DOM Testing Library
Introduction to Testing in JavaScript with Testing Library
Welcome to the "Testing JavaScript and DOM with DOM Testing Library" course. In this course, we will explore how and why to test your JavaScript applications using the DOM Testing Library. We will see how this tool can help you write more effective and maintainable tests, focusing on the user experience.
What is Testing in JavaScript?
Testing in JavaScript involves writing and running scripts that verify the correct functioning of the code. These scripts ensure that your application does what you expect and that new changes do not break existing functionalities. There are several levels of testing:
- Unit Tests: Verify the functionality of small parts of the code.
- Integration Tests: Ensure that multiple components work together correctly.
- End-to-End (E2E) Tests: Verify that the entire application works correctly in real-world scenarios.
What is DOM Testing Library and why use it?
DOM Testing Library is a tool that facilitates testing the Document Object Model (DOM) to verify the functionality of web components from the user's perspective.
Advantages of using DOM Testing Library:
- User-Focused: Allows writing tests centered on how the user interacts with your application.
- Ease of Use: Offers an intuitive API with query methods that mimic user interaction.
- Accessibility Testing: Facilitates writing tests that ensure the accessibility of your web application.
Simple Example
To illustrate the simplicity and power of DOM Testing Library, let's see a basic example of how to verify that a rendered button in the DOM exists and is accessible:
javascript
[Placeholder for explanatory image: Show a simple diagram of how DOM Testing Library interacts with the DOM and unit tests]
Benefits of Automated Testing
Automating tests brings numerous benefits, including:
- Consistency and Repeatability: Tests can be run as many times as needed without variations.
- Early Error Detection: Helps detect errors early in the development cycle.
- Living Documentation: Tests act as living documentation that describes how your application should work.
In summary, testing in JavaScript with DOM Testing Library is an effective way to ensure the quality and functionality of your web applications focusing on the user experience. This course will guide you step by step so you can make the most of this powerful tool.
We hope you enjoy and learn a lot throughout this course. Let's get started!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
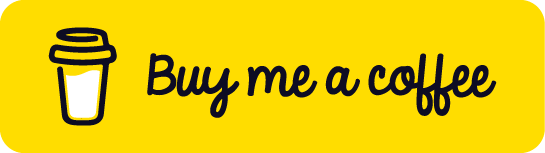
Chat with Chuck
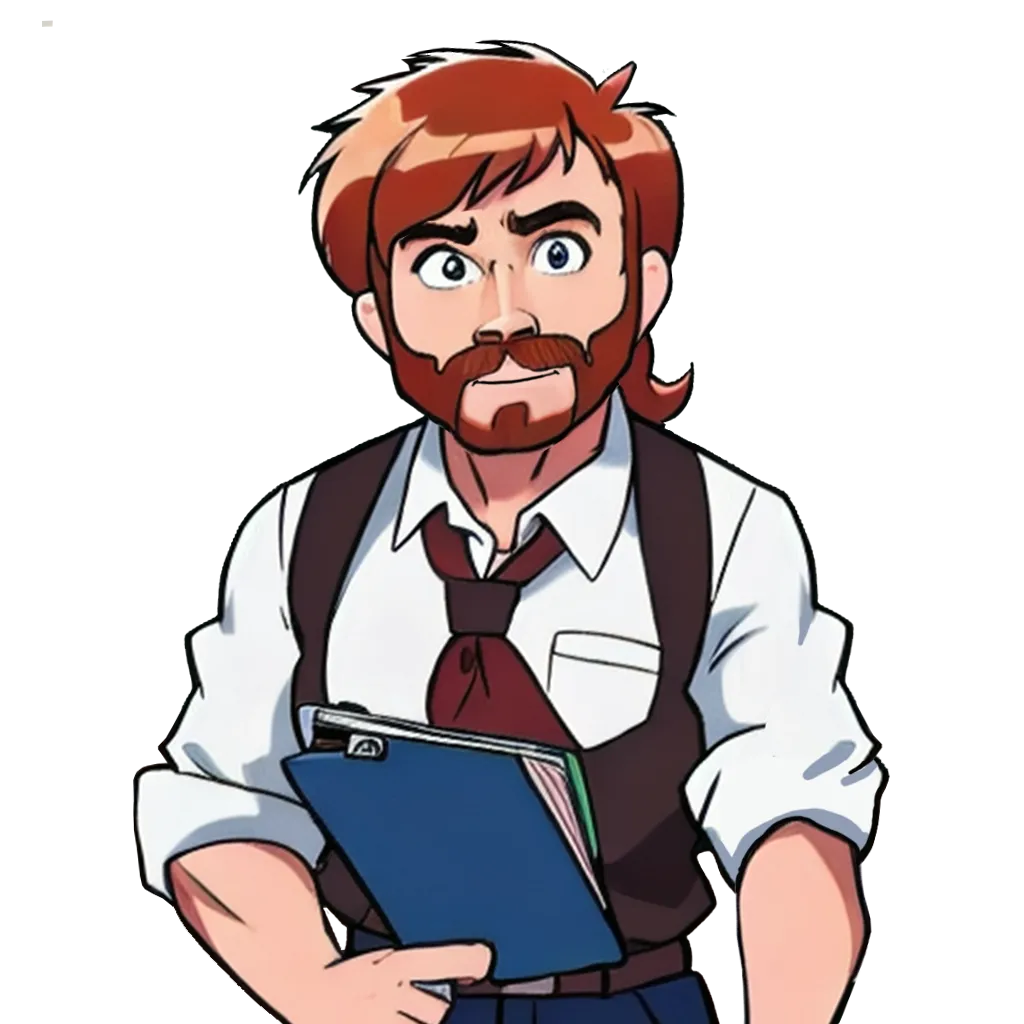
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library