DOM Testing Library
Installation and Setup of Testing Library
To start using DOM Testing Library in your projects, you need to install and set up the appropriate environment. In this topic, we will see how to configure DOM Testing Library step by step, including installation and initial setup.
Prerequisites
Make sure you have Node.js and a package manager like npm or yarn installed. This is necessary to install the dependencies for our tests.
Installing Testing Library
Follow these steps to install DOM Testing Library in your development environment:
-
Initialize your project (if you haven't already):
bashor
bash -
Install DOM Testing Library and its necessary dependencies:
bashor
bash -
Install Jest (or another testing framework). For this course, we will use Jest:
bashor
bash
Configuring Jest
To configure Jest, add the following lines to your package.json
file:
json
Project Structure
Organize your project as follows:
Complete Configuration Example
Let's write a complete example to verify that our configuration works correctly.
- Create a test file in the
tests
folder:
File example.test.js
:
javascript
-
Run your tests:
bashor
bash
If everything is configured correctly, you should see output indicating that the test passed successfully.
[Placeholder for explanatory image: Screenshot of the terminal showing Jest running the test and successful result]
Common Troubleshooting
-
Problem: "Jest encountered an unexpected token": This usually occurs if your code contains ES6 or JSX syntax that Jest doesn't understand. Make sure to have Babel configured if you need support for these features.
-
Problem: "Cannot find module '@testing-library/dom'": Ensure you have installed DOM Testing Library correctly. Check your
package.json
and thenode_modules
folder.
Additional Configuration (Optional)
You can customize Jest's configuration by creating a jest.config.js
file at the root of your project:
javascript
This additional configuration is useful for adding extensions and custom configurations to your testing environment.
With this, you have your testing environment configured and ready to start writing and running tests with DOM Testing Library. You are ready to move on to writing your first unit tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
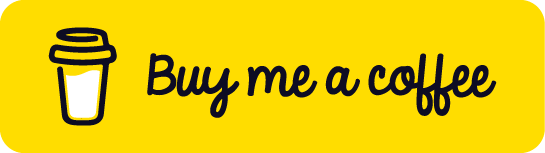
Chat with Chuck
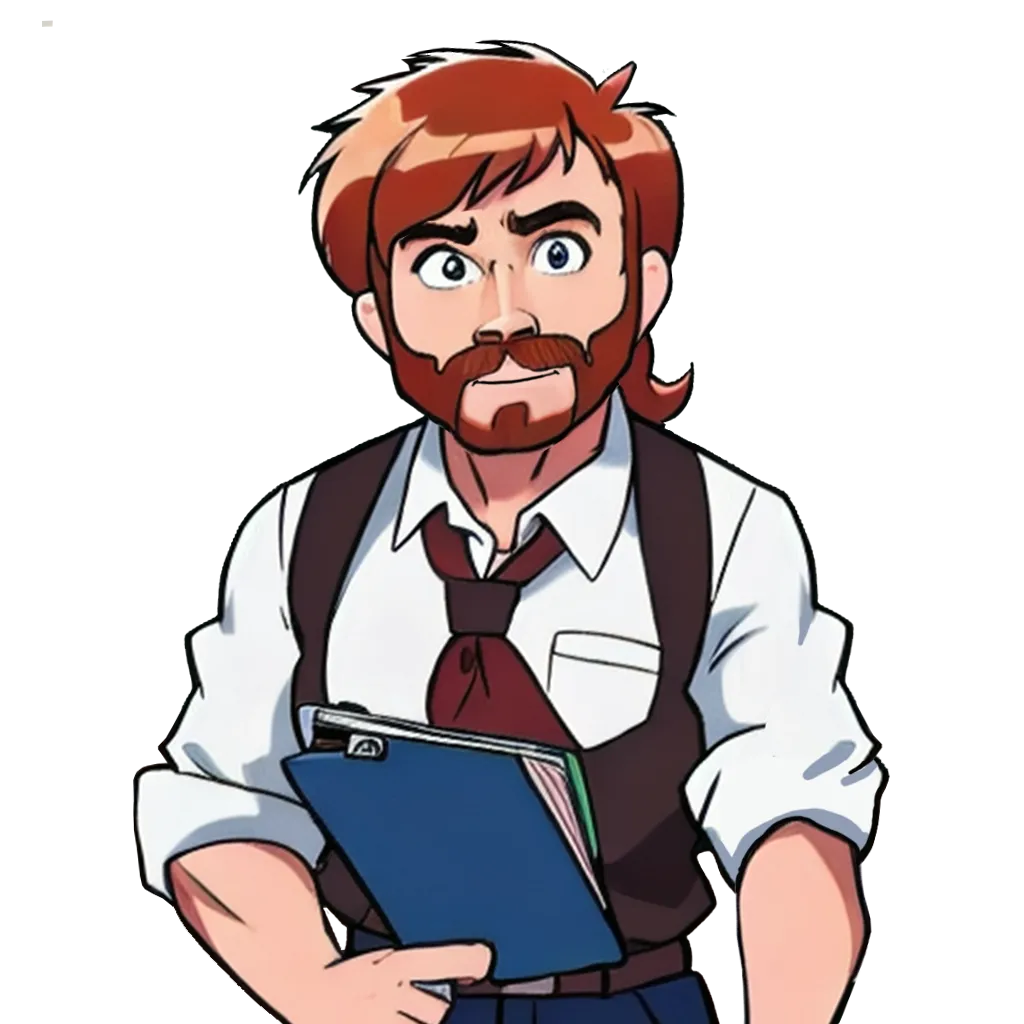
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library