DOM Testing Library
Best Practices for Testing with Testing Library
To ensure that our tests are efficient, maintainable, and robust, it is important to follow some best practices when working with DOM Testing Library. In this chapter, we will discuss a series of recommendations and strategies that will help you write high-quality tests.
Writing Clear and Readable Tests
-
Descriptive names: Ensure that your test names clearly describe what they are verifying.
javascript -
Comments when necessary: Use comments to explain complex logic or special cases that are not directly evident.
javascript
Keeping Test Code Simple
-
Avoid complex logic in tests: Tests should be as simple as possible. Avoid loops, conditionals, and complex logic.
javascript -
Reuse common setups: Avoid repeating the same setup code in each test by using
beforeEach
and helpers.javascript
Ensuring Test Independence
-
Do not depend on previous tests: Each test should be able to run independently and should not depend on state modified by another test.
javascript -
Use mocks to isolate components: Mock dependencies to isolate the component you are testing.
javascript
Using Robust Selectors
-
Prefer selectors by
role
andlabelText
: These selectors better emulate how users interact with the UI and are more robust against design changes.javascript -
Avoid selectors by ID or classes: ID or class-based selectors are more prone to breaking with minor design changes.
javascript
Validating Built-in Accessibility
- Use jest-axe for automated accessibility: Incorporate
jest-axe
in your tests to automatically validate accessibility best practices.javascript
Ensuring Reliable Asynchronous Tests
-
Use
waitFor
andfindBy
: Ensure that your asynchronous tests properly wait for DOM changes.javascript -
Mocking time operations: Use
jest.useFakeTimers()
to control and speed up timers in your tests.javascript
Code Coverage Strategies
-
Coverage configuration: Set up Jest to collect and generate code coverage reports.
javascript -
Review and act on coverage: Regularly review coverage reports and focus on covering critical areas of your application.
Examples of Best Practices in Tests
javascript
[Placeholder for explanatory image: Diagram illustrating best practices from writing clear and readable tests to validating accessibility and ensuring reliable asynchronous tests]
Conclusion
By following these best practices, you can write more robust, clear, and maintainable tests that not only ensure the correct functionality of your code but also improve the overall quality and user experience of your application.
In the next chapter, we will discuss how to debug failing tests in Testing Library to quickly identify and resolve issues.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
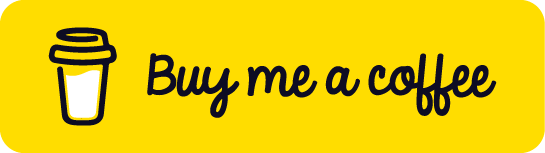
Chat with Chuck
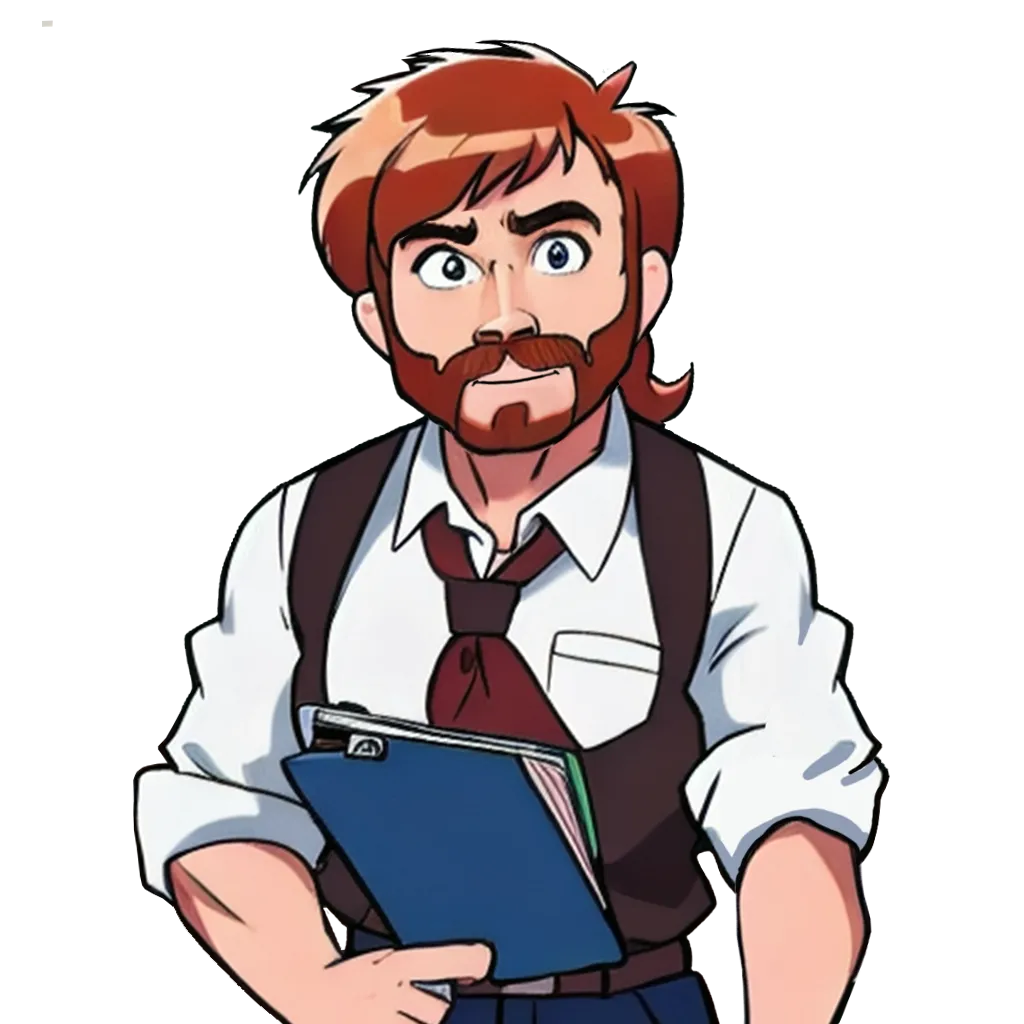
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library