Testing JavaScript and DOM with DOM Testing Library
DOM Fundamentals
Before delving into the use of DOM Testing Library, it is crucial to understand the fundamentals of the Document Object Model (DOM). The DOM is a tree-like representation that allows for the dynamic manipulation of the content, structure, and style of web documents.
What is the DOM?
The DOM is a programming interface for HTML and XML documents. It provides a structural representation of the document and defines how programs can access and modify its content and presentation.
DOM Structure
The HTML document is represented as a tree of nodes, where each node corresponds to a part of the document. The most common types of nodes are:
- Element Nodes: Represent HTML tags like
<div>
,<a>
,<p>
, etc. - Text Nodes: Represent the text content within the elements.
- Attribute Nodes: Represent the attributes of HTML tags (such as
class
,id
,src
).
Accessing and Manipulating the DOM
We can access and manipulate the DOM using various JavaScript APIs. Here are some fundamental methods and properties:
document.getElementById(id)
: Returns the element with the specifiedid
.document.querySelector(selector)
: Returns the first element that matches the specified CSS selector.element.textContent
: Sets or returns the textual content of an element.element.innerHTML
: Sets or returns the inner HTML content of an element.element.setAttribute(name, value)
: Sets the value of an attribute on the element.
Example of DOM Manipulation
Let's create a small example to illustrate how to manipulate the DOM with JavaScript.
Initial HTML:
html
JavaScript (app.js
):
javascript
[Placeholder for explanatory image: Diagram showing the structure of the DOM tree based on the HTML example]
Traversing the DOM Tree
We can traverse the DOM tree to retrieve and manipulate elements. Some useful properties are:
parentNode
: Returns the parent node of the selected node.childNodes
: Returns a collection of child nodes.firstChild
: Returns the first child node.lastChild
: Returns the last child node.
Example:
javascript
Events in the DOM
Events are a fundamental part of the DOM, enabling interactivity in web applications. Some of the most common events are click
, input
, submit
, among others.
Example of Adding an Event:
javascript
[Placeholder for explanatory image: Diagram illustrating event interaction in the DOM, showing how a click
event on a button propagates to the event handler]
Understanding these DOM fundamentals will allow you to perform more effective and precise tests in your web applications. In the upcoming topics, we will apply this foundational knowledge to write interaction tests with DOM Testing Library.
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library
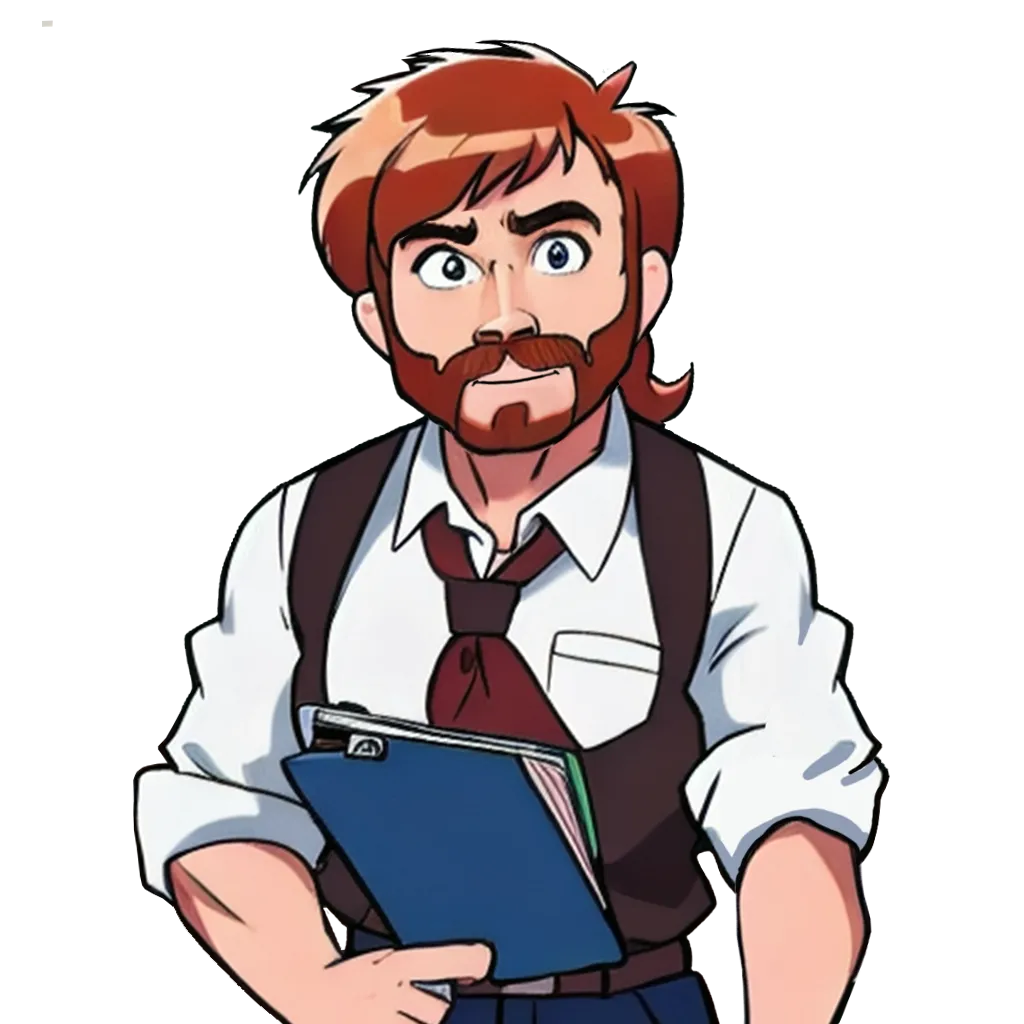