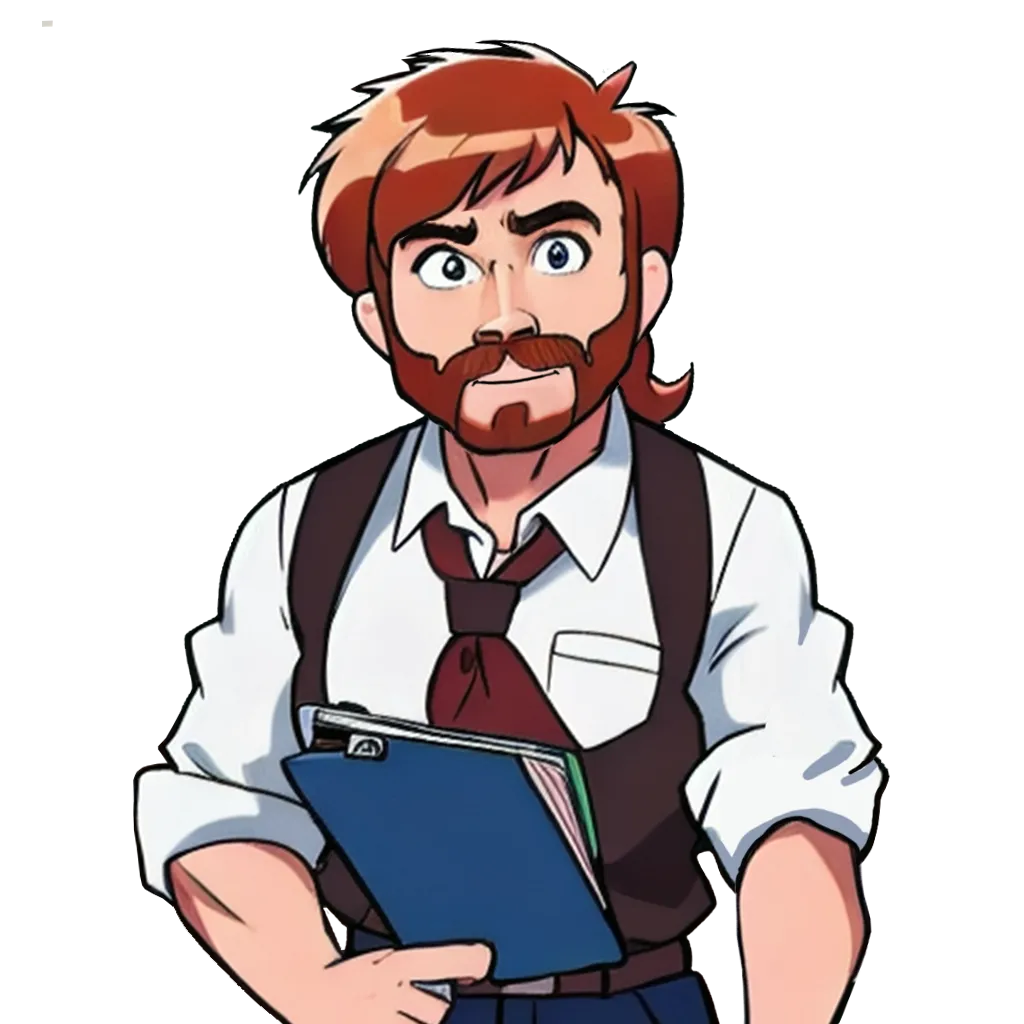