DOM Testing Library
Organization and Structure of Tests in Testing Library
The organization and structure of tests are crucial for maintaining a manageable project and facilitating team collaboration. In this chapter, we will explore best practices for organizing and structuring your tests using DOM Testing Library.
Principles of Test Organization
-
Separation of Concerns:
- Keep your unit tests separate from integration tests and end-to-end tests.
- Organize tests based on the components or functionalities they are testing.
-
Code Reusability:
- Use setup and teardown functions to configure and clean the environment before and after each test.
- Create utilities and helpers for repetitive tasks.
-
Meaningful Names:
- Name your tests descriptively so that their purpose is easy to understand.
- Use file and folder names that clearly reflect the content of the tests.
Common Project Structure
A common project structure might look like this:
Environment Setup and Teardown
Use beforeEach
and afterEach
functions to set up and tear down the test environment.
Example of setup and teardown:
javascript
Using Helpers and Utilities
Creating helpers and utility functions can make your tests more maintainable and avoid code repetition.
Example of a helper to render components:
File test-utils.js
:
javascript
Using the helper in a test:
javascript
Grouping Tests with Describe
You can group related tests using Jest's describe
for better readability and organization.
Example of grouping with describe:
javascript
Dividing Tests by Types
Clear separation between unit tests, integration tests, and end-to-end tests makes management easier and allows focus on different testing levels.
Unit Tests
To test individual components in isolation.
javascript
Integration Tests
To test how multiple components or functions interact.
javascript
Using HOC (Higher Order Components) to Simplify Tests
Higher Order Components (HOC) can be useful for reusing setup and cleanup logic.
Example of a HOC for tests:
javascript
[Placeholder for explanatory image: Diagram illustrating the typical structure of a project with tests organized by types and file hierarchy]
Conclusion
Properly organizing and structuring your tests is essential to maintain a manageable project and facilitate collaboration. Using the techniques and best practices described in this chapter, you can ensure that your tests are clear, consistent, and easy to maintain.
In the next chapter, we will discuss how to integrate your tests into a CI/CD pipeline to automate execution and ensure continuous quality of your application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
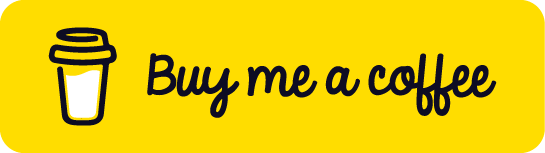
Chat with Chuck
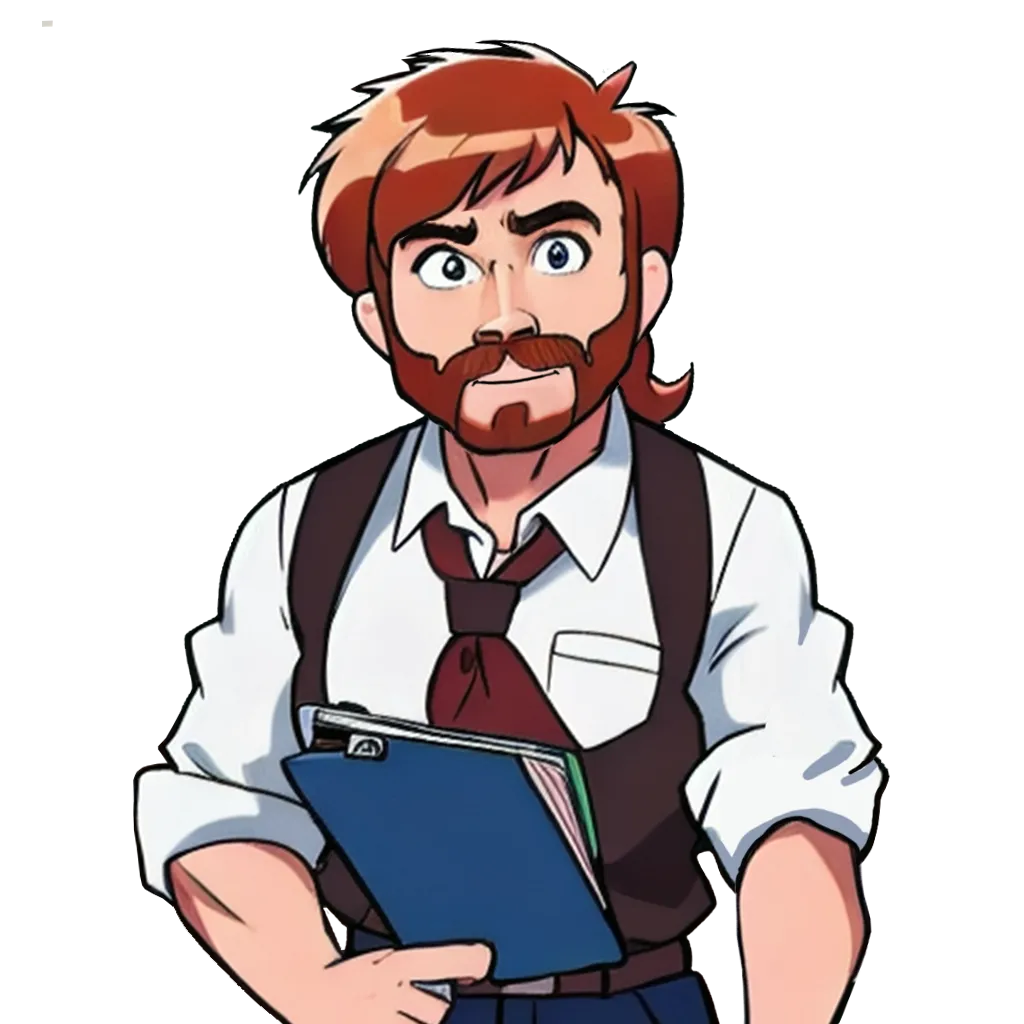
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library