DOM Testing Library
Debugging Failed Tests in Testing Library
When facing failed tests, it is crucial to have an effective debugging strategy. Here we present several techniques and tools you can use to identify and solve issues in your tests.
Using debugger and screen.debug()
To start, you can use the debugger
statement in your code to pause execution at that point and open the debugger in your development environment. Additionally, Testing Library's screen.debug()
prints the current state of the DOM, allowing you to visually inspect which elements are present and their states.
Example:
javascript
Selector Verification
One of the most common issues in failed tests is the use of incorrect or fragile selectors. Make sure you are using the most robust selectors possible, such as getByRole
, getByLabelText
, and getByText
.
Example:
javascript
Waiting for Asynchronous Elements
When testing components that interact with asynchronous operations like API calls, make sure to use waiting methods like waitFor
and findBy
to handle these operations properly.
Example with waitFor
:
javascript
Example with findByText
:
javascript
Mocking and Stubbing
Make sure your mocks and stubs are correctly configured and are not interfering with the expected behavior. You can debug their behaviors by printing results to the console.
Example:
javascript
Browser Debugging Tools
Running your tests in a real browser or in a browser-compatible testing environment (like Jest with the --runInBand
option) can provide additional tools such as the Element Inspector, JavaScript Console, and other browser debugging features.
Live Reload Configuration
Some tools and development environments allow live reload, which can be useful to quickly see changes and debug on the fly.
Complete Example of a Debugging Session
Here is a complete example of a debugging session using several techniques mentioned above.
javascript
[Placeholder for explanatory image: Diagram illustrating the debugging steps, from error identification to correction using the mentioned techniques]
Conclusion
Debugging failed tests can be challenging, but with the right tools and techniques, you can efficiently identify and resolve issues. We have covered various strategies ranging from using screen.debug()
and console.log
to setting breakpoints and correctly utilizing mocks.
In the next and final chapter, we will discuss conclusions and next steps to continue improving your testing skills with DOM Testing Library.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
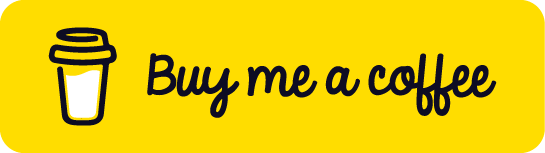
Chat with Chuck
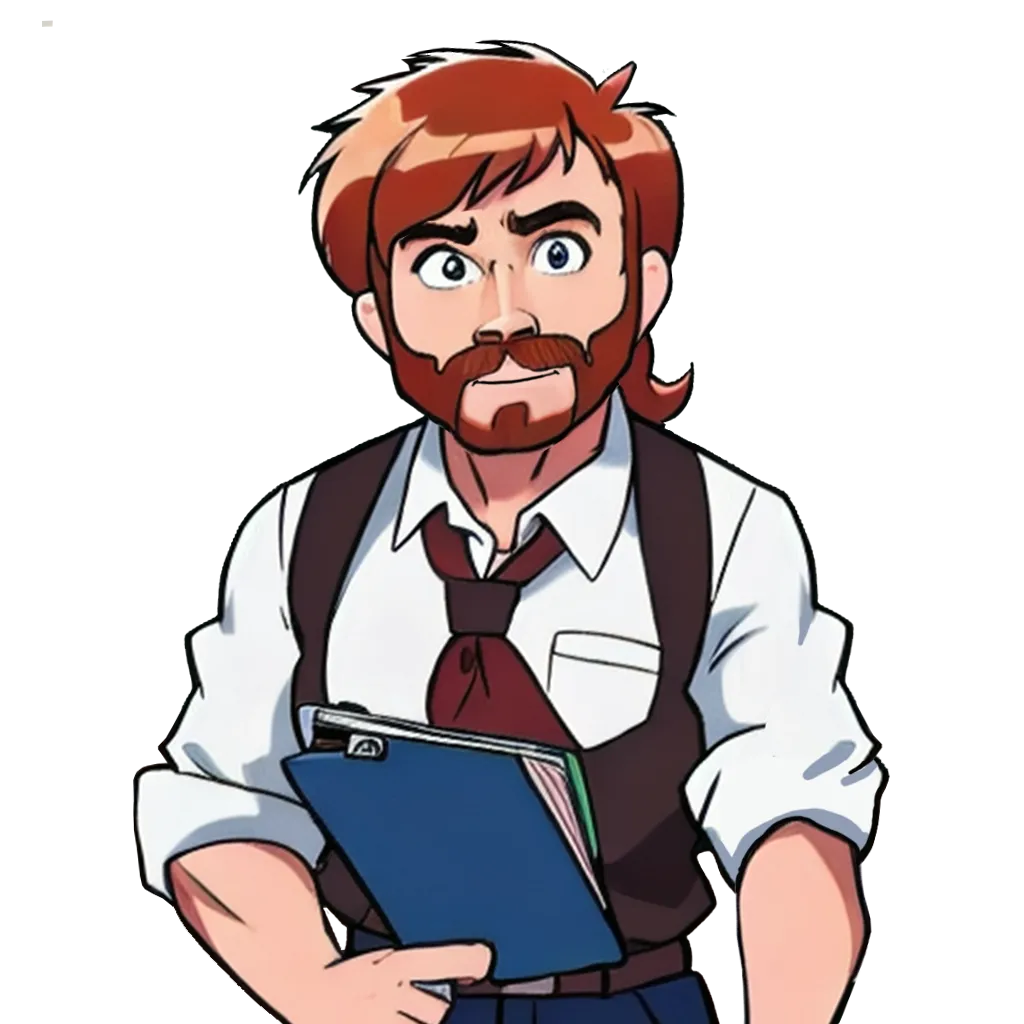
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library